SQL Server add auto increment primary key to existing table
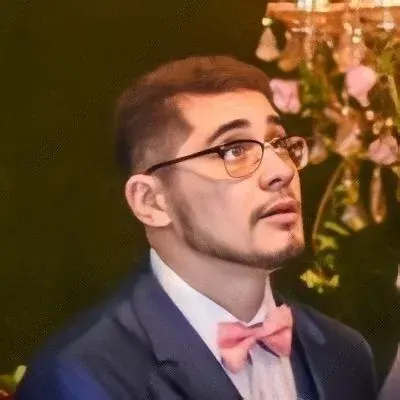
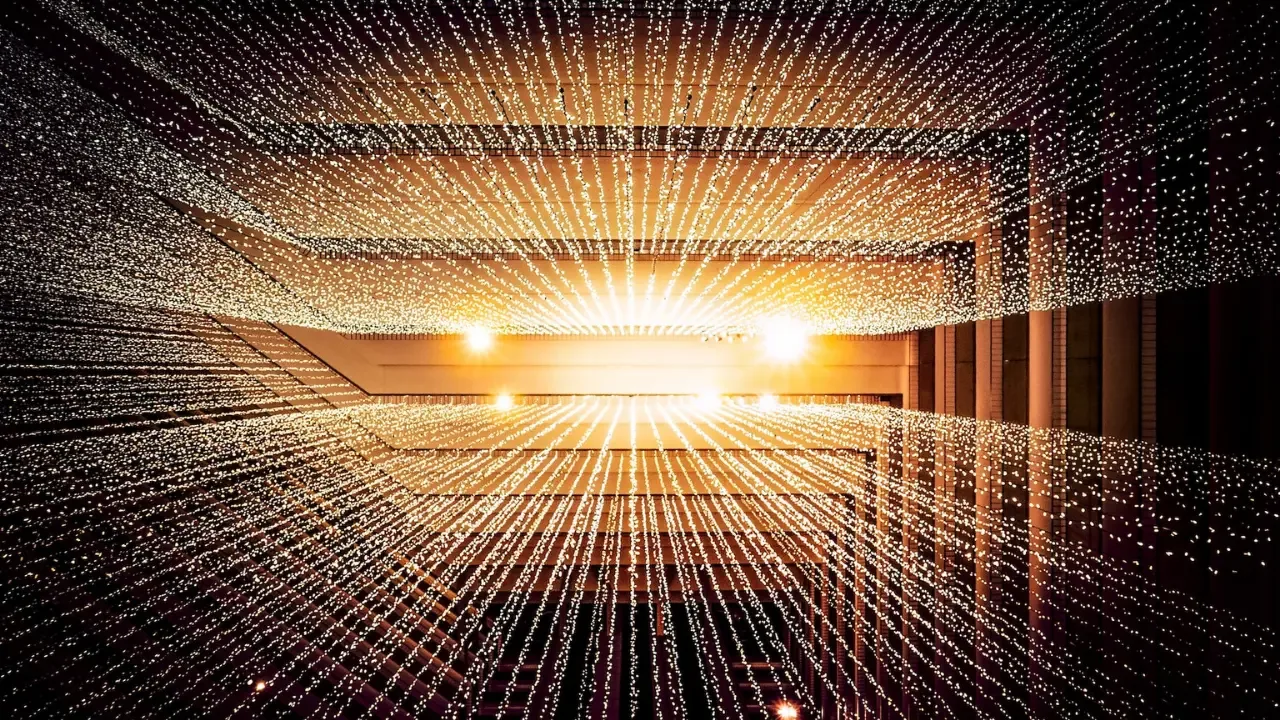
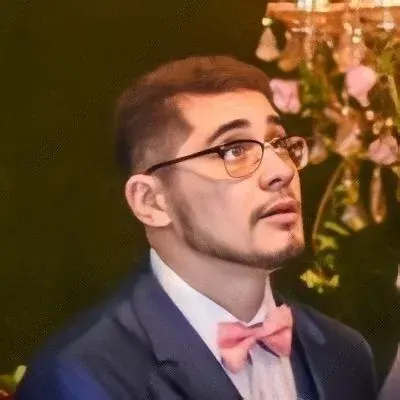
🏗️ How to Add an Auto-Increment Primary Key to an Existing SQL Server Table
So, you have an existing SQL Server table with 150,000 records, and you want to add an auto-increment primary key to it. Excellent! 👍
🧠 Understanding the Problem
The challenge here is twofold. First, you need to fill the new Id
column with incremental numbers. Second, you need to set this column as the primary key and enable the auto-increment feature. Let's break it down step by step.
💡 Easy Solutions
1. Fill the Initial Numbers
To fill the initial numbers in the Id
column, you can use the ROW_NUMBER
function combined with an UPDATE
statement. Here's an example query to get you started:
UPDATE YourTable
SET Id = NewId
FROM (
SELECT ROW_NUMBER() OVER (ORDER BY SomeColumn) AS NewId, *
FROM YourTable
) AS SubQuery
In this query:
YourTable
is the name of your existing table.SomeColumn
is the column that defines the order in which the numbers should be assigned. If you don't have any specific order, you can use any column that makes sense for your data.
Make sure to replace YourTable
and SomeColumn
with your actual table and column names. 🔄
2. Set the Primary Key and Enable Auto-Increment
Once you've filled the Id
column with the initial numbers, it's time to set it as the primary key and enable auto-increment.
Use the
ALTER TABLE
statement to add the primary key constraint, specifying the column name:
ALTER TABLE YourTable
ADD CONSTRAINT PK_YourTable PRIMARY KEY (Id)
Now, you can enable auto-increment for the
Id
column using theIDENTITY
property:
ALTER TABLE YourTable
ALTER COLUMN Id ADD IDENTITY(1,1)
The IDENTITY
property with (1,1)
ensures that the column will start at 1 and increment by 1 for each new record.
✨ Conclusion
And there you have it! Adding an auto-increment primary key to an existing SQL Server table doesn't have to be a pain. By following these easy solutions, you can seamlessly achieve your goal and maintain the integrity of your data.
Remember, you can always adapt these solutions to fit your specific requirements. So give it a try and let us know how it worked out for you! 💪
Do you have any other SQL Server questions or challenges? Share them in the comments below, and let's get the conversation rolling! 🎉