Check if a string contains another string
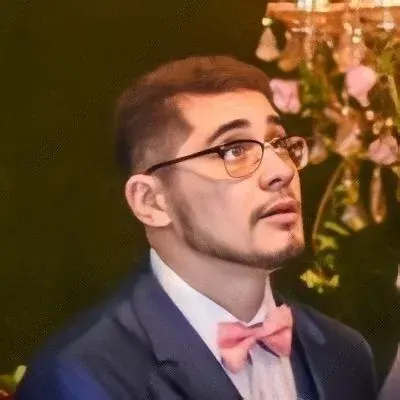
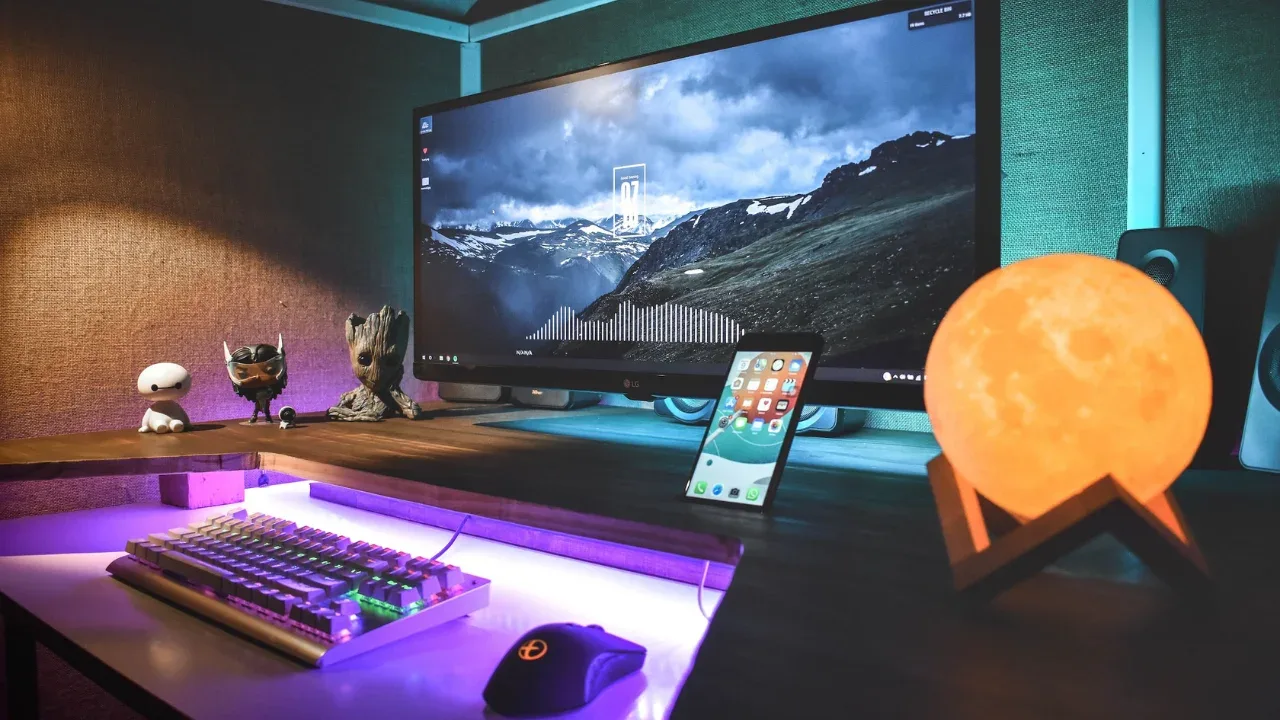
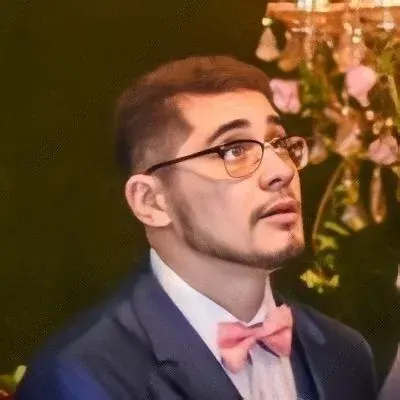
💡 How to Check if a String Contains Another String: The Comma Dilemma
Are you tired of reading a string character by character in search of a specific substring? 🤔 In this blog post, we will explore different options to address the question: "How can I check if a string contains a comma without resorting to reading char-by-char?" 😯
👀 The Problem: Spotting the Comma
The challenge at hand is to determine whether a given string contains a comma. 📝 Our user was specifically looking for an alternative to tedious character-by-character reading.
💡 Easy Solution #1: Using the includes()
Method
JavaScript comes to the rescue with an easy solution! ✨ The includes()
method checks if a string contains a specified substring, returning either true
or false
. Here's how you could use it to check for a comma:
const stringToCheck = "Hello, World!";
const containsComma = stringToCheck.includes(",");
console.log(containsComma); // Output: true
By using includes()
, we avoid reading the string character by character. Isn't that a relief? 🎉
💡 Easy Solution #2: Using Regular Expressions
Regular expressions offer a powerful toolset for pattern matching and finding strings within strings. We can use a regular expression to check for the presence of a comma. Here's how:
const stringToCheck = "Hello, World!";
const regex = /,/;
const containsComma = regex.test(stringToCheck);
console.log(containsComma); // Output: true
In this example, we create a regular expression /,/
that matches any occurrence of a comma. Then, we use the test()
method to check if our string contains the comma. Pretty nifty, huh? 😎
🌟 Your Turn to Shine
Now that you have learned two easy solutions to check if a string contains a comma, it's your turn to try them out! 🔍 Use these methods in your own projects and see how they make your code more efficient and readable.
📣 Share Your Experience
Have you used any of these techniques before? ✍️ What other creative methods have you used? Share your thoughts, experiences, and ideas in the comments section below. Let's learn from each other! 👥💬
Remember, the world of programming is vast, and there's always something new to discover. Stay curious! 😊