How can I remove duplicate rows?
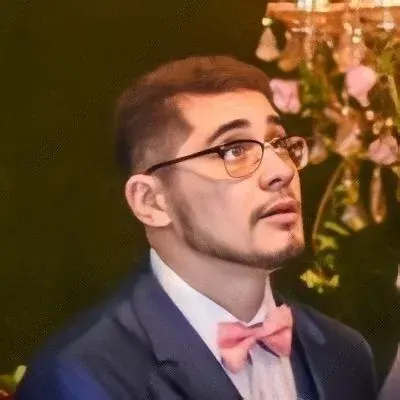
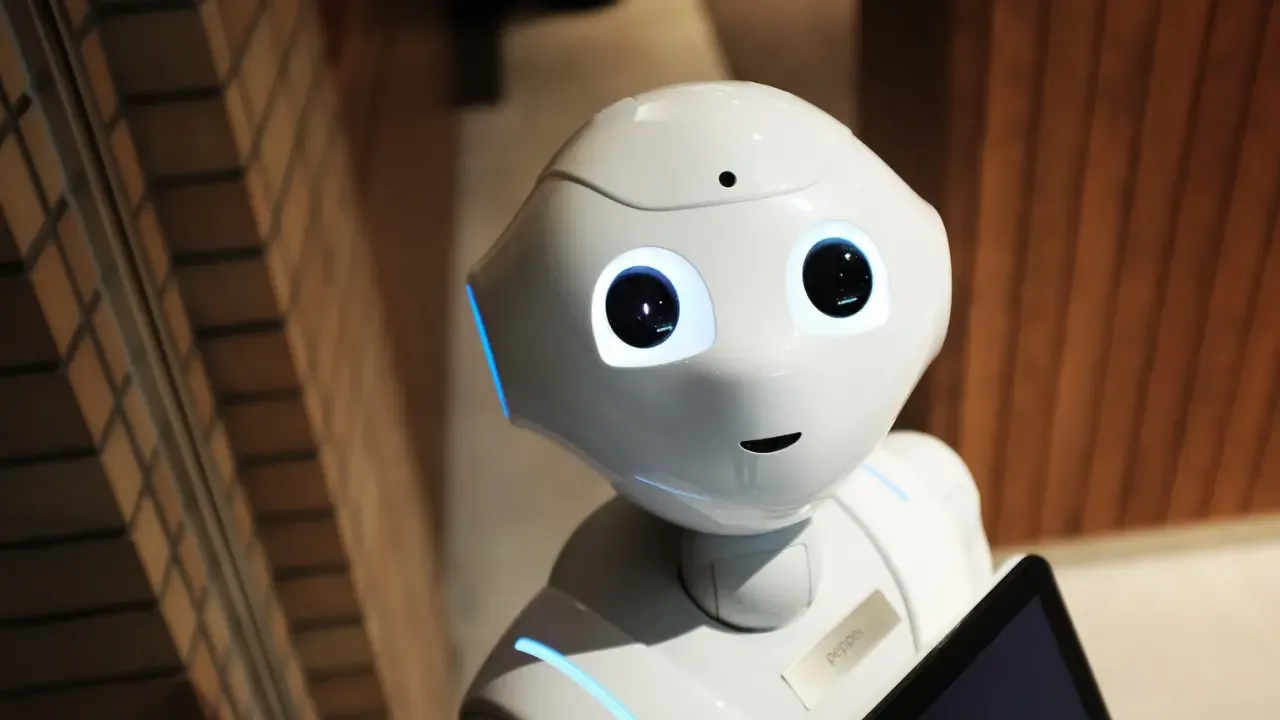
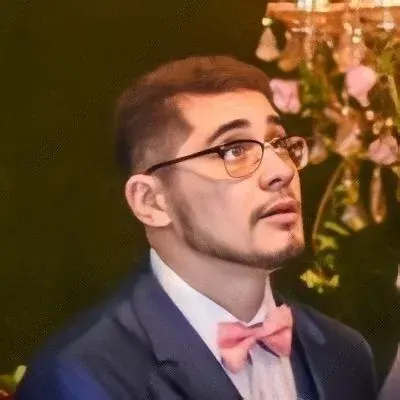
🔄 How to Remove Duplicate Rows in SQL Server Table
Are you tired of dealing with duplicate rows in your SQL Server table? 😫 Don't worry, we've got you covered! In this guide, we'll walk you through the process of removing duplicate rows from your table, even if it contains a large number of rows. Let's dive in!
💡 Understanding the Problem
Before we get started, let's quickly understand the problem at hand. You have a SQL Server table with over 300,000 rows, and you want to remove duplicate rows based on certain columns, but not including the RowID
identity field. Makes sense, right? Let's take a look at the table structure:
MyTable
RowID int not null identity(1,1) primary key,
Col1 varchar(20) not null,
Col2 varchar(2048) not null,
Col3 tinyint not null
🔍 Finding Duplicate Rows
To remove duplicate rows, we first need to identify them. One way to do this is by using the ROW_NUMBER()
function along with the PARTITION BY
clause. Here's an example query that does just that:
WITH CTE AS (
SELECT *,
ROW_NUMBER() OVER (
PARTITION BY Col1, Col2, Col3
ORDER BY RowID -- or any other column(s) that determine the preferred row
) AS RowNumber
FROM MyTable
)
SELECT *
FROM CTE
WHERE RowNumber > 1 -- Retrieve only the duplicate rows
This query groups the rows based on the specified columns (Col1
, Col2
, Col3
), orders them by the RowID
(or any other preferred column), and assigns a row number to each group. Rows with a RowNumber
greater than 1 are considered duplicates.
🗑️ Removing Duplicate Rows
Now that we have identified the duplicate rows, it's time to remove them from the table. We can achieve this by using the DELETE
statement with a subquery. Here's an example:
WITH CTE AS (
SELECT *,
ROW_NUMBER() OVER (
PARTITION BY Col1, Col2, Col3
ORDER BY RowID
) AS RowNumber
FROM MyTable
)
DELETE FROM CTE
WHERE RowNumber > 1
This query uses the same approach as before to identify the duplicate rows, but instead of selecting them, we directly delete them from the CTE
(Common Table Expression) subquery. Easy peasy!
🏁 Wrapping Up
And there you have it! You now know how to remove duplicate rows from your SQL Server table, no matter how big it is. Just follow the steps we discussed: find the duplicates using the ROW_NUMBER()
function, and delete them using the DELETE
statement with a subquery.
Now it's time for you to put this knowledge into action and declutter your database. Remember to take a backup of your table before performing any deletions, just in case.
If you found this guide helpful, don't forget to share it with your friends and colleagues who might be struggling with duplicate rows. And if you have any questions or cool tips to share, drop them in the comments below. Happy coding! 🚀