Update multiple rows in same query using PostgreSQL
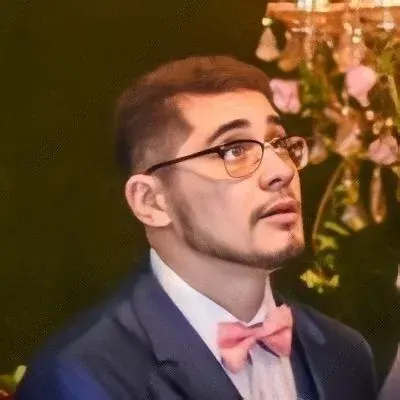
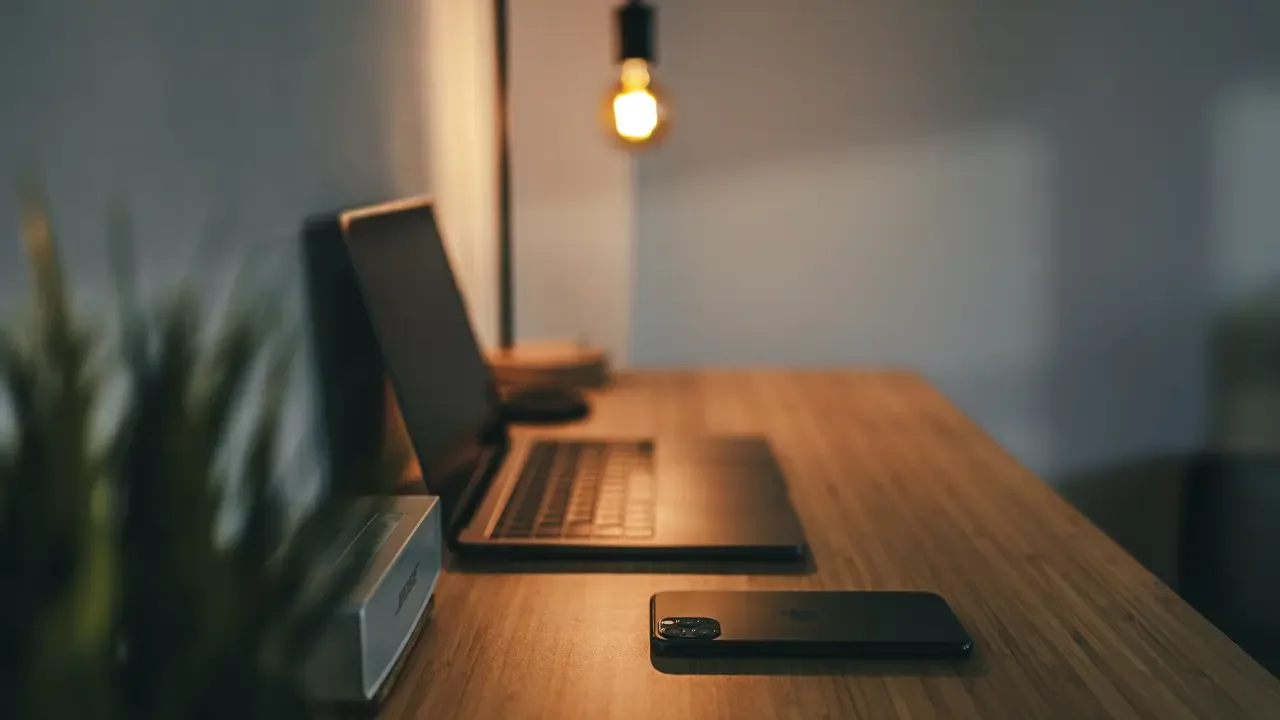
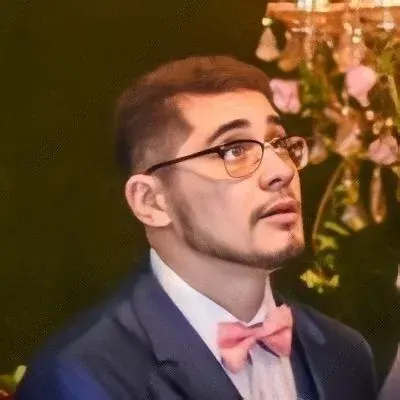
Updating Multiple Rows in One Go Using PostgreSQL 💪
Updating multiple rows in PostgreSQL can be a bit tricky, but fear not! We've got you covered with easy solutions to this common problem. In this blog post, we'll explore how you can update multiple rows in one statement using PostgreSQL, saving you time and effort. 🚀
The Challenge ⚡️
Let's start by understanding the challenge at hand. You want to update multiple rows in a single query, but the syntax you provided in your question is not quite right. Unfortunately, PostgreSQL doesn't support updating multiple rows using a single set of SET
statements like that.
The Solution ✔️
Don't worry! PostgreSQL provides us with a powerful feature called the CASE
expression, which we can leverage to update multiple rows at once. Here's how you can achieve this:
UPDATE table
SET
column_a = CASE
WHEN column_b = '123' THEN 1
WHEN column_b = '345' THEN 2
ELSE column_a
END
WHERE
column_b IN ('123', '345');
Let's break down this solution:
We use the
CASE
expression within theSET
statement to conditionally update thecolumn_a
value.Each
WHEN
clause checks the value ofcolumn_b
and setscolumn_a
accordingly.The
ELSE
clause ensures thatcolumn_a
remains unchanged if none of the specified conditions are met.The
WHERE
clause specifies which rows to update by filtering on the values ofcolumn_b
.
You can customize the conditions and values according to your specific requirements. 🛠️
Test Drive 🚗
To better understand how this works, let's assume we have the following table called "employees":
| id | name | salary |
|----|--------|--------|
| 1 | Alice | 3000 |
| 2 | Bob | 2500 |
| 3 | Charlie| 2000 |
We want to update the salaries of Alice and Charlie. Here's how our query would look like:
UPDATE employees
SET
salary = CASE
WHEN name = 'Alice' THEN 3500
WHEN name = 'Charlie' THEN 2200
ELSE salary
END
WHERE
name IN ('Alice', 'Charlie');
After executing this query, our updated table would look like this:
| id | name | salary |
|----|--------|--------|
| 1 | Alice | 3500 |
| 2 | Bob | 2500 |
| 3 | Charlie| 2200 |
Hooray! We successfully updated multiple rows using a single query. 👏
Take It to the Next Level 👩💻
Now that you've learned how to update multiple rows in one go using PostgreSQL, the possibilities are endless. You can apply this technique to a wide range of scenarios, from simple updates to more complex conditional modifications. 🌟
So go ahead and unleash the power of PostgreSQL with multiple row updates! If you have any questions or face any issues, feel free to leave a comment below. Happy coding! 😄💻
[INSERT ENGAGING CALL-TO-ACTION HERE]
Liked this post? Share it with your fellow developers and spread the PostgreSQL goodness! 🙌📢
➡️ Do you have more questions about PostgreSQL or any other tech topics? Follow our blog for more informative and engaging content! And don't forget to connect with us on social media for daily updates and tech discussions. Let's grow together! 🌱💪
*[CASE]: A syntax structure in SQL that allows conditional evaluations and execution of code blocks. *[SET]: A keyword used in SQL to assign new values to database columns. *[WHERE]: A clause used to filter specific rows in a SQL statement.