Generate random alphanumeric string in Swift
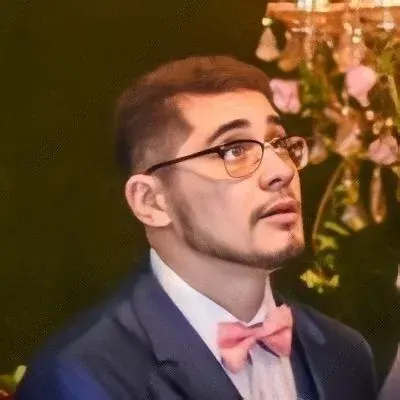
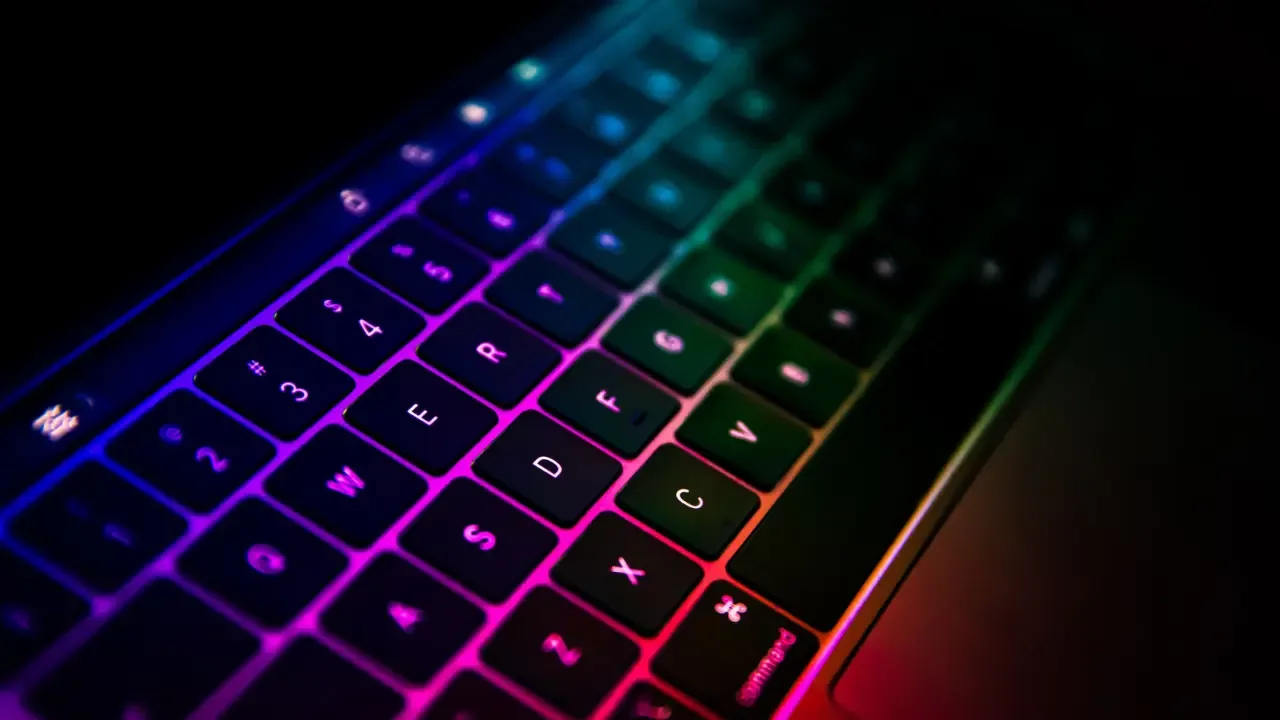
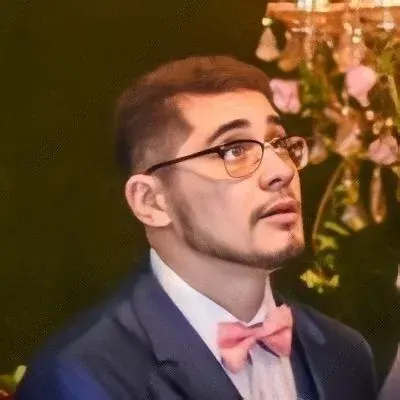
π² How to Generate Random Alphanumeric Strings in Swift
π¬ Introduction:
Are you looking for a way to generate a random alphanumeric string in Swift? Look no further! In this blog post, we'll explore common issues around generating random strings and provide easy solutions to help you tackle this problem with ease. So let's dive right in!
β The Problem:
The question of generating a random alphanumeric string in Swift is a common one among developers. Whether you're building an app, a game, or need a unique identifier, random strings come in handy. But how do you generate such strings programmatically?
π‘ The Solution:
1. Using arc4random_uniform
:
One way to generate a random alphanumeric string is by utilizing the arc4random_uniform
function in Swift. This function generates a random number within a specified range. Here's an example:
func generateRandomString(length: Int) -> String {
let letters = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
var randomString = ""
for _ in 0..<length {
let randomIndex = Int(arc4random_uniform(UInt32(letters.count)))
let character = letters[letters.index(letters.startIndex, offsetBy: randomIndex)]
randomString += String(character)
}
return randomString
}
let randomString = generateRandomString(length: 10)
print(randomString)
In this example, we define a function generateRandomString
that takes a desired length
as a parameter. It then creates a string letters
containing all possible characters for our alphanumeric string. Next, we iterate length
times, generating a random index from 0
to letters.count-1
using arc4random_uniform
. We then retrieve the character at the random index and append it to our randomString
. Finally, we return the generated random string.
2. Using UUID
:
Another approach is to use the built-in UUID
type in Swift to generate a random alphanumeric string. UUID
generates a unique identifier that consists of alphanumeric characters separated by dashes. However, if you want a string without the dashes, you can remove them using replacingOccurrences
. Here's an example:
let randomString = UUID().uuidString.replacingOccurrences(of: "-", with: "")
print(randomString)
In this example, we directly utilize the UUID
type to generate a unique identifier as a string. We then use replacingOccurrences(of:with:)
to remove the dashes from the generated string.
πͺ Try it yourself:
Now that you have two easy ways to generate random alphanumeric strings in Swift, feel free to experiment with them in your own projects. Need a 20-character string? Simply call the generateRandomString(length:)
function with length: 20
! Have fun coding!
βοΈ Share your thoughts:
What other methods or techniques have you used to generate random alphanumeric strings in Swift? We'd love to hear your thoughts and experiences. Leave a comment below and let's start a discussion!
π Conclusion:
Generating random alphanumeric strings in Swift doesnβt need to be a complicated task. By utilizing the arc4random_uniform
function or the UUID
type, you can easily generate unique strings of your desired length. So go ahead, give it a try, and level up your app or game with some random awesomeness!
Note: Remember that while these methods generate random strings, they do not guarantee perfect randomness. If you require cryptographically secure random strings, consider using a library specifically designed for that purpose.