SQL Update from One Table to Another Based on a ID Match
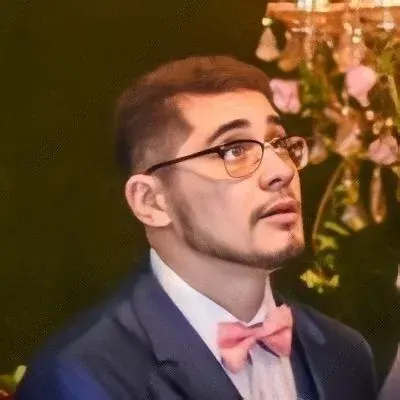
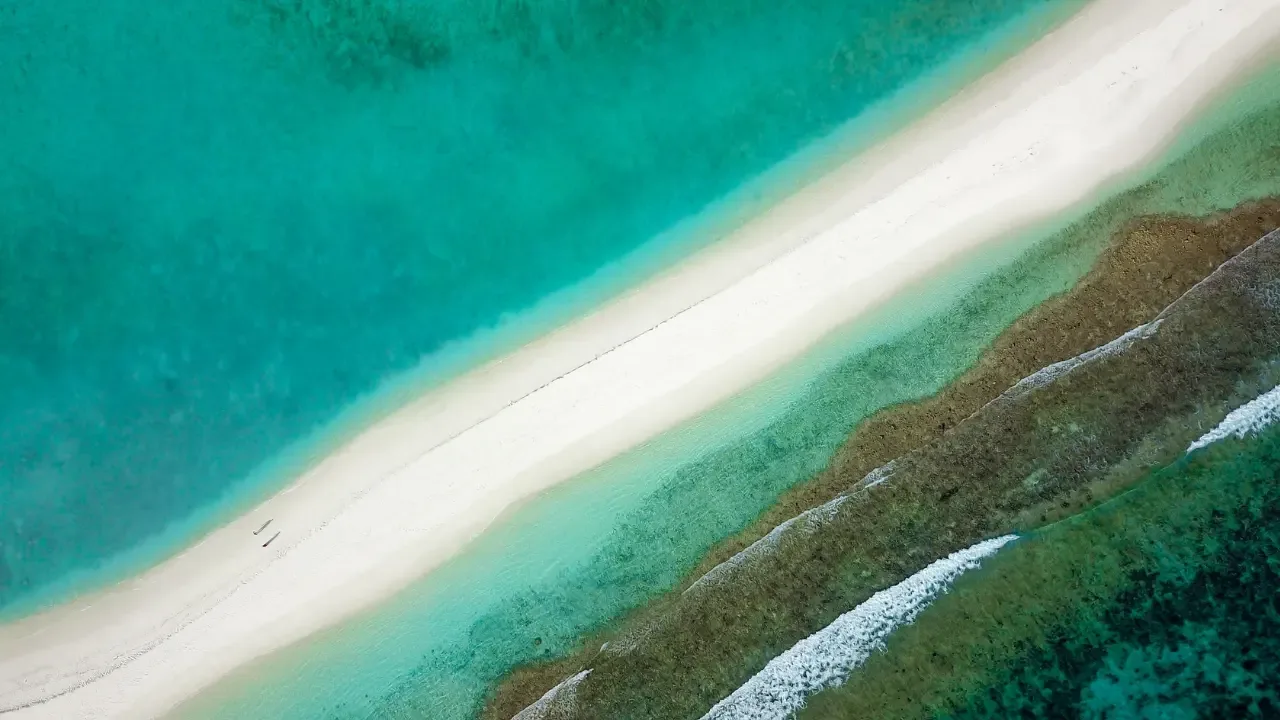
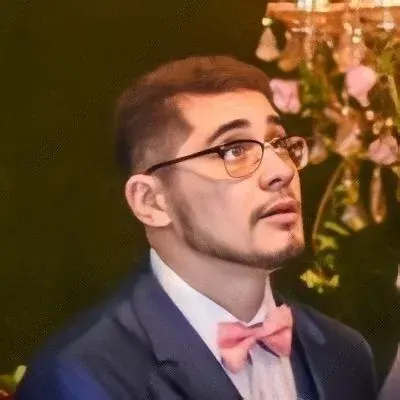
Updating Account Numbers from One Table to Another Based on ID Match
Updating data in one table based on the values in another table is a common task in SQL. In this blog post, we will explore a specific problem where we need to update account numbers in the Sales_Import
table based on a matching ID in the RetrieveAccountNumber
table. We will address the issue of the account numbers getting replaced by NULL
and provide an easy solution to fix it.
Understanding the Problem
The problem statement provides two tables: Sales_Import
and RetrieveAccountNumber
. We need to update the AccountNumber
field in the Sales_Import
table with the corresponding account number from the RetrieveAccountNumber
table, based on a matching LeadID
.
The Initial Attempt
The code snippet provided in the problem statement is a good start, but it is missing a crucial piece. Let's take a closer look at it:
UPDATE [Sales_Lead].[dbo].[Sales_Import]
SET [AccountNumber] = (SELECT RetrieveAccountNumber.AccountNumber
FROM RetrieveAccountNumber
WHERE [Sales_Lead].[dbo].[Sales_Import]. LeadID =
RetrieveAccountNumber.LeadID)
This UPDATE
query attempts to update the AccountNumber
column in the Sales_Import
table by selecting the corresponding account number from the RetrieveAccountNumber
table. The join condition is based on the LeadID
column.
Fixing the Issue
To address the problem of the account numbers getting replaced by NULL
, we need to update our query slightly. Let's take a look at the modified query:
UPDATE [Sales_Lead].[dbo].[Sales_Import]
SET [AccountNumber] = (SELECT RetrieveAccountNumber.AccountNumber
FROM RetrieveAccountNumber
WHERE [Sales_Lead].[dbo].[Sales_Import]. LeadID =
RetrieveAccountNumber.LeadID)
WHERE EXISTS (SELECT 1
FROM RetrieveAccountNumber
WHERE [Sales_Lead].[dbo].[Sales_Import]. LeadID =
RetrieveAccountNumber.LeadID)
The key addition in this updated query is the WHERE EXISTS
clause. This clause ensures that only the rows with a matching LeadID
in the RetrieveAccountNumber
table are updated in the Sales_Import
table. It filters out the rows where there is no corresponding account number available.
Wrapping Up
Updating data from one table to another based on a matching ID can be tricky, especially when dealing with null values and missing matches. By adding the WHERE EXISTS
clause to our query, we can ensure that only valid updates are performed, preventing the account numbers from being replaced by NULL
.
If you found this blog post helpful or have any questions, feel free to leave a comment below. Happy coding! 😄🎉