How to efficiently concatenate strings in go
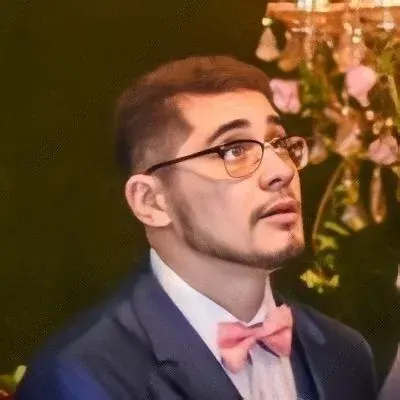
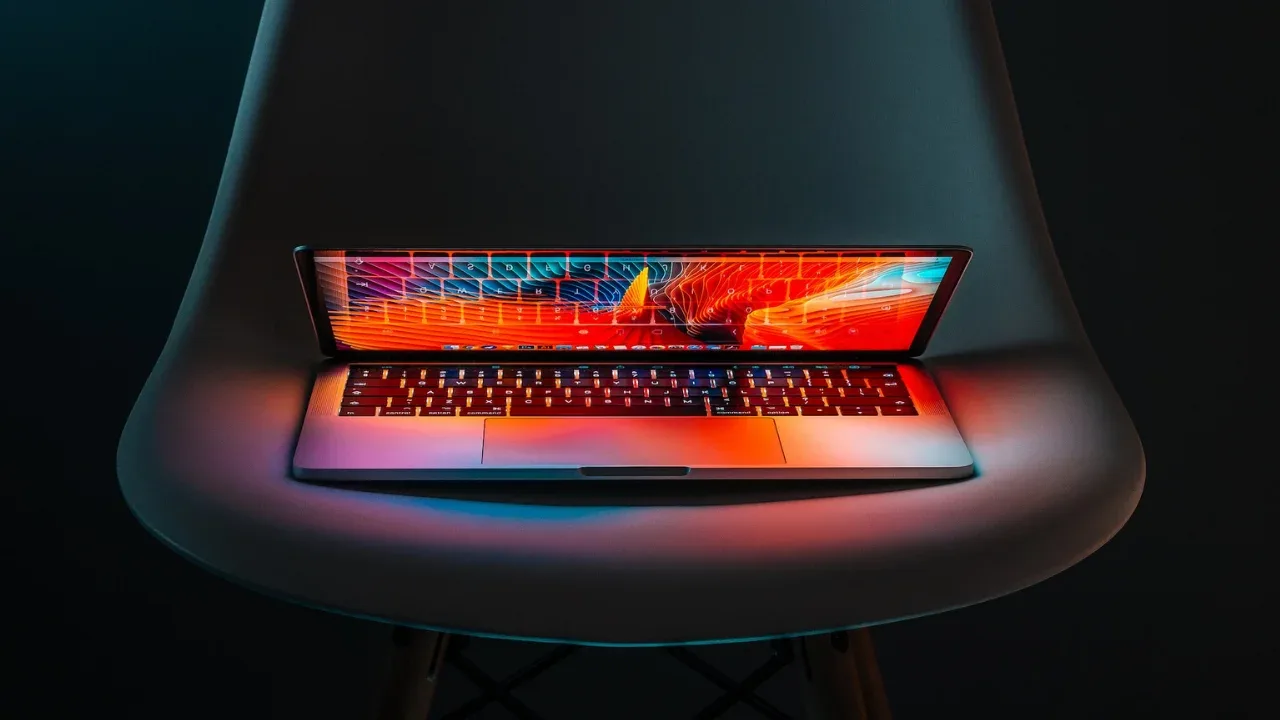
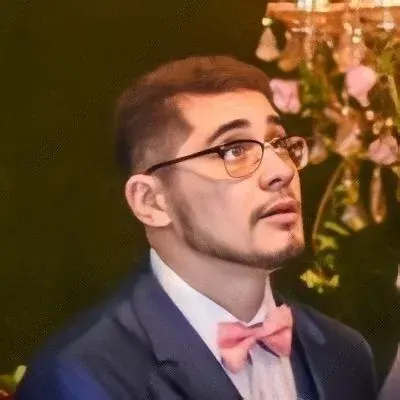
Efficient String Concatenation in Go: 🚀
Are you struggling with concatenating strings efficiently in Go? You've come to the right place! In this blog post, we'll explore the common issue of inefficient string concatenation and provide you with easy solutions to make your code 💨 faster and more optimized. So let's dive in!
Understanding the Problem: 🤔
In Go, strings are read-only and attempting to manipulate them directly will result in the creation of a new string object each time. This can lead to performance issues, especially when you need to concatenate strings multiple times without knowing the final length.
The Naive Approach: 🤦♀️
Before we reveal the efficient solutions, let's address the less optimal approach commonly used:
var s string
for i := 0; i < 1000; i++ {
s += getShortStringFromSomewhere()
}
return s
While it may seem straightforward, this method creates a new string for each concatenation. Consequently, it can lead to excessive memory allocations and slower execution times.
Solution 1: Using the strings.Builder
Type: 👷♂️
Go provides the strings.Builder
type, which is specifically designed for efficient string concatenation. It minimizes memory allocations by reserving extra space and reducing the number of string copies.
Here's an example on how to use strings.Builder
:
var sb strings.Builder
for i := 0; i < 1000; i++ {
sb.WriteString(getShortStringFromSomewhere())
}
return sb.String()
By utilizing the WriteString
method, we can efficiently append strings together. Finally, the String()
method retrieves the resulting concatenated string.
Solution 2: Utilizing strings.Join()
: ⛓️
Another approach to achieve efficient string concatenation is by using strings.Join()
. This method takes a slice of strings and concatenates them efficiently using a separator.
Here's an example of strings.Join()
in action:
slice := make([]string, 1000)
for i := range slice {
slice[i] = getShortStringFromSomewhere()
}
return strings.Join(slice, "")
By pre-allocating a slice and populating it with the desired strings, we can leverage the optimized concatenation abilities of strings.Join()
.
Time to Optimize Your Code! 💪
Now that you have two efficient solutions for concatenating strings in Go, it's time to optimize your code and improve its performance. Choose the method that suits your requirements best and start enjoying faster execution times!
Remember, when efficiency meets simplicity, your code becomes a powerhouse! 👩💻
Did you find this blog post helpful? Share your thoughts, experiences, or alternative approaches in the comments below. Let's optimize our Go code together! 🚀