How to SELECT FROM stored procedure
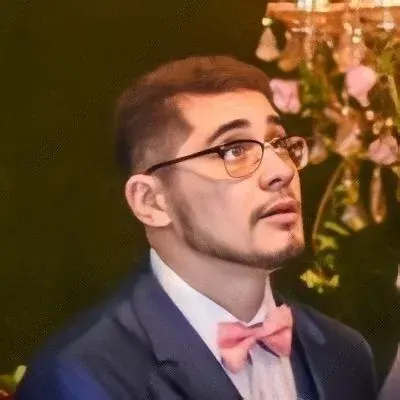
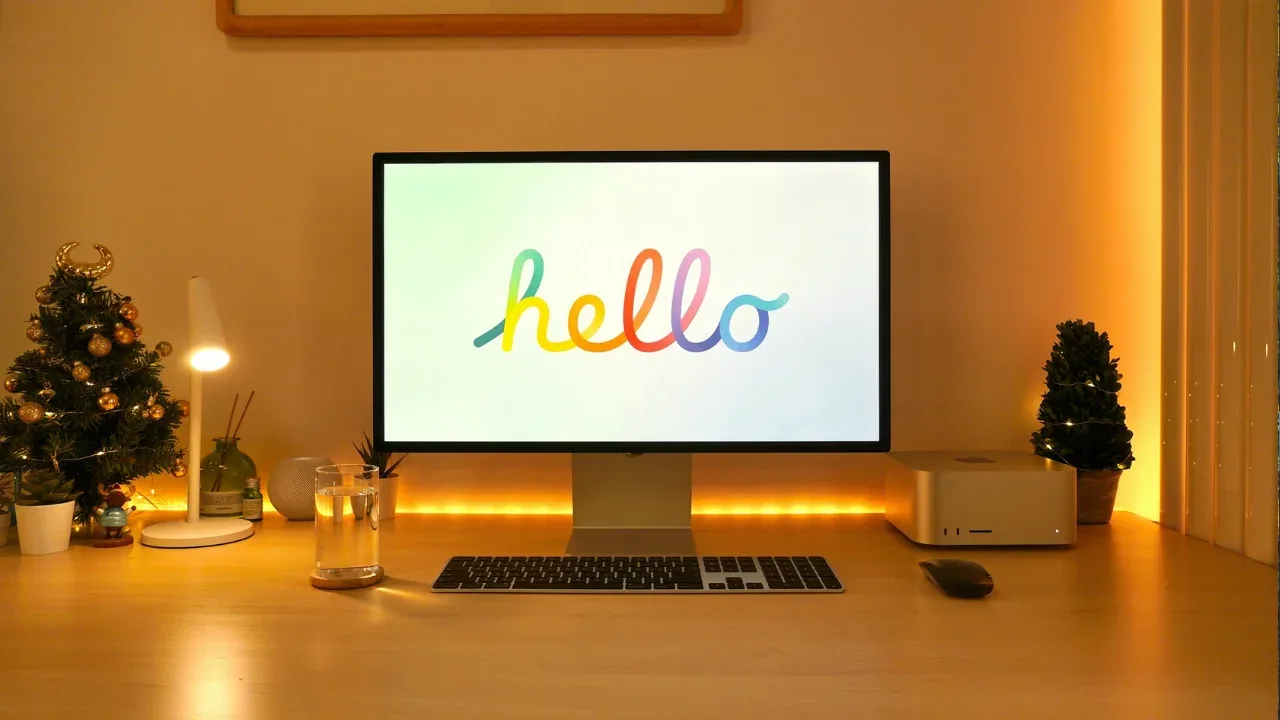
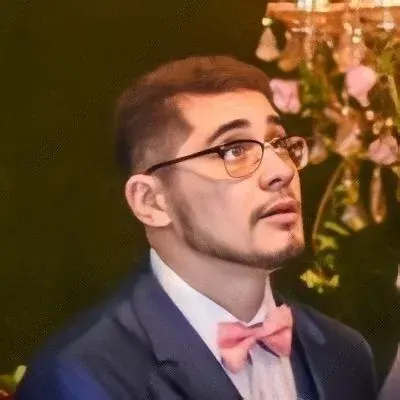
How to SELECT FROM stored procedure ๐๐ป
So, you've got a stored procedure that is returning rows? ๐ค And now you're wondering if it's possible to select the output by calling this procedure directly, without any additional hassle? ๐คทโโ๏ธ Well, you're in luck! In this blog post, we'll explore common issues, provide easy solutions, and show you how to do exactly that! ๐ช
The Stored Procedure ๐งช
Let's take a look at the stored procedure in question:
CREATE PROCEDURE MyProc
AS
BEGIN
SELECT * FROM MyTable
END
Seems pretty straightforward, right? But what if you want to select the output of this procedure? Can we simply do something like this?
SELECT * FROM (EXEC MyProc) AS TEMP
The Challenge ๐ค
As much as we would love for this solution to work seamlessly, unfortunately, it's not that simple. ๐ The syntax SELECT * FROM (EXEC MyProc)
won't give you the desired result. But fear not! We have a couple of simple solutions to this problem. ๐
Solution 1: Using INSERT INTO ๐ฅ
One way to achieve the desired result is by using the INSERT INTO
statement. Here's how it works:
CREATE TABLE #TempTable (
-- Define columns here
)
INSERT INTO #TempTable
EXEC MyProc
SELECT * FROM #TempTable
What we're doing here is creating a temporary table (#TempTable
) with the desired column structure. Then, we use the INSERT INTO
statement along with the EXEC
command to execute the stored procedure and insert the result into our temporary table. Finally, we can simply select from the temporary table to get our desired output! ๐
Solution 2: Using OPENROWSET ๐ช
Another way to achieve the same result is by using the OPENROWSET
function. Here's how it works:
SELECT *
FROM OPENROWSET('SQLNCLI', 'Server=(local);Trusted_Connection=yes;',
'EXEC MyProc') AS Result
In this solution, we utilize the OPENROWSET
function, specifying the server name and trusted connection, followed by the EXEC MyProc
statement. This allows us to select the output directly, without the need for temporary tables.
Taking it Further ๐
Now that you know how to select from a stored procedure, you might want to take it even further! Maybe you want to implement paging using SELECT TOP X
, ROW_NUMBER
, and an additional WHERE
clause, without passing these values as parameters. Here's a little bonus tip for you! ๐
WITH CTE AS (
SELECT *,
ROW_NUMBER() OVER (ORDER BY SomeColumn) AS RowNum
FROM (EXEC MyProc) AS ProcResult
)
SELECT *
FROM CTE
WHERE RowNum BETWEEN 1 AND X
In the example above, we use a common table expression (CTE) to apply the ROW_NUMBER()
function to the result of the stored procedure. We then use the BETWEEN
clause in the final SELECT
statement to implement paging, selecting the desired range of rows.
Wrap Up and Engage! ๐
So there you have it! ๐ Two simple solutions to select from a stored procedure, along with a bonus tip to implement paging. We hope you found this guide helpful and easy to follow! If you have any questions, feel free to leave a comment below. Also, don't forget to share this post with your fellow developers who might find it useful! ๐ฃ๐ป
Now go ahead and put your newfound knowledge to use! Happy coding! ๐๐ฉโ๐ป
What other challenges or questions have you encountered while working with stored procedures? Share your experiences in the comments below!