Rails raw SQL example
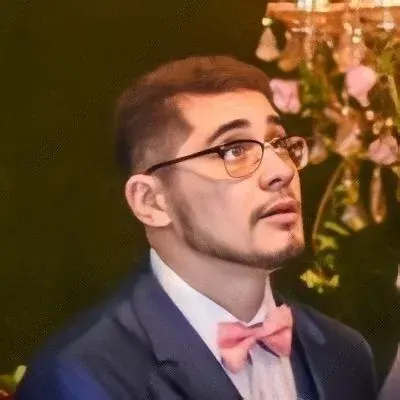
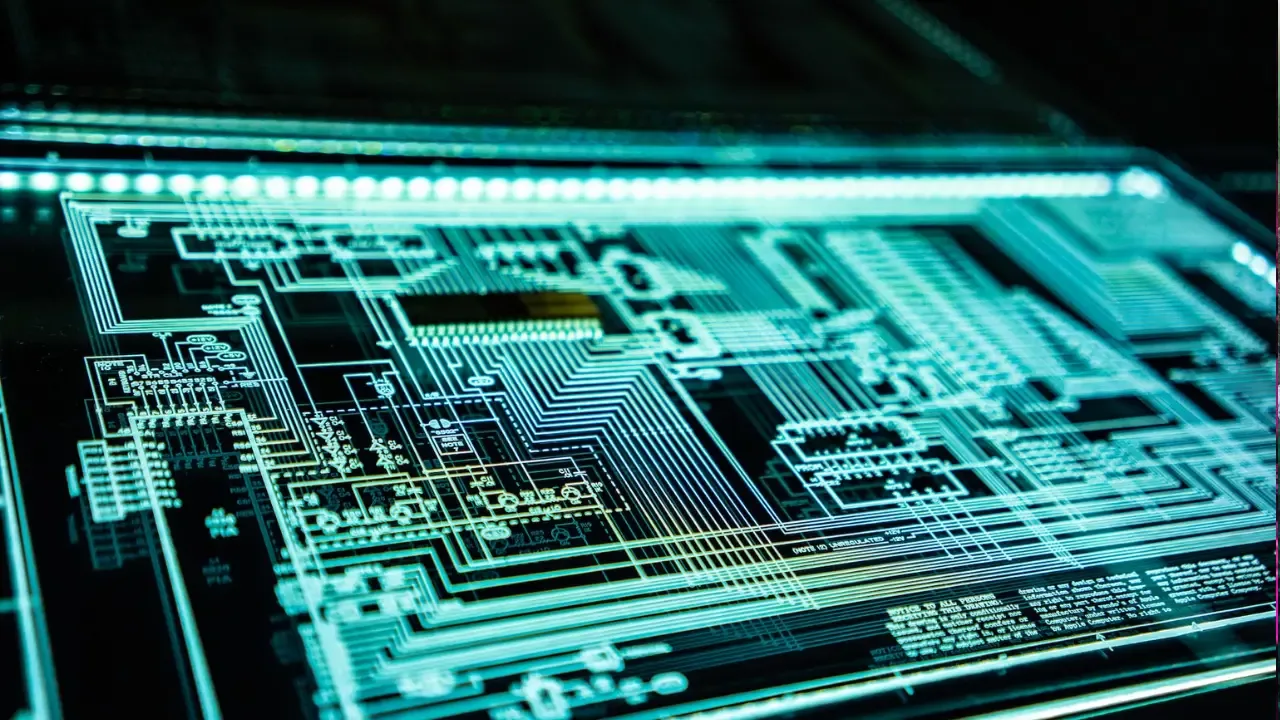
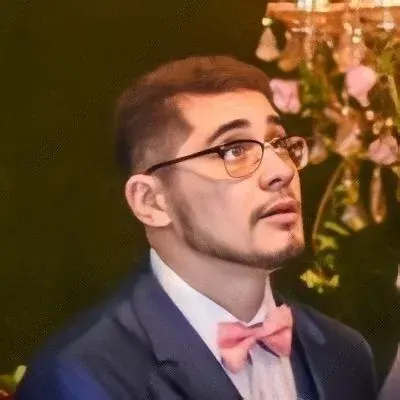
🔍 Rails raw SQL example: Boosting Performance and Resolving Timeout Errors
If you're facing a request timeout error while deploying your code to Heroku, converting your Rails code to raw SQL can be a viable solution to speed up your application. In this blog post, we'll explore how to optimize your code and address the common issues associated with using raw SQL in Rails. Let's dive in! 🏊♀️
Understanding the Code 🤔
To begin our journey, let's examine the code snippet you provided:
@payments = PaymentDetail.joins(:project).order('payment_details.created_at desc')
@payment_errors = PaymentError.joins(:project).order('payment_errors.created_at desc')
@all_payments = (@payments + @payment_errors)
Here, we can see that you're retrieving payment details, payment errors, and combining them into a single @all_payments
variable. However, if this code is causing a request timeout error, it's time to optimize it! 💪
Using Raw SQL to Optimize Performance ⚡
To translate the above Rails code into raw SQL, we need to understand the underlying database schema and structure. Assuming the payments
and payment_errors
tables have a common project_id
column, we can rewrite the code as follows:
raw_sql = <<-SQL
SELECT payment_details.*
FROM payment_details
INNER JOIN projects ON projects.id = payment_details.project_id
UNION
SELECT payment_errors.*
FROM payment_errors
INNER JOIN projects ON projects.id = payment_errors.project_id
ORDER BY created_at desc
SQL
@all_payments = ActiveRecord::Base.connection.execute(raw_sql)
In this snippet, we're using a raw SQL query that performs a UNION operation between the payment_details
and payment_errors
tables, then ordering the results by created_at
in descending order.
By executing this raw SQL query, you can significantly improve the efficiency of your code and potentially resolve the request timeout error you encountered during deployment. 🚀
Going the Extra Mile: Addressing Potential Risks 🚧
While using raw SQL can provide performance benefits, it's important to be aware of potential risks associated with this approach. Here are a few points to consider:
SQL Injection: Be cautious when handling user input in your raw SQL queries. Always use parameterized queries or sanitization methods to prevent SQL injection attacks.
Database Portability: Raw SQL queries may optimize performance for a specific database, but they can limit your application's portability across different database engines. Ensure that your raw SQL code is compatible with all target databases.
Maintenance and Readability: Raw SQL queries can become hard to maintain and understand over time, especially for complex queries. Ensure you adequately document and test your code to minimize potential issues.
Conclusion and Call-to-Action ✨
We've explored how to convert your Rails code to raw SQL to improve performance and resolve a request timeout error on Heroku. By optimizing your code with a raw SQL query, you can enhance your application's efficiency and handle larger datasets effectively.
If you found this blog post helpful, share it with your fellow Rails developers and let them unleash the power of raw SQL. 💡 Leave a comment below with your questions, thoughts, or any alternative solutions you've found. Happy coding! 😊✍️