Pad a string with leading zeros so it"s 3 characters long in SQL Server 2008
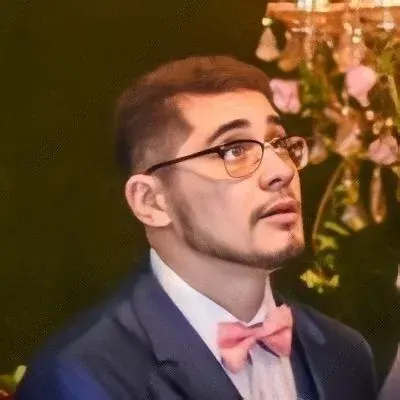
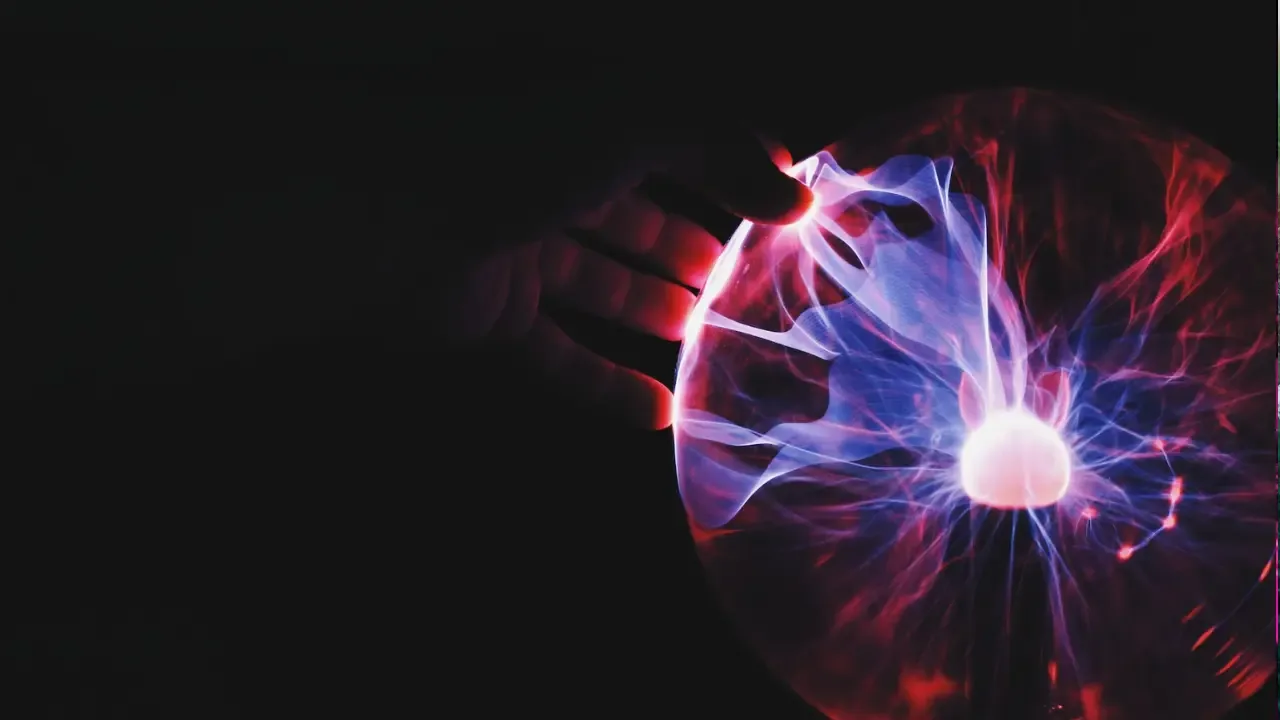
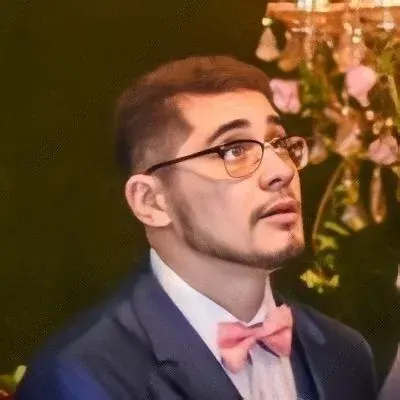
🔢 Padding a String with Leading Zeros in SQL Server 2008 R2
So you have a string in SQL Server 2008 R2 and you want to pad it with leading zeros to make it a length of 3 characters. Don't worry, we've got you covered! In this guide, we will walk you through the process of achieving this using T-SQL, step by step. Let's dive in! 👨💻
🔑 Understanding the Problem
Before we jump into the solution, let's take a moment to understand the problem at hand. The string you have can be up to 3 characters long. In cases where the original value is less than 3 characters, you want to pad it with leading zeros. However, if the original value is already 3 characters long or more, no padding is required.
💡 Easy Solutions
Here are a couple of T-SQL solutions that you can use to pad your string with leading zeros:
Solution 1 - RIGHT() and REPLICATE() Functions
SELECT RIGHT('000' + YourColumn, 3)
FROM YourTable
Let's break down this solution:
The
RIGHT()
function takes two parameters: the string expression (YourColumn
) and the number of characters to return (in this case, 3).The
REPLICATE()
function is used to generate a string of leading zeros ('000') which is then concatenated with the original value using the '+' operator.The result is a string that is always 3 characters long, with leading zeros if necessary.
Solution 2 - STUFF() Function
SELECT STUFF('000', 4 - LEN(YourColumn), LEN(YourColumn), YourColumn)
FROM YourTable
In this solution, we make use of the STUFF()
function to achieve the padding:
The first parameter is the original string of leading zeros ('000').
The second parameter calculates the starting position for the replacement based on the length of the original value (
YourColumn
).The third parameter determines the number of characters to replace, which is equal to the length of the original value.
The fourth parameter is the replacement value, which is the original value itself (
YourColumn
).
✨ Bonus Tip
If you want to handle cases where the original value is NULL or an empty string, you can use the COALESCE()
function or the ISNULL()
function in combination with the solutions mentioned above. These functions allow you to provide a default value, such as '000', when the original value is missing.
📢 Call-to-Action
Now that you know how to pad a string with leading zeros in SQL Server 2008 R2, go ahead and give it a try! Experiment with different scenarios, test out the solutions we provided, and see how it works in your specific case. And don't forget to let us know your thoughts and any questions in the comments below. Happy coding! 😄🚀