How do I create a unique constraint that also allows nulls?
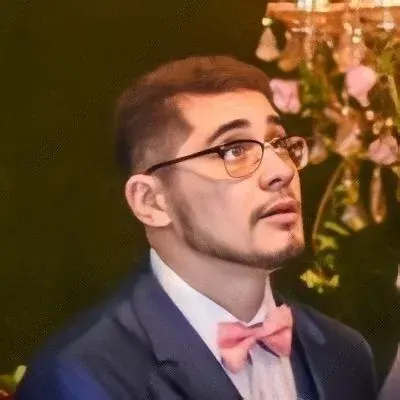
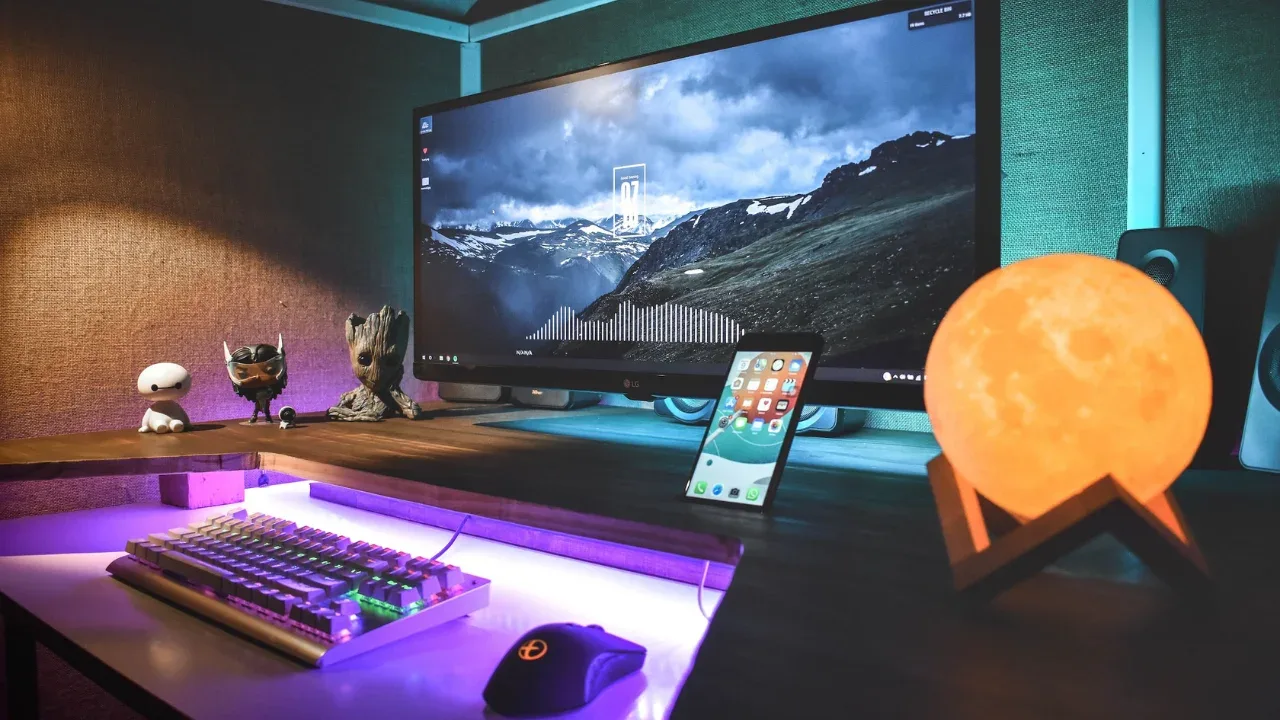
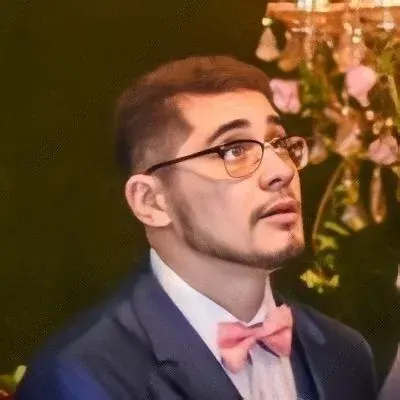
How to Create a Unique Constraint that Allows Nulls
Are you facing trouble while trying to create a unique constraint on a column that also allows null values? 🤔 Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions to overcome it. Let's dive in! 💪
Understanding the Problem
Let's start by understanding the problem statement. You want to have a unique constraint on a column that will contain GUIDs. However, your data might contain null values for this column. The challenge is to create a constraint that allows multiple null values while still ensuring uniqueness for non-null values. 🔄
Analyzing the Scenario
To help us understand the problem better, let's consider a scenario. Here's an example schema:
CREATE TABLE People (
Id INT CONSTRAINT PK_MyTable PRIMARY KEY IDENTITY,
Name NVARCHAR(250) NOT NULL,
LibraryCardId UNIQUEIDENTIFIER NULL,
CONSTRAINT UQ_People_LibraryCardId UNIQUE (LibraryCardId)
)
In this schema, we have a table called 'People' with three columns: 'Id', 'Name', and 'LibraryCardId'. The 'LibraryCardId' column allows null values and has a unique constraint applied to it.
