Parameterize an SQL IN clause
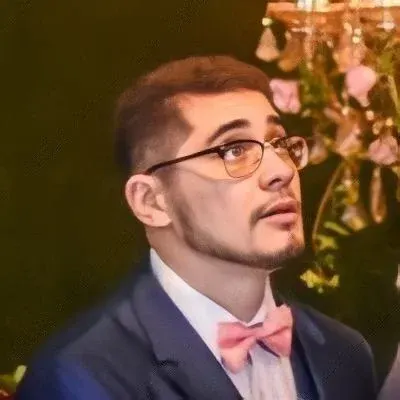
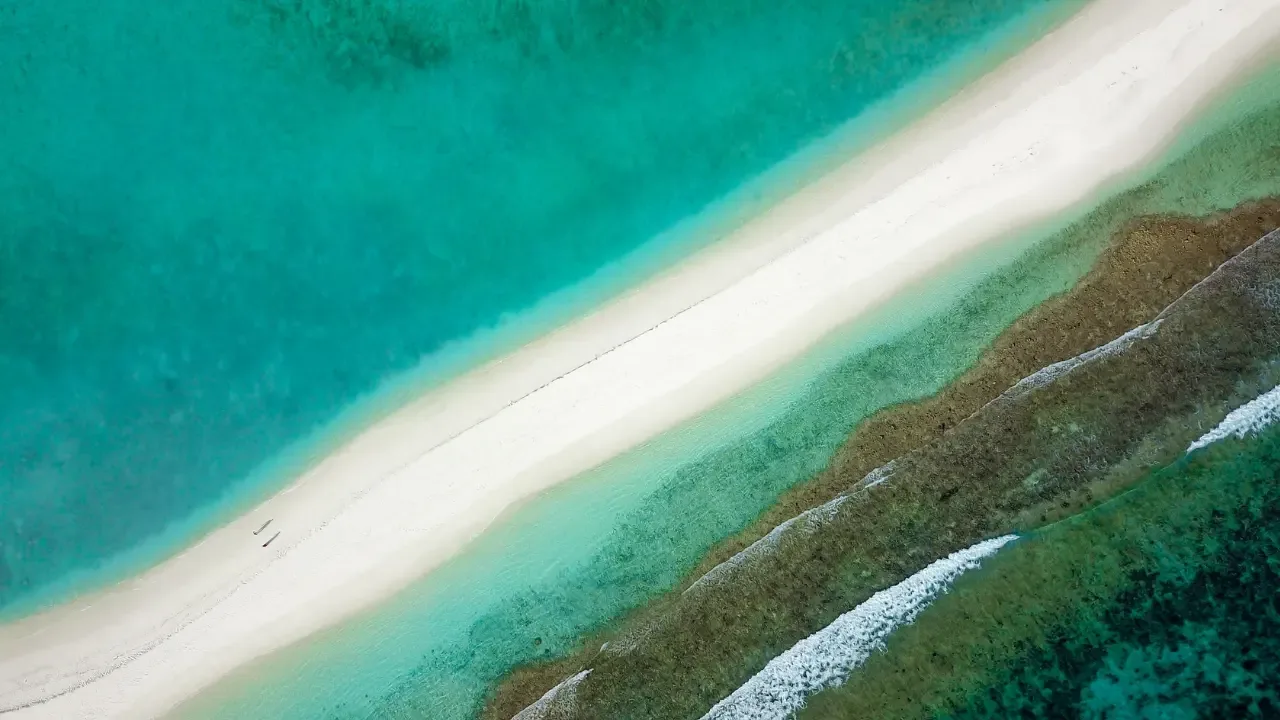
Parameterizing an SQL IN Clause: A Simple Guide 👨💻🔧
So you want to parameterize an SQL query with an IN clause that has a variable number of arguments? 🤔 No worries, we've got you covered! In this blog post, we'll walk you through common issues, provide easy solutions, and offer a compelling call-to-action to keep you engaged. Let's dive in! 💪
Understanding the Problem 😓
Let's first take a look at the query you're dealing with:
SELECT * FROM Tags
WHERE Name IN ('ruby','rails','scruffy','rubyonrails')
ORDER BY Count DESC
The challenge arises when the number of arguments within the IN clause can vary from 1 to 5. 😫 We want to find an elegant solution without resorting to dedicated stored procedures or XML.
