How can I select from list of values in SQL Server
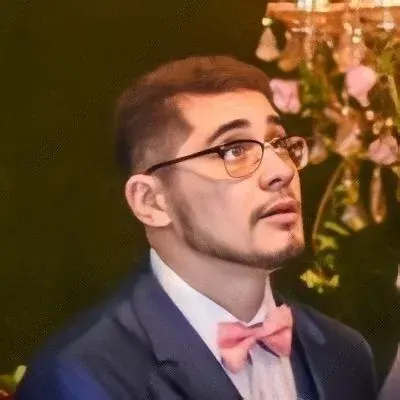
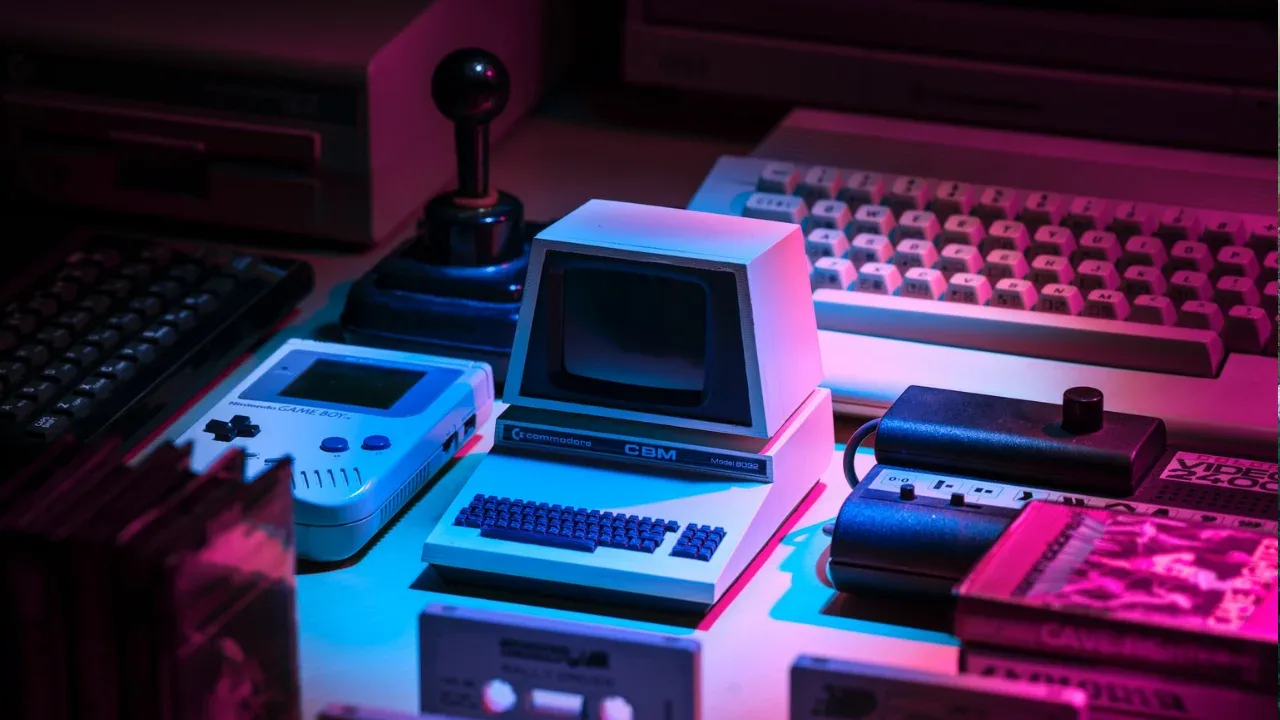
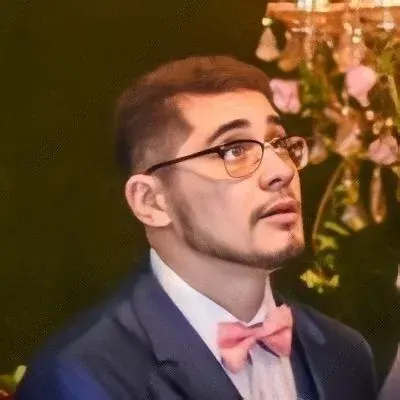
š Blog Post: How to Select from a List of Values in SQL Server like a Pro!
š Hey there SQL enthusiasts! š©āš»
We've all been there. Staring at the screen, scratching our heads, trying to figure out how to select from a list of values in SQL Server. But fear not, because I'm here to guide you through this common issue and provide easy solutions that will have you querying like a boss in no time! šŖ
š” The Problem:
So, you have a list of values and you want to select only the distinct ones. Our friend here was facing the same challenge:
select distinct * from (1, 1, 1, 2, 5, 1, 6).
But alas, it's not a valid SQL statement. š
š¤ The Solution:
1ļøā£ Option 1: Using the VALUES Clause
To select from a list of values, you can use the VALUES clause. It allows you to specify multiple rows in a single statement. š
SELECT *
FROM (VALUES (1), (1), (1), (2), (5), (1), (6)) AS MyTable(columnName)
Here, we create a table alias "MyTable" with a column named "columnName". Each row in the VALUES clause represents a distinct value.
2ļøā£ Option 2: Using a Temporary Table
Another approach is to create a temporary table and insert the values into it. This allows you to manipulate the data more easily and reuse it in multiple queries. šļø
CREATE TABLE #MyValues (columnName INT)
INSERT INTO #MyValues (columnName)
VALUES (1), (1), (1), (2), (5), (1), (6);
SELECT DISTINCT columnName
FROM #MyValues;
In this example, we create a temporary table "#MyValues" with a column named "columnName". We then insert the values into the table and select the distinct values from it.
3ļøā£ Option 3: Using a CTE (Common Table Expression)
If you prefer a cleaner and more elegant solution, you can use a CTE. This allows you to define a temporary result set that can be used within the scope of a single SQL statement. š
WITH MyCTE AS (
SELECT columnName
FROM (VALUES (1), (1), (1), (2), (5), (1), (6)) AS MyTable(columnName)
)
SELECT DISTINCT columnName
FROM MyCTE;
Here, we define a CTE named "MyCTE" that selects the distinct values from the VALUES clause. We then select the distinct values from the CTE.
š£ Take Action:
Now that you know how to select from a list of values in SQL Server, it's time to put your newfound knowledge to use! Try these solutions on your own and see the magic happen. Don't forget to share your success stories and any other SQL tips you might have in the comments below. Let's learn and grow together! š
Remember, SQL querying can be challenging, but with the right techniques, you can overcome any obstacle. Happy coding, my fellow SQL warriors! š»
Disclaimer: Always make sure to test and validate your SQL statements before deploying them in a production environment.
--
š Further Reading:
If you're hungry for more SQL knowledge, check out these helpful resources:
Note: The examples provided assume you're running SQL Server. Keep in mind that syntax may vary slightly based on your specific database system.