In Bash, how can I check if a string begins with some value?
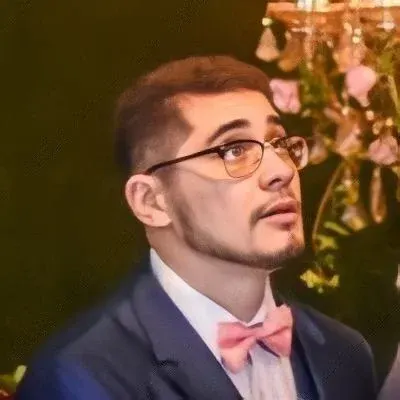
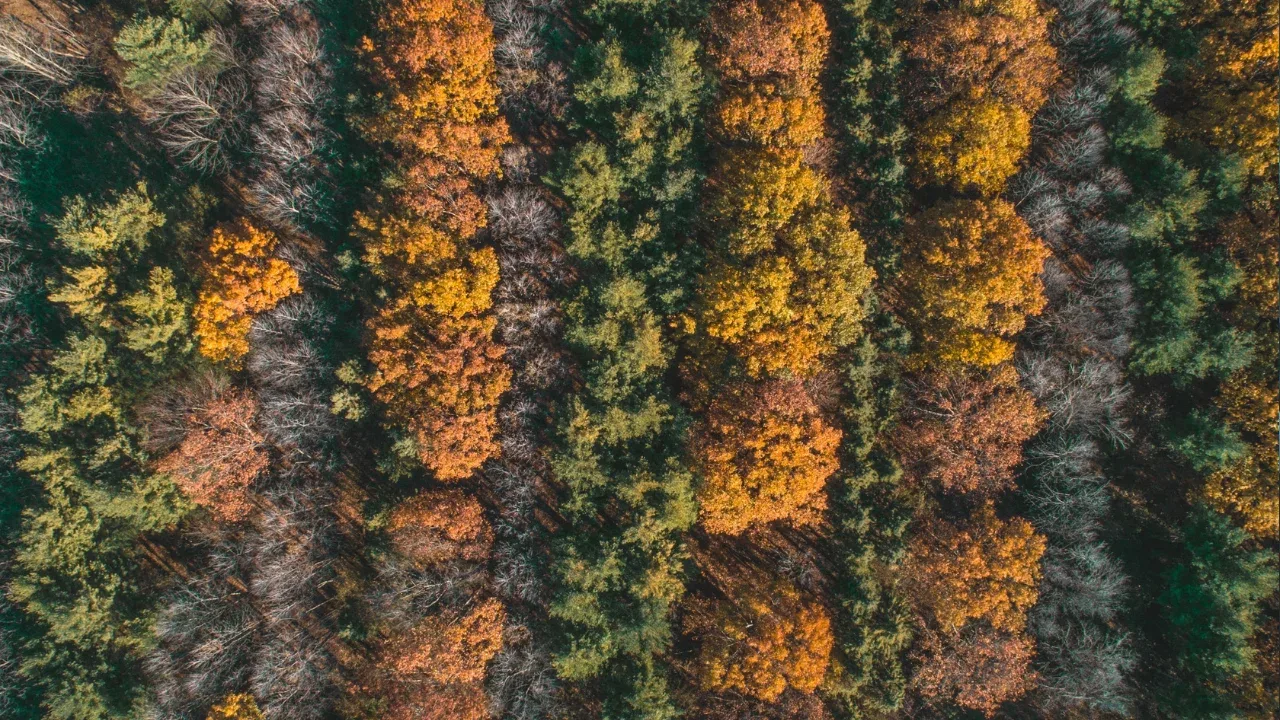
🔍 Checking if a string begins with a value in Bash
Are you having trouble checking if a string starts with a specific value in Bash? Don't worry, we've got you covered! In this blog post, we'll show you easy solutions to address this common issue.
🔸 Problem 1: Checking if a string begins with a specific value
Let's start with the first problem mentioned. The code snippet provided tries to check if the HOST
string starts with "node". However, it's not working as expected:
if [ $HOST == node* ]
then
echo yes
fi
This code isn't correct because the comparison operator ==
does not handle wildcards or pattern matching. To check if a string begins with a specific value, you can use the double square bracket [[ ... ]]
construct with the ==
operator.