Experimenting with Code
Now, let's look at some code to understand the issue at hand:
-- This works fine:
INSERT INTO People (Name, LibraryCardId)
VALUES ('John Doe', 'AAAAAAAA-AAAA-AAAA-AAAA-AAAAAAAAAAAA');
-- This also works fine, obviously:
INSERT INTO People (Name, LibraryCardId)
VALUES ('Marie Doe', 'BBBBBBBB-BBBB-BBBB-BBBB-BBBBBBBBBBBB');
-- This would *correctly* fail:
-- INSERT INTO People (Name, LibraryCardId)
-- VALUES ('John Doe the Second', 'AAAAAAAA-AAAA-AAAA-AAAA-AAAAAAAAAAAA');
-- This works fine first time:
INSERT INTO People (Name, LibraryCardId)
VALUES ('Richard Roe', NULL);
-- THE PROBLEM: This fails even though I'd like to be able to do this:
INSERT INTO People (Name, LibraryCardId)
VALUES ('Marcus Roe', NULL);
In the above code, we can successfully insert non-null values into the 'LibraryCardId' column, but we encounter an issue when attempting to insert multiple null values. The final statement fails with a violation of the unique key constraint.
Finding the Solution
Now that we have a clear understanding of the problem, let's explore the solutions to create a unique constraint that allows nulls:
Option 1: Modify the Unique Constraint
One approach is to modify the existing unique constraint to include an additional condition to allow multiple null values. You can achieve this by using the
WHERE
clause in the constraint definition.-- Alter the existing constraint ALTER TABLE People DROP CONSTRAINT UQ_People_LibraryCardId; -- Add the modified constraint ALTER TABLE People ADD CONSTRAINT UQ_People_LibraryCardId UNIQUE (LibraryCardId) WHERE (LibraryCardId IS NOT NULL);
By adding the
WHERE (LibraryCardId IS NOT NULL)
condition, the constraint will only be enforced for non-null values. This allows multiple null values in the column while still ensuring uniqueness for non-null values.Option 2: Create a Filtered Unique Index
Another approach is to create a filtered unique index on the 'LibraryCardId' column. This index will have a filter condition to include only non-null values, effectively allowing multiple null values.
-- Create a filtered unique index CREATE UNIQUE NONCLUSTERED INDEX UX_People_LibraryCardId_NotNull ON People (LibraryCardId) WHERE (LibraryCardId IS NOT NULL);
This filtered unique index will serve the same purpose as the modified unique constraint in Option 1.
Problem Solved! ✅
Congratulations! You now have two solutions to create a unique constraint that allows null values in your column. Choose the option that best fits your requirements and implement it in your schema.
Engage with Us!
We hope this blog post helped you solve the problem of creating a unique constraint that also allows nulls in SQL. If you have any questions or insights to share, we'd love to hear from you! Leave a comment below or reach out to us on social media. Let's continue the conversation! 🎉
*[SQL]: Structured Query Language