Search text in stored procedure in SQL Server
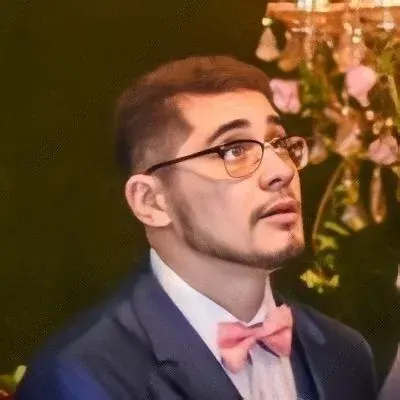
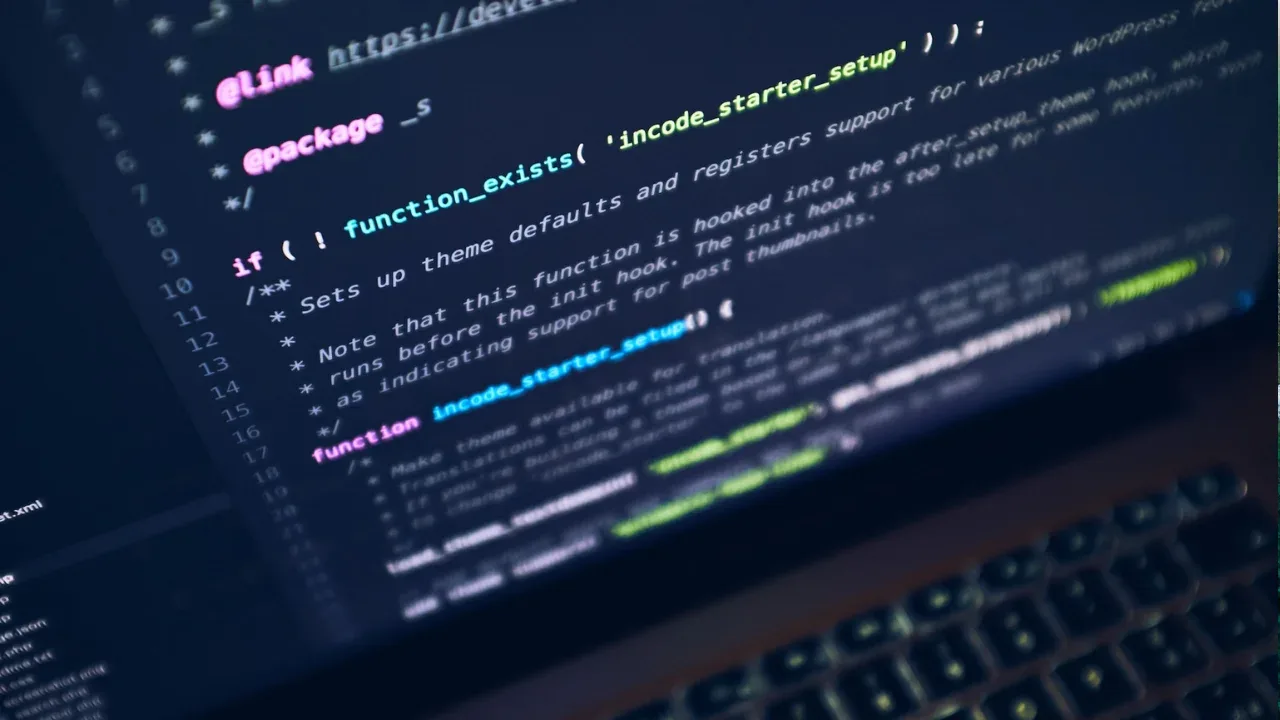
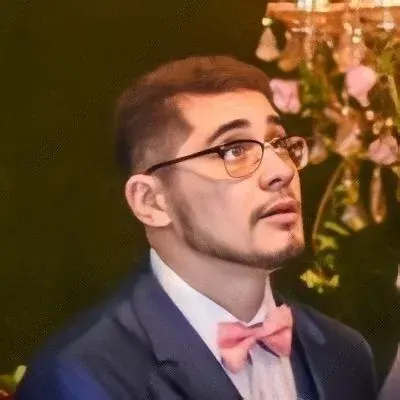
📝 Blog Post: How to Search Text in Stored Procedures in SQL Server
Are you struggling to search for a specific text in your SQL Server stored procedures? 🤔 Don't worry, we've got you covered! In this guide, we'll address the common issue users face when searching for text in stored procedures and provide an easy solution. Let's dive in and find that needle in the haystack! 💪
The Misleading Query
The first thing you need to know is that the query you're using, which looks like this:
SELECT DISTINCT
o.name AS Object_Name,
o.type_desc
FROM sys.sql_modules m
INNER JOIN
sys.objects o
ON m.object_id = o.object_id
WHERE m.definition Like '%[ABD]%';
may seem like it should do the trick. The LIKE
operator with the [ABD]
pattern seems like a perfect fit to search for the desired text. However, this is where things get a little tricky. 😅
The Square Brackets Dilemma
The issue lies in the fact that square brackets in the LIKE
pattern have a special meaning in SQL Server. They are used to define character ranges, making the query look for any occurrence of 'A', 'B', or 'D' in the text. 😱 As a result, the query won't give you the proper result you're looking for.
The Solution: Escaping the Square Brackets
To make the query work as expected, you need to escape the square brackets by using the ESCAPE
clause. Here's an updated version of the query:
SELECT DISTINCT
o.name AS Object_Name,
o.type_desc
FROM sys.sql_modules m
INNER JOIN
sys.objects o
ON m.object_id = o.object_id
WHERE m.definition LIKE '%![ABD]!%' ESCAPE '!';
By adding the ESCAPE
clause and specifying the exclamation mark (!) as the escape character, the query now looks for the exact occurrence of '[ABD]' in the stored procedure text. 🙌
Take It a Step Further
Now that you have the solution to your problem, there's no reason to stop there. You can enhance your search by modifying the query to be more flexible and enable case-insensitive searching. Here's an example:
SELECT DISTINCT
o.name AS Object_Name,
o.type_desc
FROM sys.sql_modules m
INNER JOIN
sys.objects o
ON m.object_id = o.object_id
WHERE LOWER(m.definition) LIKE '%![abd]!%' ESCAPE '!';
In this updated version, we've made the comparison case-insensitive by converting the stored procedure text to lowercase using the LOWER()
function. This allows for a more comprehensive search. 👍
Your Turn to Search!
Now it's your turn to put this knowledge into action! Go ahead and modify the query according to your needs and start searching for that elusive text in your stored procedures. Remember to escape those square brackets and consider making your search case-insensitive for more accurate results. 🔍
And don't forget to share your success story in the comments below! We'd love to hear how this solution worked for you. 😊
Keep coding and happy searching! 💻🔎
*[ABD]: Specific characters or pattern you're searching for in the stored procedures