Easy Solutions, Effortless Results 💡
To parameterize an SQL IN clause with a variable number of arguments, consider the following solutions:
1. Dynamic SQL Generation
One approach is to dynamically generate the SQL statement based on the number of arguments. Using a programming language or stored procedure, construct the query string with the appropriate number of placeholders. Then, bind the values to those placeholders at runtime. Here's an example using pseudo code:
num_args = 4
query = "SELECT * FROM Tags WHERE Name IN ("
placeholders = ','.join(['?']*num_args)
query += placeholders + ") ORDER BY Count DESC"
2. Table Valued Parameters (TVPs)
If you're using SQL Server 2008 or later, you can leverage Table Valued Parameters (TVPs) to handle the variable number of arguments efficiently. TVPs allow you to pass a table structure as a parameter to a stored procedure. Here's how you can use TVPs in this scenario:
Create a User-Defined Table Type to represent the values you want to pass.
Use the TVP as a parameter in your stored procedure, enabling it to accept multiple values.
Inside the stored procedure, join the TVP with your main query using the IN clause.
3. Splitting and Joining Strings
If you're working with a database that doesn't support TVPs or dynamic SQL generation, you can split the input string into individual values and join them with the IN clause. While this method may not be the most efficient, it can be effective for smaller datasets. Here's a rudimentary example in SQL Server:
DECLARE @tags nvarchar(max) = 'ruby,rails,scruffy,rubyonrails'
SELECT *
FROM Tags
WHERE CHARINDEX(Name, @tags) > 0
ORDER BY Count DESC
Take Action and Engage! 🚀
Now that you have these easy solutions, it's time to put them to use! Decide which method works best for your specific scenario and implement it in your SQL code. Remember to consider factors such as performance, scalability, and compatibility with your database system.
Have you encountered any other challenges in parameterizing SQL queries? Share your thoughts, experiences, and insights in the comments below! Let's learn together! 😊💬
Conclusion
Parameterizing an SQL IN clause with a variable number of arguments doesn't have to be a headache. With dynamic SQL generation, Table Valued Parameters, or string splitting/joining, you can tackle this problem easily. Choose the solution that suits your needs best, and happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
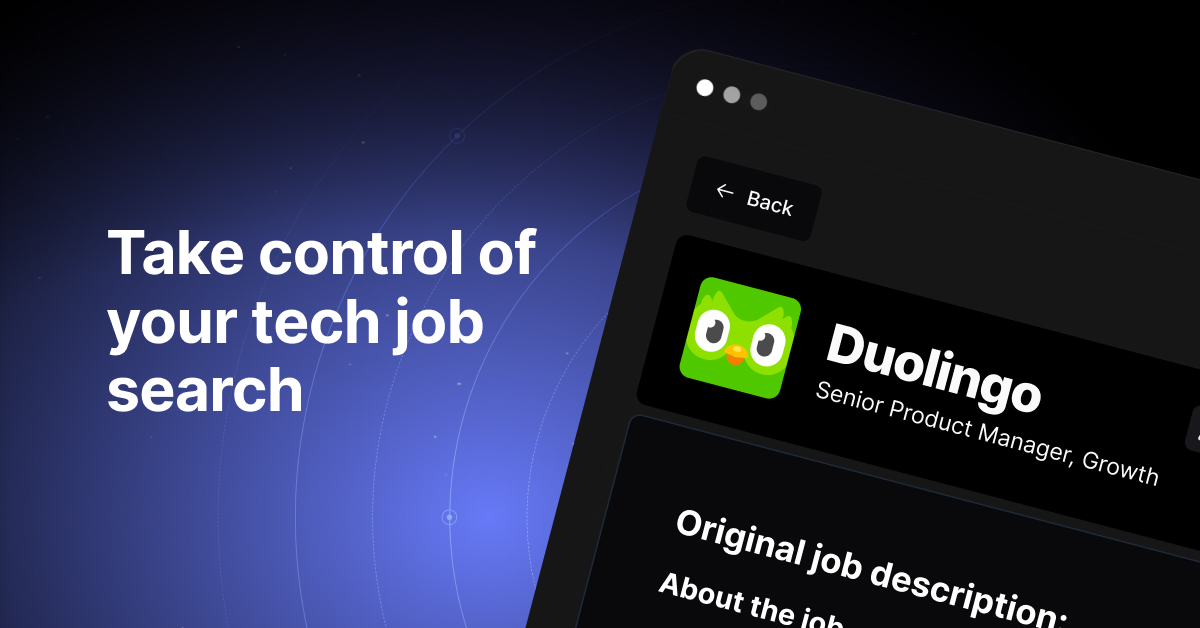