How to select from subquery using Laravel Query Builder?
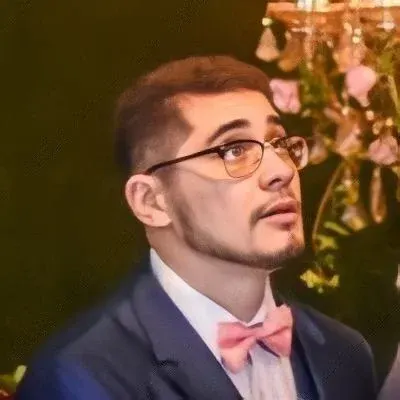
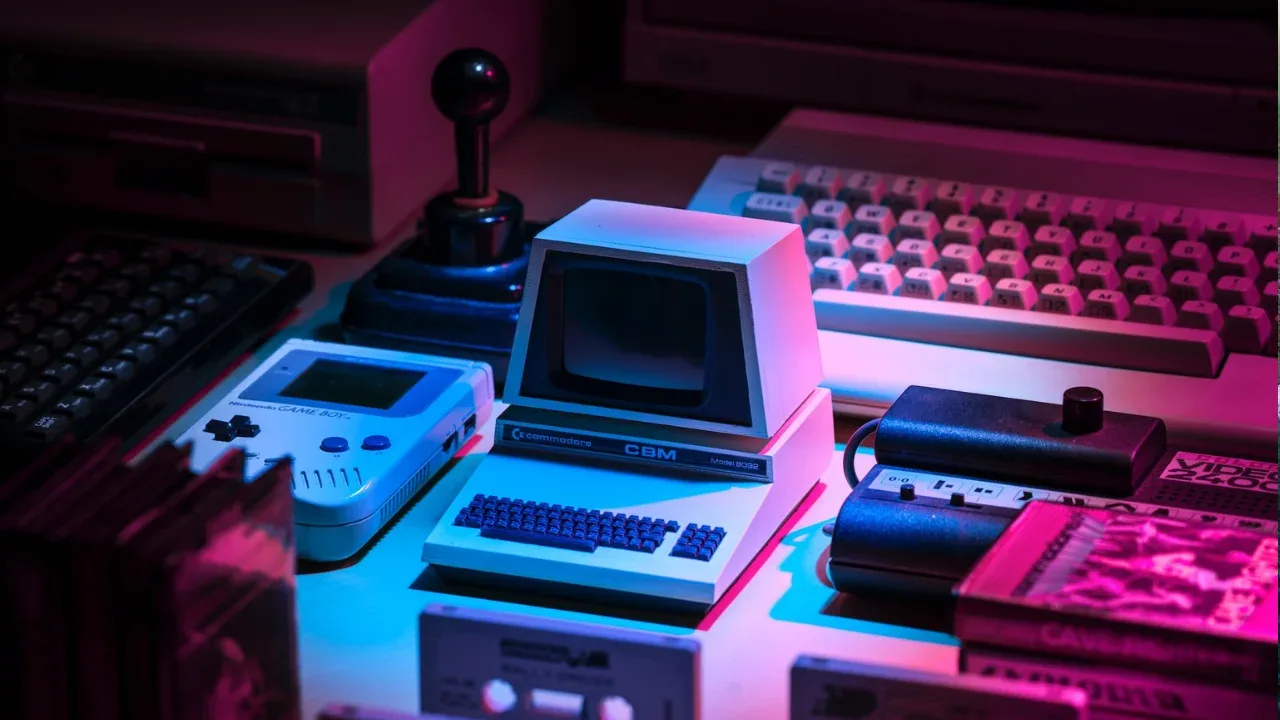
🚀 How to Select from Subquery using Laravel Query Builder?
So, you want to know how to select from a subquery using Laravel Query Builder? 🤔 Don't worry, I've got you covered! In this blog post, I will address this common issue and provide you with some easy solutions that will save your day. Let's dive right in, shall we? 💪
💡 Understanding the Problem
First, let's understand the problem at hand. The original SQL query you provided looks like this:
SELECT COUNT(*) FROM
(SELECT * FROM abc GROUP BY col1) AS a;
The goal is to achieve the same result using Laravel's Query Builder, specifically Eloquent ORM. The sample code you presented is close, but you're looking for an even simpler solution.
⚙️ The Existing Solution
Currently, you have the following code:
$sql = Abc::from('abc AS a')->groupBy('col1')->toSql();
$num = Abc::from(\DB::raw($sql))->count();
print $num;
While this code will do the job, there is indeed a simpler way to accomplish the same result. Let's check it out! 😎
🚀 The Simpler Solution
To achieve the desired result with less code, you can use a subquery directly in the Laravel Query Builder. Here's how it works:
$num = DB::table(function ($query) {
$query->from('abc')->groupBy('col1');
}, 'a')->count();
print $num;
That's it! With just a few lines of code, you can select from a subquery using Laravel Query Builder. Pretty neat, right? 😉
🤝 Call-to-Action
I hope this blog post has helped you simplify the process of selecting from a subquery using Laravel Query Builder. Now, it's your turn to try it out! Implement this solution in your project and let me know how it goes. If you have any questions or concerns, feel free to leave a comment below. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
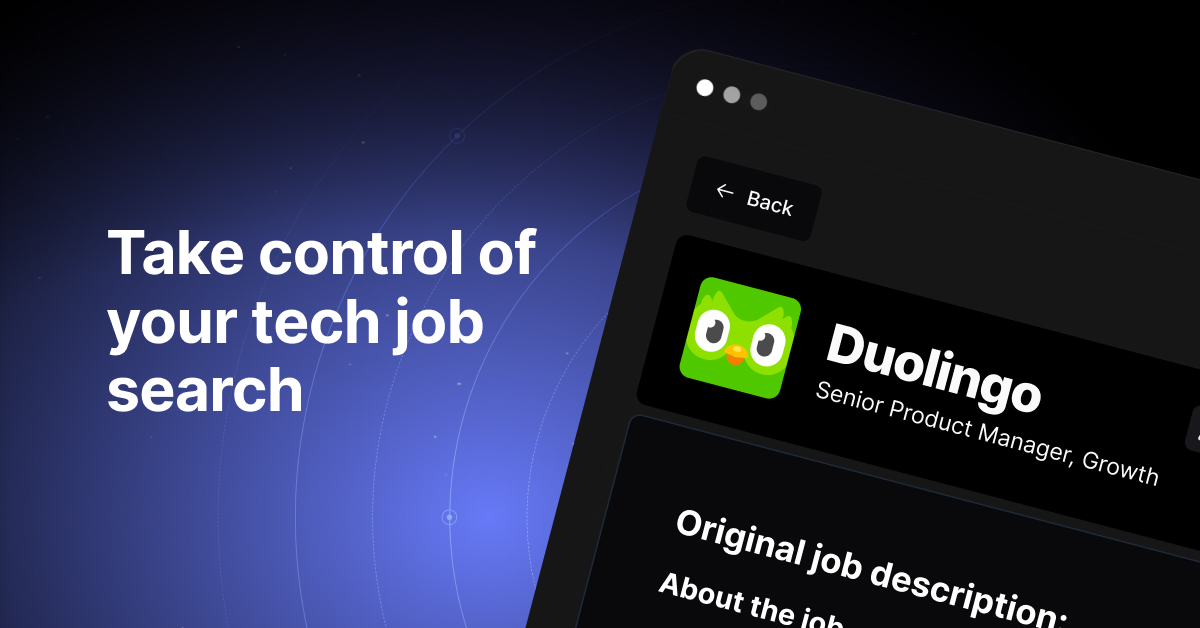