How to set variable from a SQL query?
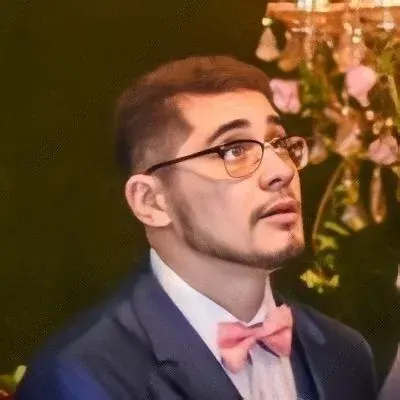
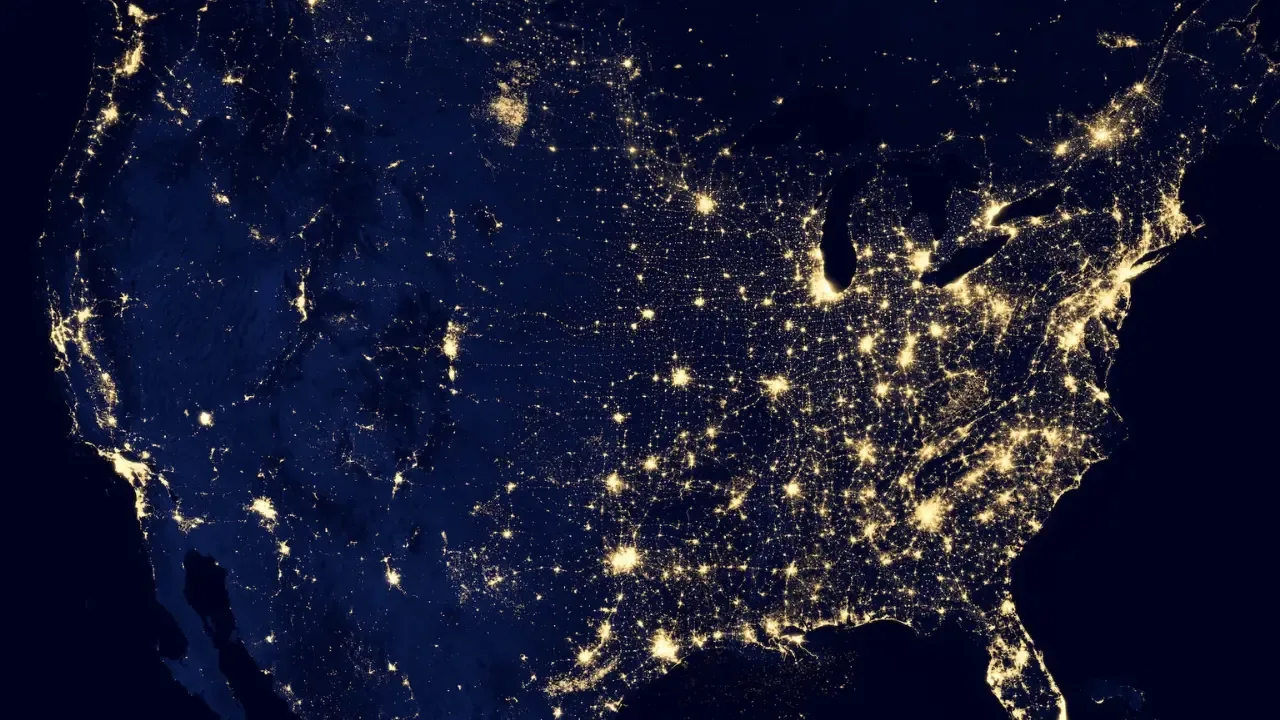
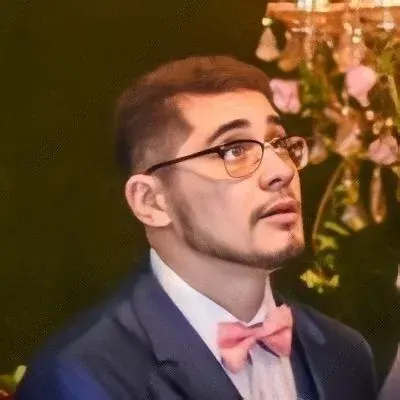
How to Set a Variable from a SQL Query: A Step-by-Step Guide
So you're trying to set a variable from a SQL query but running into issues? Don't worry, you're not alone! This is a common problem that many developers face. In this guide, we'll walk you through the process and provide easy solutions to help you achieve your goal. Let's get started!
The Issue at Hand
First, let's take a look at the code snippet you provided:
declare @ModelID uniqueidentifer
Select @ModelID = select modelid from models
where areaid = 'South Coast'
The error is clear - you're trying to assign the result of a query to a variable, but the syntax is incorrect. Don't worry, we've got you covered with some simple fixes!
Solution 1: Using the SELECT INTO Statement
To set a variable from a query, you can use the SELECT INTO
statement. This statement allows you to assign the result of a query to a variable in a single line. Here's how you can modify your code to make it work:
declare @ModelID uniqueidentifier
Select @ModelID = modelid
into @ModelID
from models
where areaid = 'South Coast'
By using the SELECT INTO
statement, you can now correctly assign the value to the @ModelID
variable.
Solution 2: Using the SET Statement
Another way to achieve the same result is by using the SET
statement. This method is often preferred when dealing with single-row queries. Here's how you can modify your code using the SET
statement:
declare @ModelID uniqueidentifier
SET @ModelID = (select modelid from models where areaid = 'South Coast')
With the SET
statement, the result of the subquery is assigned directly to the @ModelID
variable.
Solution 3: Handling Multiple Rows
If your query returns multiple rows and you want to assign all the values to a variable, you can use a table variable or a temporary table. Here's an example using a table variable:
declare @ModelIDs table (modelid uniqueidentifier)
insert into @ModelIDs (modelid)
select modelid from models where areaid = 'South Coast'
-- You can now access each value individually using a loop or perform further operations on the @ModelIDs table variable
By inserting the query results into a table variable, you can store and use the values as needed.
Your Turn to Explore!
Now that you have learned three different solutions to set variables from SQL queries, it's time to put your newfound knowledge to work in your own projects. Experiment with these techniques, and feel free to share your experiences and any additional tips you may have in the comments below!
Conclusion
Setting a variable from a SQL query may seem tricky at first, but with the right syntax and knowledge, it's a breeze! In this guide, we covered three easy solutions to help you achieve this goal. Remember to use the SELECT INTO
statement or the SET
statement, depending on your specific requirements. And if you're dealing with multiple rows, don't forget to explore table variables or temporary tables.
We hope this guide has been helpful and has provided you with a clear understanding of how to set variables from SQL queries. If you have any questions or further insights, don't hesitate to reach out. Happy coding!
Did you find this guide helpful? Let us know in the comments below and share it with your fellow developers! 🚀️📝️