Solutions for INSERT OR UPDATE on SQL Server
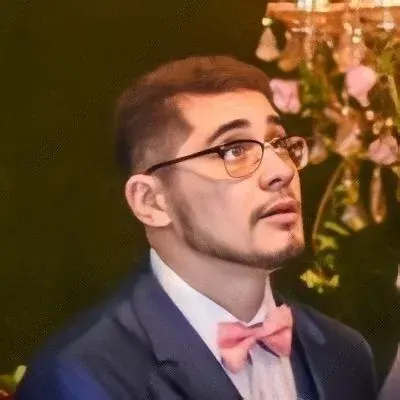
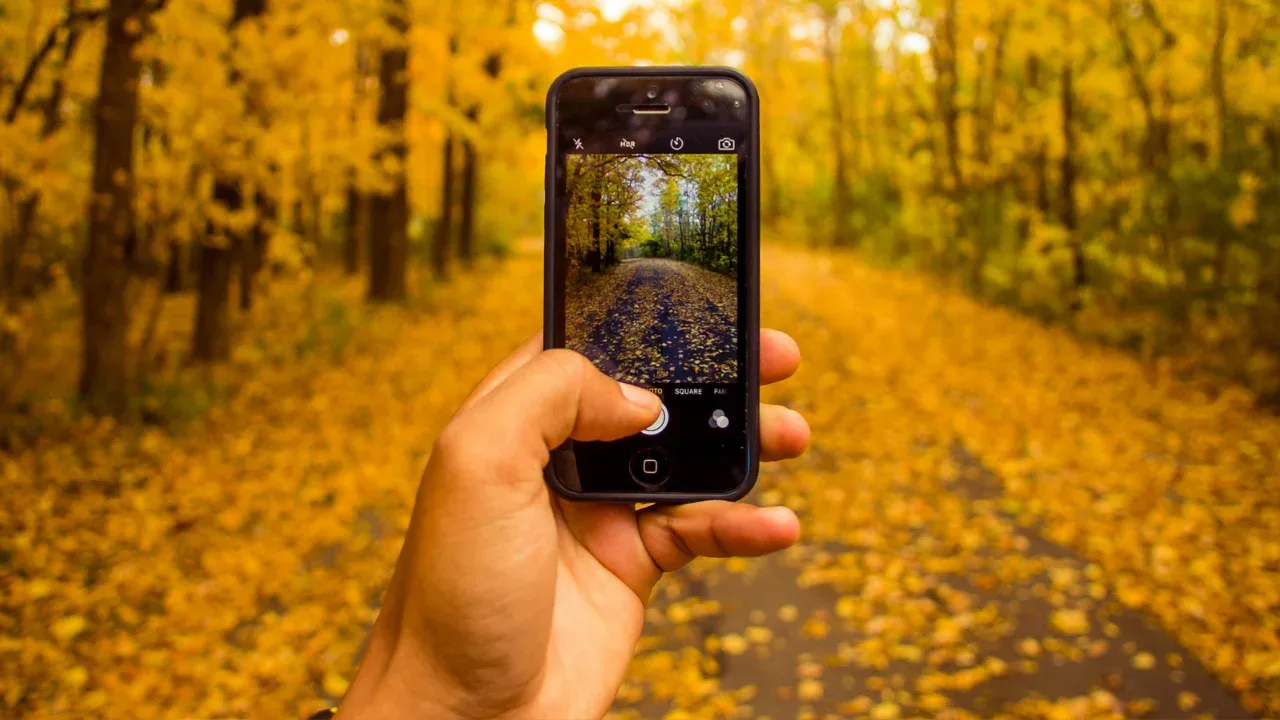
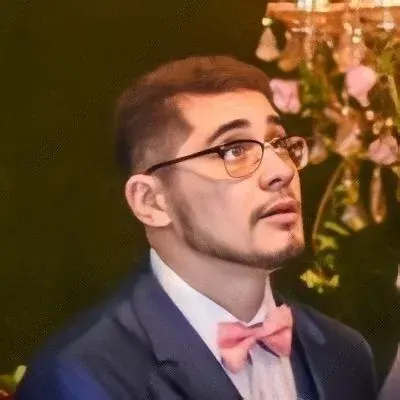
The Best Performing Way to INSERT OR UPDATE on SQL Server
š Welcome to my tech blog! In this post, we'll tackle the age-old question of how to efficiently handle the common scenario of inserting or updating records in a SQL Server database. š»š¾
The Problem:
š¤ Imagine you have a table named MyTable
with several data fields, including the primary key KEY
. You often find yourself needing to either update an existing record or insert a new record if it doesn't exist. You want to achieve this in the most optimal way, in terms of performance and simplicity.
The Solution:
š Fortunately, SQL Server provides us with a powerful feature called MERGE
that allows us to handle this situation efficiently.
Here's an example query that achieves the desired functionality:
MERGE MyTable AS target
USING (VALUES (@key, @datafield1, @datafield2, ... )) AS source (KEY, datafield1, datafield2, ...)
ON target.KEY = source.KEY
WHEN MATCHED THEN
UPDATE SET datafield1 = source.datafield1, datafield2 = source.datafield2, ...
WHEN NOT MATCHED THEN
INSERT (KEY, datafield1, datafield2, ...)
VALUES (source.KEY, source.datafield1, source.datafield2, ...);
This query uses the MERGE
statement to combine the update and insert operations into one atomic operation. It first attempts to match the KEY
column in the source with the target table. If a match is found, the data fields are updated. If not, a new record is inserted into the table.
Benefits of the MERGE Statement:
ā
Performance: The MERGE
statement optimizes the operation by minimizing round trips to the database and reducing network overhead. It can handle large datasets efficiently, making it the best choice for bulk insert/update scenarios.
ā
Simplicity: By using a single query, you eliminate the need for multiple conditional statements or separate update and insert queries. This simplifies your codebase and improves readability.
Action Time!
š£ Now that you know the best way to handle INSERT OR UPDATE scenarios in SQL Server, give it a try! Adapt the provided example to your specific table structure and requirements. Feel free to reach out in the comments section if you have any questions or need further assistance.
Let's optimize our SQL statements together! šŖ
ā Have you faced similar data manipulation scenarios before? How did you tackle them? Share your experiences, tips, and tricks in the comments below. Let's learn from each other! š
Happy coding! šš©āš»šØāš»