How to convert an int value to string in Go?
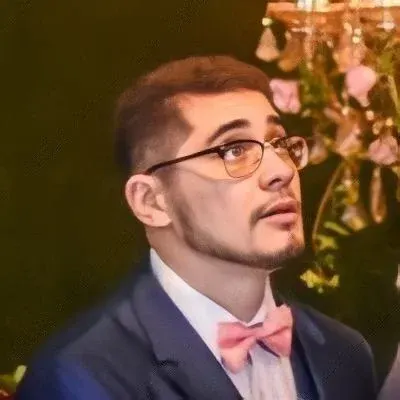

Converting an int value to string in Go: The Ultimate Guide
👋 Hey there, fellow Gophers! Are you struggling to convert an int value to a string in your Go code? Fret not, because I've got you covered! In this comprehensive guide, we'll explore common issues, easy solutions, and some bonus tips to help you tackle this problem.
The Problem: "E" instead of "123"
Let's start by taking a look at a common issue that Gophers face when converting an int value to string in Go. Consider the following code snippet:
i := 123
s := string(i)
At first glance, you might expect the value of s
to be "123". However, to your surprise, s
is actually 'E'. 🙀
The Solution: strconv.Itoa()
The reason for this unexpected behavior is that the string()
function in Go expects a Unicode code point as an argument, not an integer value. To convert an int value to string in Go, we can leverage the strconv
package's Itoa()
function.
import "strconv"
i := 123
s := strconv.Itoa(i)
Voilà! 🎉 By using strconv.Itoa()
, the value of s
will be "123", just as you intended. The Itoa()
function stands for "integer to ASCII," and it's specifically designed to convert integer values to their corresponding string representation.
Bonus: Concatenating Strings in Go
Now that we've solved the int-to-string conversion, let's tackle another question brought up in the initial context: how to concatenate two strings in Go. In Java, you might be used to using the "+" operator for string concatenation, as demonstrated in this example:
String s = "ab" + "c"; // s is "abc"
In Go, the process is slightly different. Instead of using the "+" operator, we can use the +=
operator to concatenate strings. Here's how it looks:
s := "ab" + "c" // s is "abc"
Easy peasy, right? 😎
Conclusion
There you have it! Converting an int value to string in Go can be achieved effortlessly using strconv.Itoa()
. Plus, by utilizing the +=
operator, string concatenation becomes a breeze in Go. Now that you're armed with this knowledge, go forth and conquer your coding challenges!
🙌 If you found this guide helpful, don't forget to share it with your fellow Gophers so they can benefit too. Feel free to leave your thoughts, questions, or any other Go-related topics you'd like me to cover in the comments section below. Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
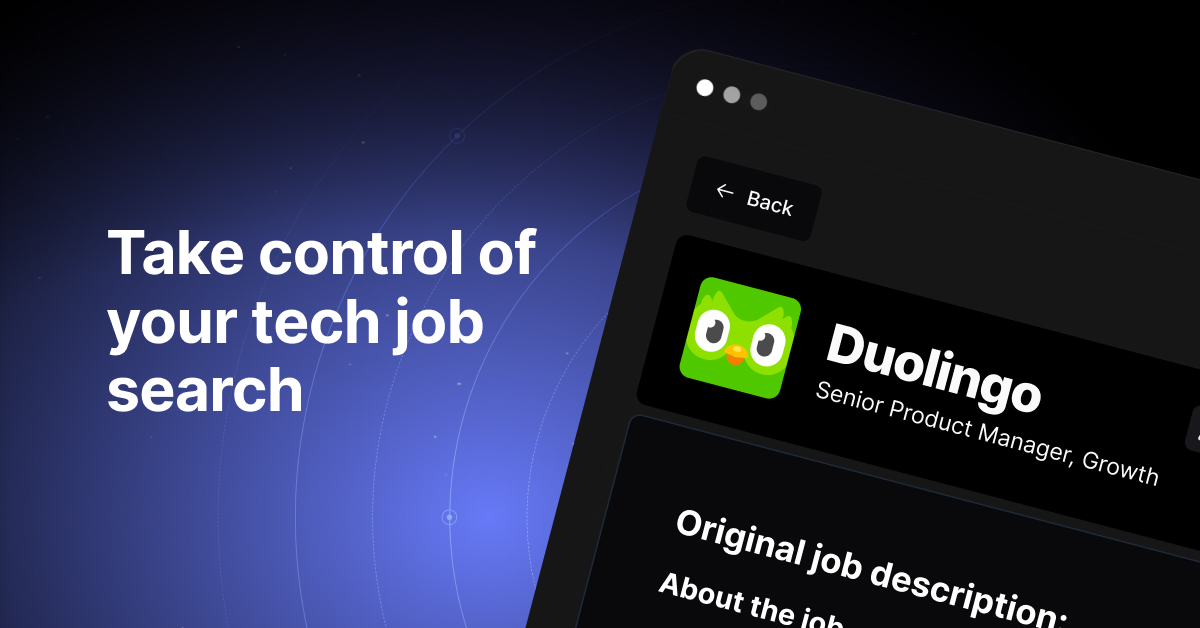