How can I get the current stack trace in Java?
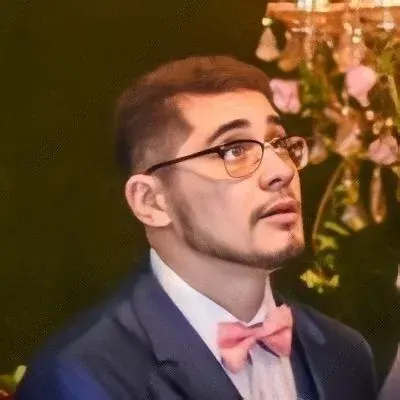
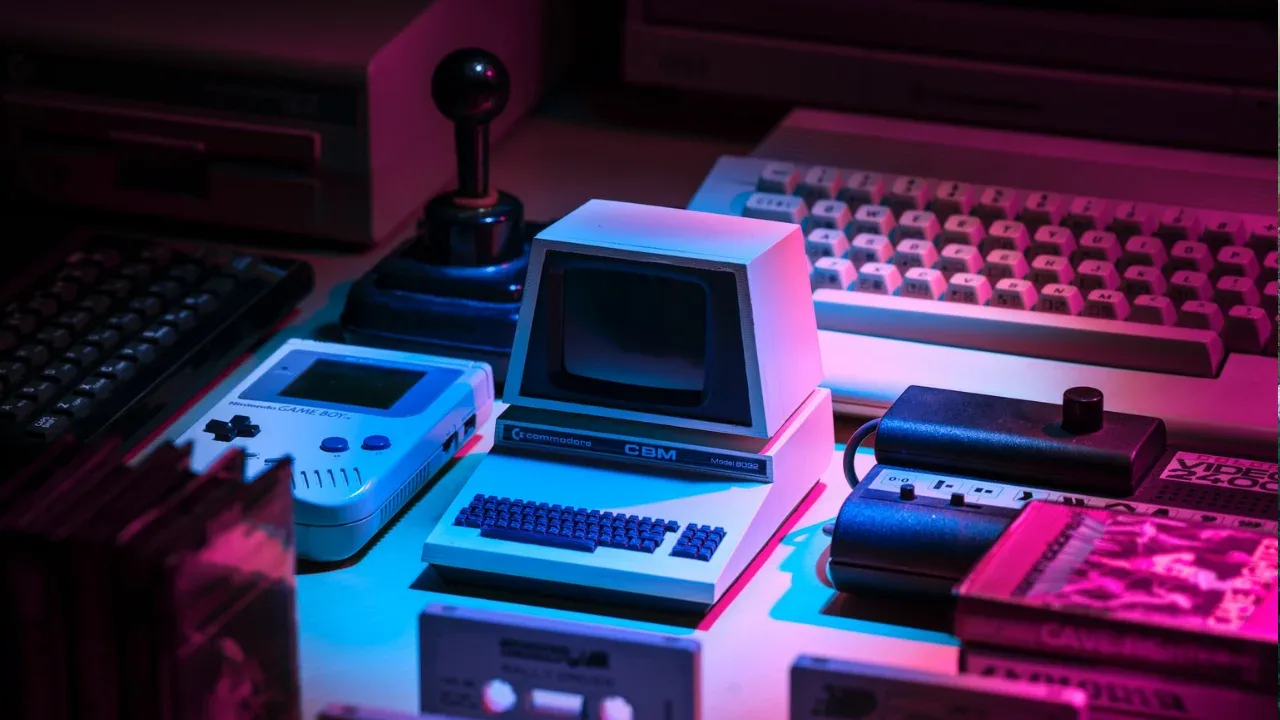
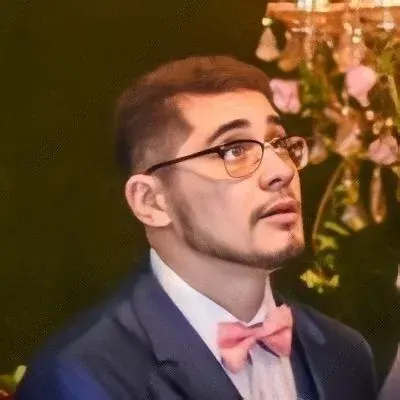
How to Get the Current Stack Trace in Java: A Comprehensive Guide 🕵️♂️
Do you ever find yourself in a situation where you need to get the current stack trace in Java? Maybe you're debugging a complex codebase or trying to understand the flow of execution in your application. Well, you're in luck! In this blog post, we will explore common issues and provide easy solutions to help you get the stack trace in Java. Let's dive in! 🚀
Understanding the Stack Trace 📚
First, let's understand what a stack trace is. In simple terms, a stack trace is a record of the active stack frames at a particular point during the execution of a program. It tells you which methods were called in what order, leading up to the current point of execution. Each stack frame represents a method call, and the stack trace shows the sequence of these method calls.
The Problem: Thread.dumpStack()
Isn't Enough 😕
You might stumble upon the Thread.dumpStack()
method, which prints the stack trace to the standard error stream. However, this method doesn't provide the stack trace as a data structure that you can manipulate programmatically. But worry not, we have a solution for you!
Solution 1: Using Thread.currentThread().getStackTrace()
🎯
To obtain the current stack trace in Java, you can utilize the getStackTrace()
method provided by the Thread
class. Here's an example of how you can use it:
StackTraceElement[] stackTrace = Thread.currentThread().getStackTrace();
for (StackTraceElement element : stackTrace) {
System.out.println(element);
}
By accessing the current thread using Thread.currentThread()
, you can then call the getStackTrace()
method to retrieve an array of StackTraceElement
objects. Each element in this array represents a stack frame, containing useful information such as the class name, method name, and line number.
Solution 2: Leveraging Throwable.getStackTrace()
🎯
Another way to obtain the stack trace is by using Throwable
class methods. Take a look at the following example:
Throwable throwable = new Throwable();
StackTraceElement[] stackTrace = throwable.getStackTrace();
for (StackTraceElement element : stackTrace) {
System.out.println(element);
}
By creating a new instance of Throwable
, you can then call the getStackTrace()
method to retrieve the current stack trace. This solution is useful when you don't have access to the current thread, such as in a static context.
Solution 3: Using Thread.getAllStackTraces()
for All Threads 🌐
Sometimes, you may need to obtain the stack traces of all currently running threads in your Java application. In such cases, you can utilize the getAllStackTraces()
method from the Thread
class. Here's an example:
Map<Thread, StackTraceElement[]> threadMap = Thread.getAllStackTraces();
for (Map.Entry<Thread, StackTraceElement[]> entry : threadMap.entrySet()) {
Thread thread = entry.getKey();
StackTraceElement[] stackTrace = entry.getValue();
System.out.println("Thread: " + thread.getName());
for (StackTraceElement element : stackTrace) {
System.out.println(element);
}
}
This solution returns a Map
where the keys represent the threads and the values are the corresponding stack traces. You can iterate over this map to access the stack traces of all the threads in your application.
Engage with Us! 📢
We hope this guide helps you retrieve the current stack trace in Java effortlessly. Now it's your turn! Share your thoughts in the comments section below:
Have you encountered any challenges when retrieving stack traces in Java?
Do you know any alternative approaches?
What other Java topics would you like us to cover in future blog posts?
Let's keep the conversation going! Don't hesitate to drop a comment and let us know your thoughts. Happy coding! 😄👩💻👨💻