Here's the correct way to do it:
if [[ $HOST == node* ]]
then
echo yes
fi
✅ Solution 1:
By using double square brackets [[ ... ]]
and the ==
operator, you can now successfully check if the HOST
string begins with "node". If the condition is met, the script will output "yes".
🔸 Problem 2: Combining expressions to check if a string is "user1" or starts with "node"
You wanted to combine expressions to check if the HOST
is either "user1" or begins with "node". However, the code you tried didn't work:
if [[ $HOST == user1 ]] -o [[ $HOST == node* ]];
then
echo yes
fi
You received the error message -bash: [: too many arguments
. This happens because the logical operator -o
is outside of the if
statement conditions.
🌟 Solution 2:
To correctly combine expressions, modify your code as shown below:
if [[ $HOST == user1 || $HOST == node* ]]
then
echo yes
fi
In Bash, the logical operator ||
is used to represent the logical OR operation. By placing it between the two conditions within the double square brackets [[ ... ]]
, you can check if HOST
is either "user1" or starts with "node". If the condition is true, the script will print "yes".
📢 Call-to-Action: Engage with us!
We hope this blog post helped you understand how to check if a string begins with a specific value in Bash. If you found it useful or have any questions, feel free to share your thoughts in the comments section below. We'd love to hear from you!
✨ Now it's your turn! Try out the examples provided and let us know your experience. Share your own tips or solutions to help the community. Together, we can master Bash scripting! ✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
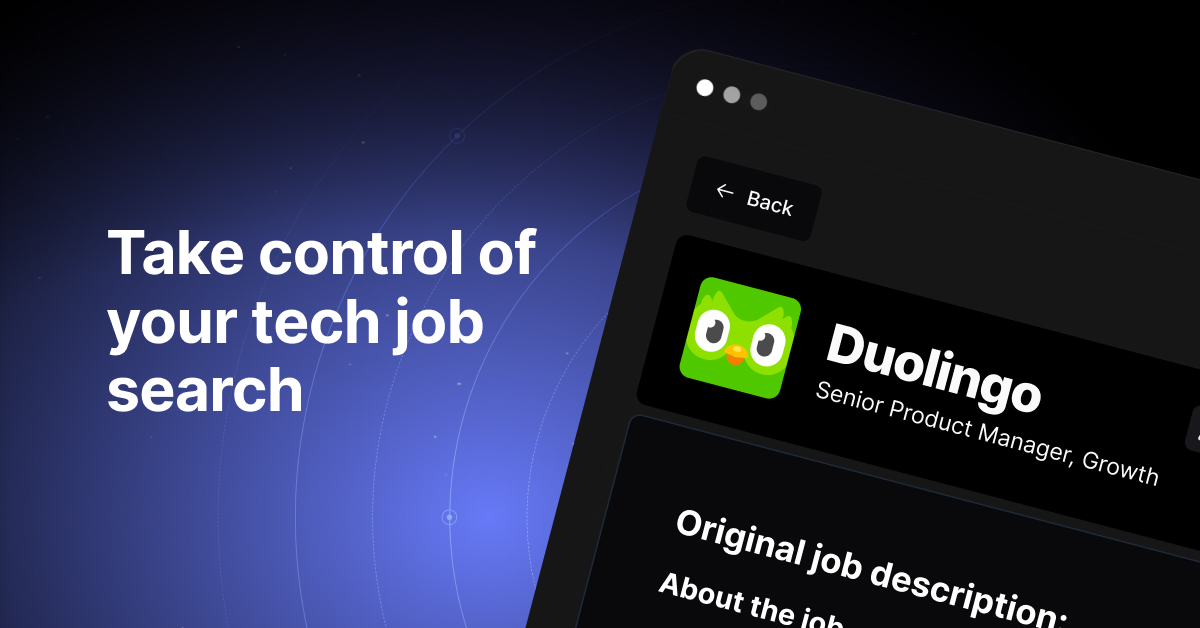