How to check if a string contains a substring in Bash
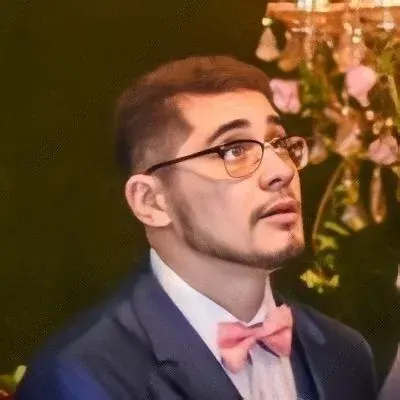
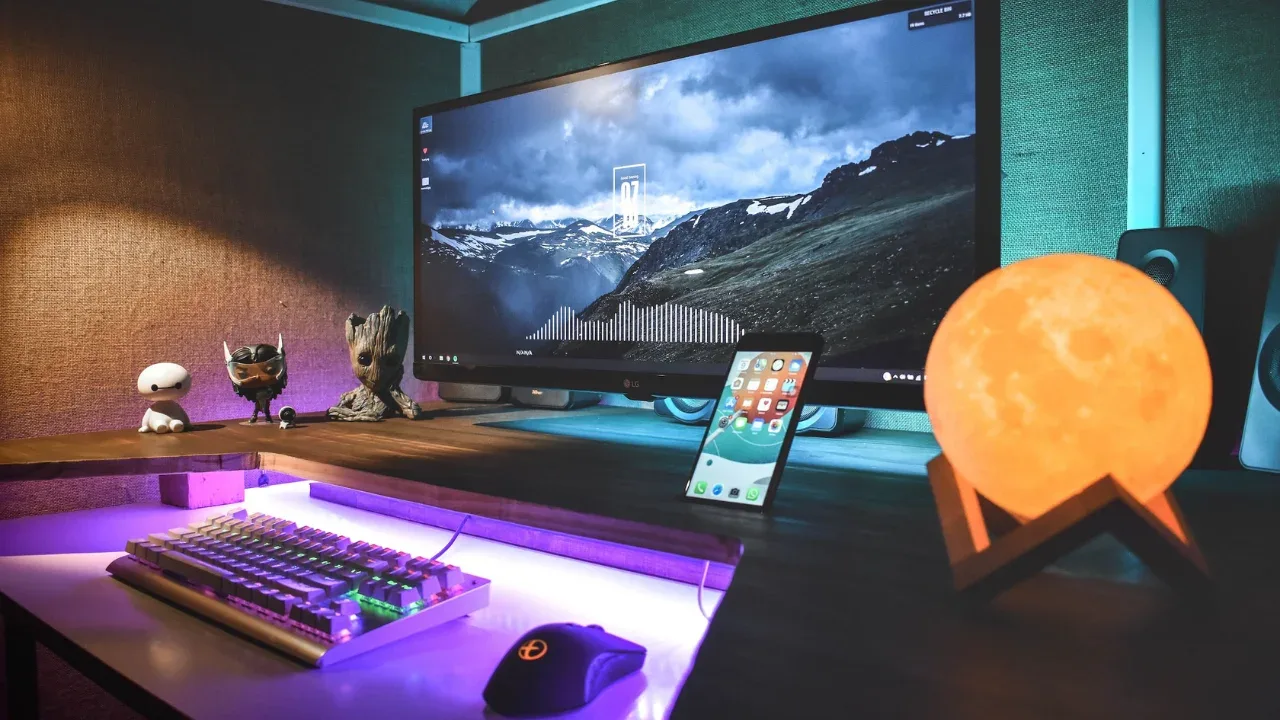
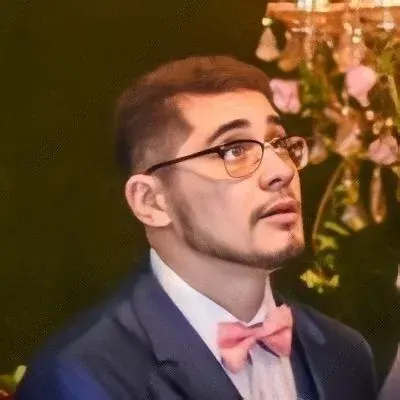
š Title: How to Check if a String Contains a Substring in Bash: Simplified Solutions and Examples! š»š
š Introduction: Have you ever wondered how to easily check if a string contains a specific substring in Bash? š¤ Look no further, as we're about to dive into some simple yet powerful solutions to solve this common problem. Whether you're a Bash novice or a pro, this guide will provide you with easy-to-understand examples and explanations. Let's get started! š
š” The Problem: Imagine you have a string in Bash, say "My string", and you need to check if it contains another string, let's say "foo". How would you tackle this task? š¤·āāļø
š ļø Solution 1: Using Wildcards:
One way to check for a substring in Bash is by using wildcards. We can employ the double brackets [[]]
and the ==
operator to achieve this. Here's an example:
string="My string"
if [[ $string == *foo* ]]; then
echo "It's there!"
fi
šļø This simple code uses the *
wildcard before and after the substring we want to find. If the substring "foo" exists within the string, the condition will be true, and "It's there!" will be displayed. š
š ļø Solution 2: Using the case
Statement:
Another Bash solution is to utilize the case
statement. This statement is often used for pattern matching, making it suitable for our needs. Check out this example:
string="My string"
case "$string" in
*foo*) echo "It's there!" ;;
esac
šļø In this case, we surround the substring we're looking for with *
wildcards, within the parentheses. If the substring "foo" is found within the string, the echo statement will be executed. Clean and simple! šŖ
š£ Call-to-Action: Now that you know how to check if a string contains a substring in Bash, it's time to put this knowledge into action! Experiment with different strings and substrings, and try out these solutions in your own projects. Don't forget to share your experiences and newfound skills in the comments section below! Let's engage and learn from each other. š¤
ā
Conclusion:
Checking for substrings in Bash doesn't have to be a daunting task anymore. With the wildcard and case
statement solutions provided, you have two simple yet effective ways to tackle this problem. Remember, simplicity is key when it comes to writing clean and efficient code. So go ahead, give these solutions a try, and level up your Bash scripting game! šŖ
(Word count: 386)