Simulate CREATE DATABASE IF NOT EXISTS for PostgreSQL?
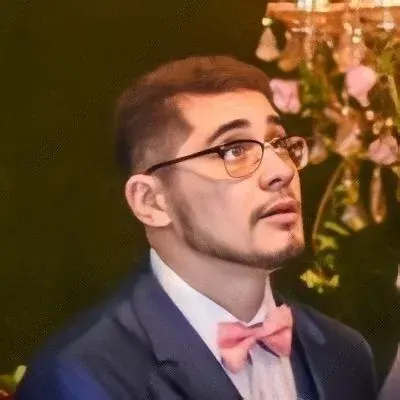
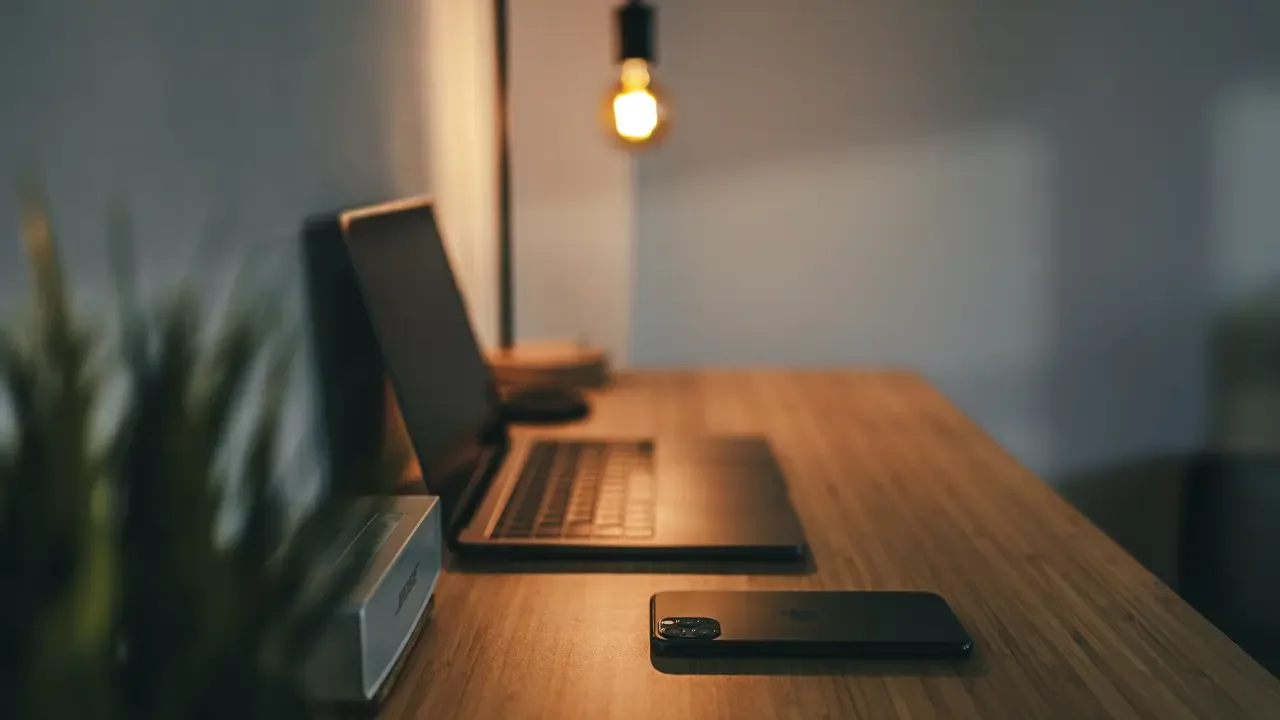
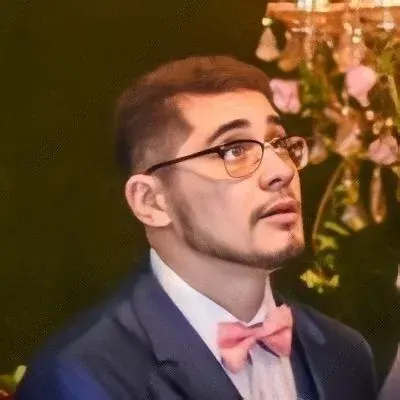
Simulate CREATE DATABASE IF NOT EXISTS for PostgreSQL?
Are you struggling to simulate the CREATE DATABASE IF NOT EXISTS
functionality in PostgreSQL? Don't worry, you're not alone! It's a common issue for developers who are used to working with MySQL, which does support this syntax. In this blog post, we'll explore the problem at hand, provide easy solutions, and encourage reader engagement with a compelling call-to-action. Let's dive in! 💻🔥
Understanding the Problem
The problem arises when you want to create a database through JDBC in PostgreSQL, but you're unsure if the database already exists. Unlike MySQL, PostgreSQL doesn't natively support the create if not exists
syntax. So, how can we accomplish this task effectively? 🤔
Solution 1: Connecting to the Desired Database
One approach is to connect to the desired database using JDBC, and if the connection fails, assume that the database does not exist. In that case, you can create a new database by connecting to the default postgres
database. Here's an example:
String dbName = "your_database_name";
String url = "jdbc:postgresql://localhost/postgres";
String username = "your_username";
String password = "your_password";
try {
Connection conn = DriverManager.getConnection(url, username, password);
Statement stmt = conn.createStatement();
String createDB = "CREATE DATABASE " + dbName;
stmt.executeUpdate(createDB);
System.out.println("Database created successfully!");
} catch (SQLException e) {
System.err.println("Failed to create database: " + e.getMessage());
}
In this code snippet, we attempt to establish a connection with the desired database. If the connection fails, an exception will be thrown, indicating that the database doesn't exist. In the catch block, we can then proceed to create the database using the default postgres
database connection. Easy, isn't it? 😄
Solution 2: Checking Database Existence
Another method to achieve the desired functionality is to connect to the postgres
database and check if the desired database already exists. If it does exist, you can use it; otherwise, create a new database. This approach requires a bit more work, but it can be equally effective. Here's an example to help you visualize:
String dbName = "your_database_name";
String url = "jdbc:postgresql://localhost/postgres";
String username = "your_username";
String password = "your_password";
try {
Connection conn = DriverManager.getConnection(url, username, password);
Statement stmt = conn.createStatement();
String checkDB = "SELECT 1 FROM pg_database WHERE datname = '" + dbName + "'";
ResultSet result = stmt.executeQuery(checkDB);
if (result.next()) {
System.out.println("Database already exists! Using existing database.");
// Use the existing database
} else {
String createDB = "CREATE DATABASE " + dbName;
stmt.executeUpdate(createDB);
System.out.println("New database created successfully!");
}
} catch (SQLException e) {
System.err.println("Failed to create or use database: " + e.getMessage());
}
In this approach, we query the pg_database
table in the postgres
database to check if the desired database already exists. If the query returns any rows, it means the database exists, and we can go ahead and use it. Otherwise, we can create a new database. 💡
Your Turn to Take Action!
Now that you've learned two effective ways to simulate the CREATE DATABASE IF NOT EXISTS
functionality in PostgreSQL, it's time to put your knowledge into practice. Try implementing one of the solutions in your code and see the magic happen! ✨
We hope this guide has been helpful in solving the problem you were facing. If you have any questions or need further clarification, feel free to leave a comment below or reach out to our support team. Happy coding! 😄🚀
Don't forget to share this blog post with your fellow developers who might be struggling with the same issue. Sharing is caring! Spread the knowledge! 🙌
[JDBC]: Java Database Connectivity [URL]: Unified Resource Locator [SQL]: Structured Query Language