SQL join: selecting the last records in a one-to-many relationship
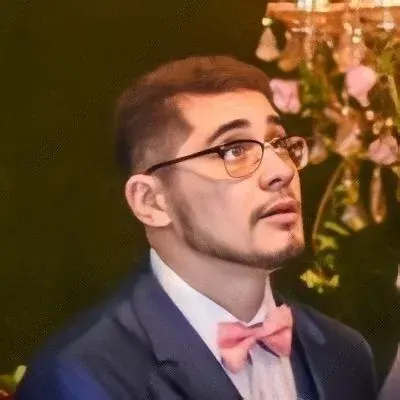
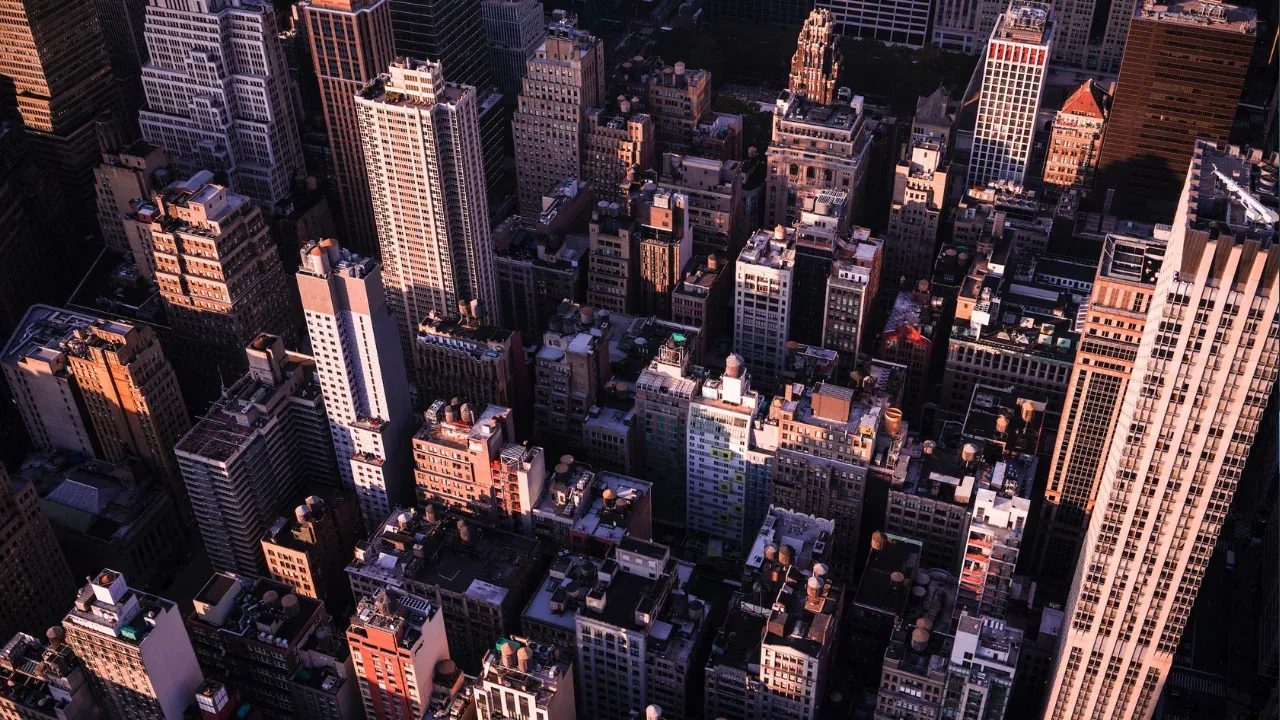
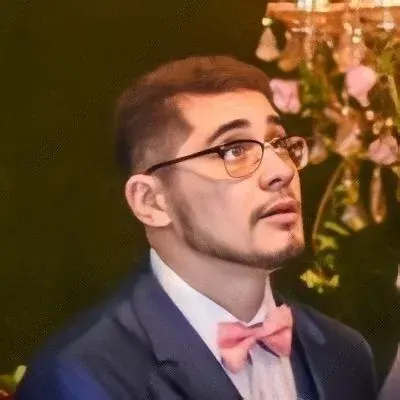
📝 SQL JOIN: Selecting the Last Records in a One-to-Many Relationship
Are you struggling with selecting the last records in a one-to-many relationship using SQL JOIN? Look no further! In this blog post, I'll provide easy solutions to this common issue and even give you advice on building indexes. Let's dive in! 💻
First, let's grasp the context. Suppose you have two tables: "customer" and "purchase". Each purchase is associated with one customer. So, the challenge is to obtain a list of all customers along with their last purchase in a single SELECT statement. 📋
Here are the table/column names we'll be working with:
customer table: id, name
purchase table: id, customer_id, item_id, date
Now, let's address the main question: What is the best practice?
To achieve our goal, we can utilize the power of SQL JOIN and some clever techniques. One way to approach this is by leveraging a subquery. We can use the subquery to fetch the maximum date for each customer and then join it with the purchase table to retrieve the corresponding record.
Here's an example query that accomplishes this:
SELECT c.id, c.name, p.item_id, p.date
FROM customer c
JOIN purchase p ON c.id = p.customer_id
JOIN (
SELECT customer_id, MAX(date) AS max_date
FROM purchase
GROUP BY customer_id
) last_purchase ON p.customer_id = last_purchase.customer_id AND p.date = last_purchase.max_date;
In this query, we first perform a subquery where we select the customer_id and the maximum date for each customer from the purchase table. Then, we join the customer and purchase tables, matching the customer_id and date to obtain the desired result.
Now, let's address the second part of the question: Any advice on building indexes?
When dealing with large datasets, indexes can significantly improve the performance of your queries. In this case, consider creating an index on the "date" column in the purchase table. This index will speed up the sorting process required to find the maximum date for each customer.
💡 Pro tip: If you frequently need to fetch the last purchase for a specific customer, you can create another index on the "customer_id" and "date" columns in the purchase table. This index will further optimize the retrieval of last purchases for individual customers.
And finally, a thought-provoking question arises: Should you denormalize the database by putting the last purchase into the customer table?
Denormalization, as the name suggests, involves storing redundant data in order to improve query performance. While denormalizing by adding the last purchase to the customer table may speed up certain queries, it also introduces data redundancy and the need for careful management to ensure data integrity.
Consider denormalization only if the performance gains outweigh the potential downsides and if it aligns with your overall database design and usage patterns.
🔍 As a bonus, let's address one last question: If the purchase id is guaranteed to be sorted by date, can the statements be simplified using something like LIMIT 1?
Unfortunately, using LIMIT 1 alone won't give you the desired result. It will only return the last purchase for the first customer. Remember, we need to fetch the last purchase for each customer, not just one.
To summarize, fetching the last records in a one-to-many relationship using SQL JOIN can be achieved by using subqueries and clever join conditions. Don't forget to consider building appropriate indexes for better query performance, and carefully analyze the trade-offs of denormalizing your database.
Now it's your turn! Have you encountered any challenges with SQL JOIN or selecting last records in a one-to-many relationship? Share your thoughts and experiences in the comments below. Let's learn from each other! 💬
Happy coding! ✨🚀