What is the Swift equivalent of isEqualToString in Objective-C?
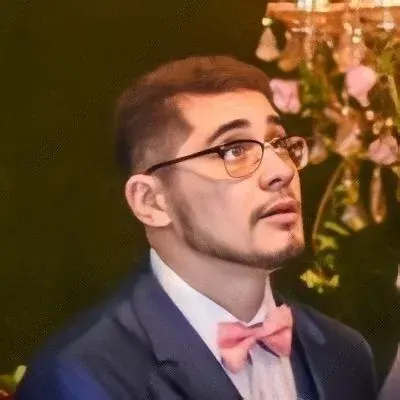
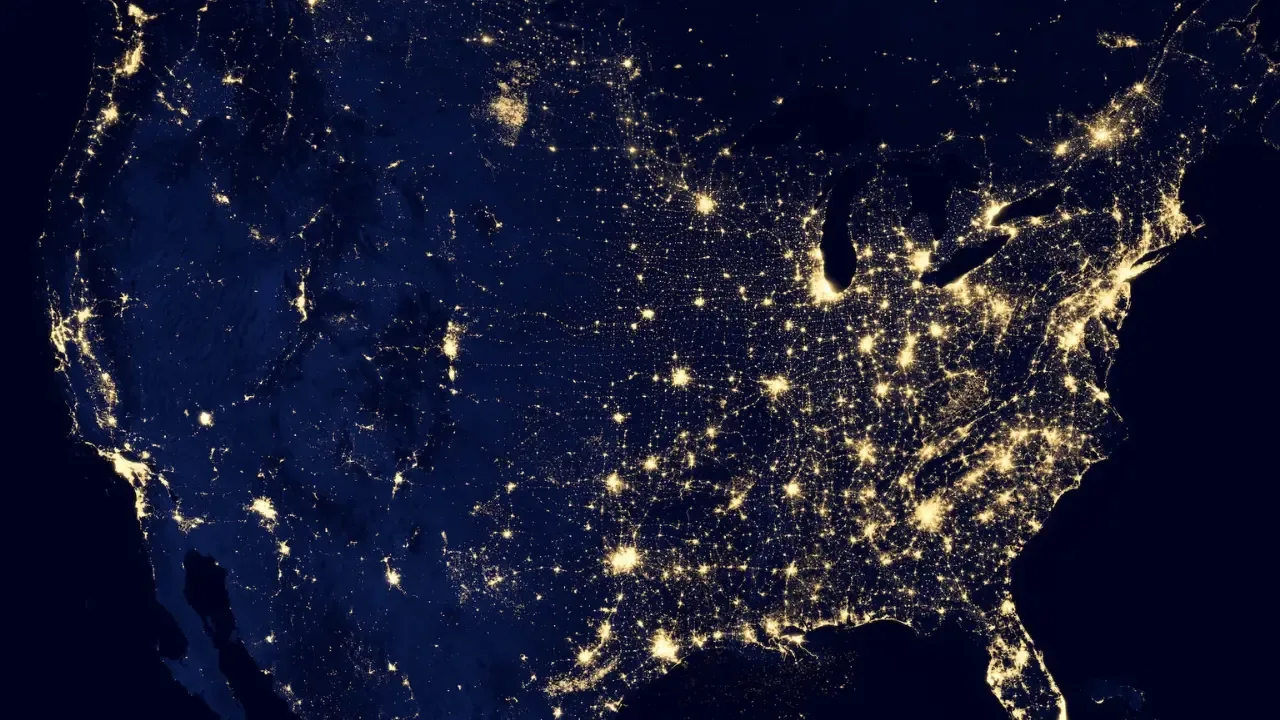
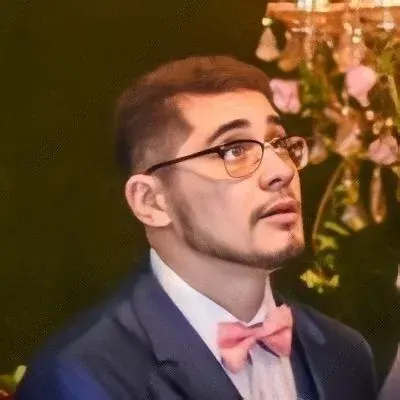
๐ฅ๏ธ๐๐ Hey there! Are you having trouble finding the Swift equivalent of the isEqualToString
method in Objective-C? No worries, I've got you covered! In this blog post, I'll walk you through the common issues surrounding this problem and provide you with an easy solution. So let's dive in! ๐ช๐ป
The code snippet you shared seems to be a part of a LoginViewController
class in Swift. In Objective-C, you were able to check if the username and password text fields were empty using the isEqualToString
method. However, in Swift, the syntax is a bit different.
In Swift, you can use the isEmpty
property of String
to check if a string is empty. So in your loginButton
function, you can modify the if condition as follows:
if username.text?.isEmpty ?? true || password.text?.isEmpty ?? true {
print("Sign in failed. Empty character")
}
Let me explain what's happening here. The text
property of a UITextField in Swift is an optional string, which means it can be either a valid string or nil
. The isEmpty
property is used to check if the optional string is nil
or an empty string.
In the above code, we use the ??
operator (also known as the nil-coalescing operator) to handle the case where text
is nil
. If text
is nil
, we consider it as an empty string and the isEmpty
property evaluates to true
.
By using this approach, you'll achieve the same behavior as the isEqualToString
method in Objective-C. It checks if either the username or password field is empty and logs a message accordingly.
Now that you have the Swift equivalent, you can confidently update your code. Remember to test it thoroughly to ensure it works as expected. ๐งช
If you found this solution helpful or if you have any further questions, feel free to leave a comment below. I'd love to hear from you! ๐๐ปโจ
Happy coding! ๐ป๐