How to check if a column exists in a SQL Server table
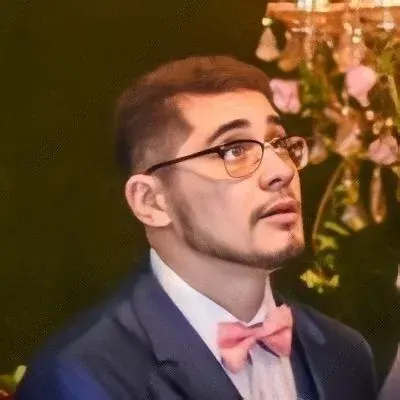
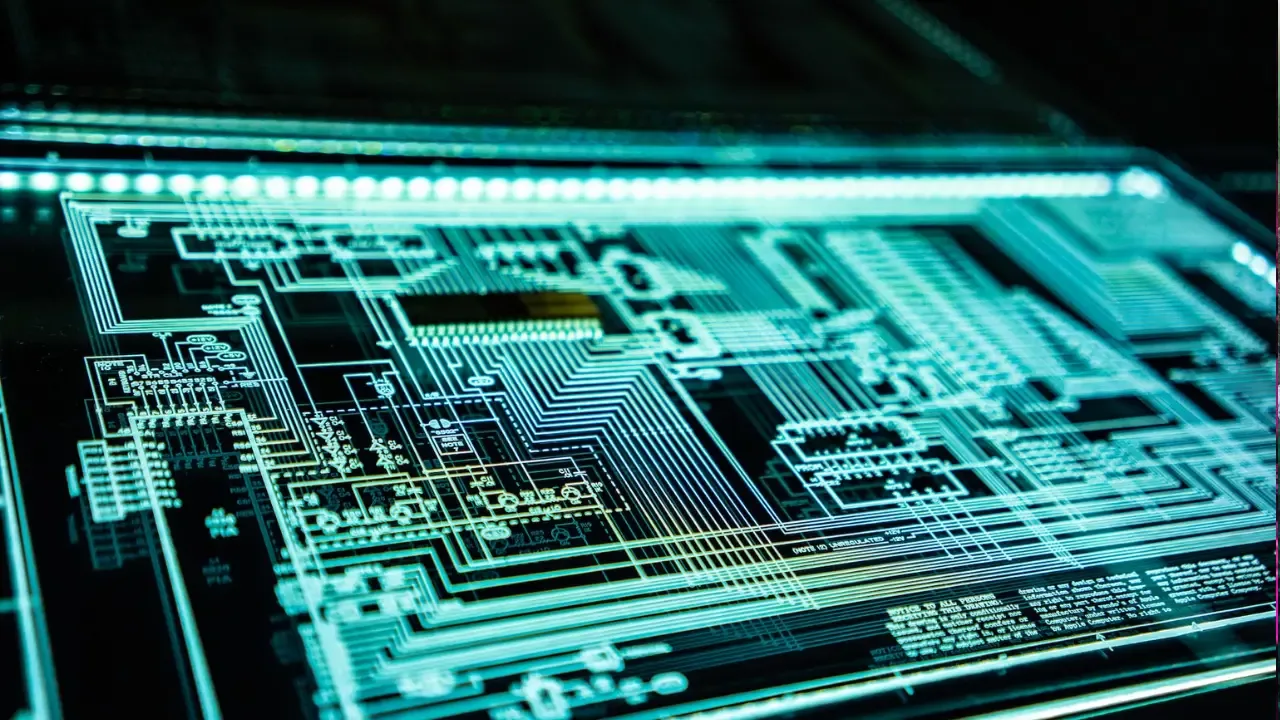
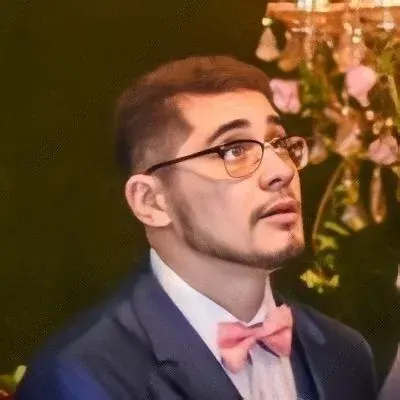
🕵️ How to Check if a Column Exists in a SQL Server Table
So you're trying to check if a specific column exists in a SQL Server table, huh? It seems like you have already tried using the IF EXISTS
statement with the INFORMATION_SCHEMA.COLUMNS
, but it's not working as expected. Don't worry, I've got you covered! In this guide, I'll walk you through some easy solutions to tackle this common problem.
📋 The Not-So-Working Solution
Let's start by taking a closer look at the code snippet you've provided:
IF EXISTS(SELECT *
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = 'myTableName'
AND COLUMN_NAME = 'myColumnName')
At first glance, this seems like the right path to follow. Unfortunately, using the INFORMATION_SCHEMA.COLUMNS
may not always yield the desired results. 😕
🚀 Solution 1: sys.columns to the Rescue!
One way to check if a column exists is to utilize the sys.columns
system view. This view contains valuable information about columns present in a SQL Server database. Here's an example of how you can use it:
IF EXISTS(SELECT *
FROM sys.columns
WHERE Name = N'myColumnName'
AND Object_ID = Object_ID(N'myTableName'))
BEGIN
-- Column exists, handle accordingly
END
By comparing the Name
column with the desired column name and the Object_ID
column with the table you want to check, you can determine if the column exists in the table. 😎
🚀 Solution 2: INFORMATION_SCHEMA.COLUMNS with Schema Name
While INFORMATION_SCHEMA.COLUMNS
may not work like a charm for some cases, adding the schema name to the mix can turn things around. Give this a shot:
IF EXISTS(SELECT *
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_SCHEMA = 'dbo'
AND TABLE_NAME = 'myTableName'
AND COLUMN_NAME = 'myColumnName')
BEGIN
-- Column exists, handle accordingly
END
Adding TABLE_SCHEMA
to the query ensures that the column name is scoped to the correct schema, making the check more accurate. 👌
🔍 Dealing with Case Sensitivity
Keep in mind that SQL Server is usually case-insensitive when it comes to querying object names. However, if you have a case-sensitive collation or have used quotes in your queries, you may experience issues with column name comparisons.
To avoid such issues, use the following query variation:
IF EXISTS(SELECT *
FROM sys.columns
WHERE LOWER(Name) = LOWER(N'myColumnName')
AND Object_ID = Object_ID(N'myTableName'))
BEGIN
-- Column exists, handle accordingly
END
By using the LOWER
function for both the column name and the desired name, you can compare them case-insensitively. This should help you tackle any case sensitivity problems that might arise. 🤓
📣 Your Turn to Take Action!
Now that you have a couple of solutions up your sleeve, it's time to put them into action! Try implementing them in your SQL Server environment and see which one works best for your scenario. Remember, understanding the structure of your database and being mindful of case sensitivity will greatly contribute to your success! 💪
If you found this guide helpful or have any other SQL-related questions, feel free to drop a comment below. Let's engage in a discussion and help each other out! 🎉
Happy querying! 🚀