How to request a random row in SQL?
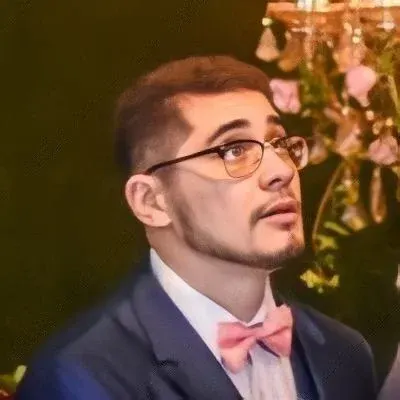
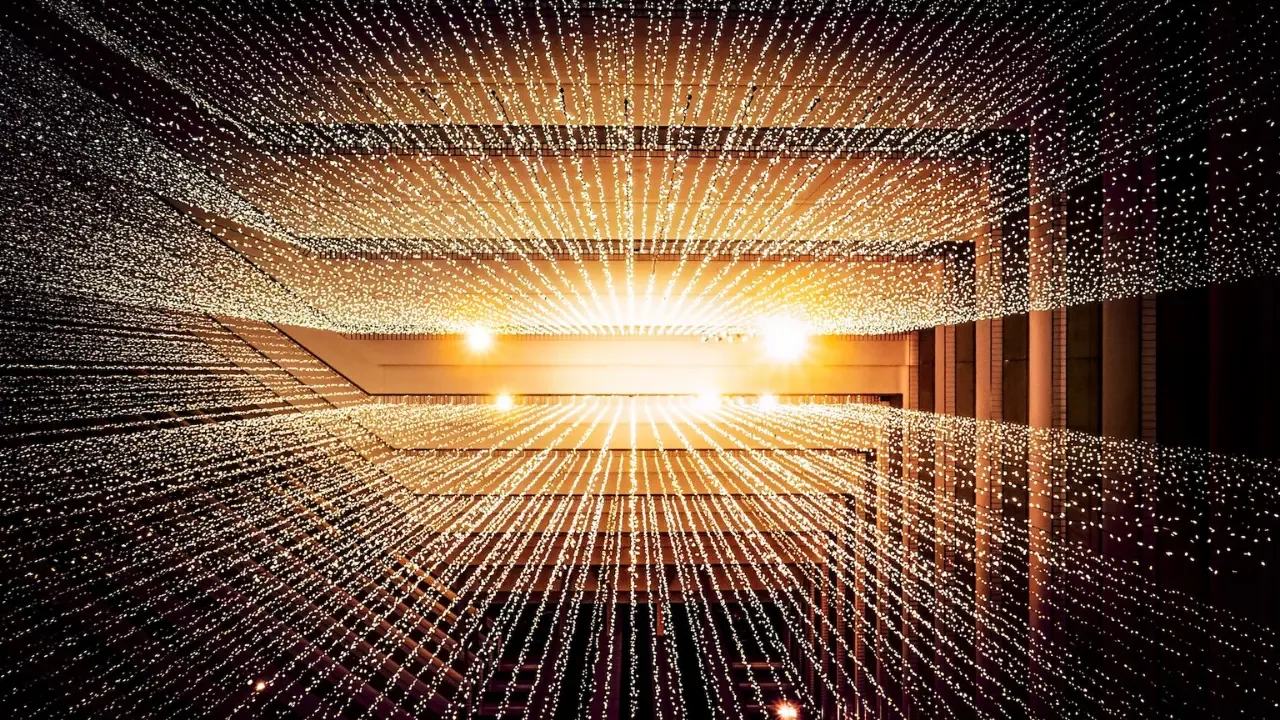
How to Request a Random Row in SQL: Unleash the Power of Chaos! ๐ฒ
Have you ever found yourself in need of a truly random row from a database? Maybe you're building a game and need to pick a random player for a special event, or perhaps you just want to keep your users entertained with some unpredictable content. Whatever the reason, fear not! In this guide, we're going to dive into the magical realm of SQL and explore different methods to request a random row. Let's bring some chaos to your SQL queries! ๐ช๏ธ
The Quest for True Randomness ๐งโโ๏ธ
Before we plunge into the depths of SQL randomness, let's address an important aspect: true randomness. Achieving true randomness within an SQL query is a challenging task. Remember, SQL is a declarative language designed to retrieve specific data based on conditions and logic. However, we can come close to true randomness by using clever tricks and techniques.
Method 1: ORDER BY with Random Function ๐ฏ
One popular method to request a random row in SQL is by using the ORDER BY
clause alongside a random function. For example, in MySQL, you can utilize the RAND()
function. Here's how you can accomplish this:
SELECT *
FROM your_table
ORDER BY RAND()
LIMIT 1;
This query fetches all rows from your table, orders them randomly using RAND()
, and then limits the result to just one row. Voila! You've got yourself a random row!
๐ก Pro Tip: Keep in mind that using ORDER BY RAND()
can be inefficient for large tables since it generates a random value for each row and then performs a sort.
Method 2: Utilizing OFFSET and COUNT ๐ฒ
Another approach to requesting a random row is by utilizing the OFFSET
and COUNT
keywords. Here's how it works:
SELECT *
FROM your_table
OFFSET FLOOR(RAND() * (SELECT COUNT(*) FROM your_table))
LIMIT 1;
In this query, we're calculating a random number between 0 and the total count of rows in your_table
. The OFFSET
keyword is then used to skip the random number of rows, and LIMIT
ensures we only receive one row. This method can be faster for large datasets compared to using ORDER BY RAND()
, so it's definitely worth considering!
Method 3: The Power of Stored Procedures ๐งช
For those seeking more control and flexibility, stored procedures can be a game-changer! By using stored procedures, you can encapsulate your SQL logic and even create reusable functions for generating random rows. Here's a simple example:
CREATE PROCEDURE getRandomRow()
BEGIN
DECLARE num_rows INT;
DECLARE random_index INT;
SELECT COUNT(*) INTO num_rows FROM your_table;
SET random_index = FLOOR(RAND() * (num_rows));
SELECT *
FROM your_table
LIMIT random_index, 1;
END;
With this stored procedure, you can conveniently call getRandomRow()
whenever you need a random row from your_table
. It provides a clean and reusable solution to fulfill your random row cravings!
The Final Roll of the Dice ๐ฒ
Now that you've learned different methods to request a random row in SQL, it's time to put your newfound knowledge into action! Experiment with these approaches and see which one suits your specific needs best.
Remember, randomness adds excitement and unpredictability to your applications. Embrace the chaos and let us know which method worked wonders for you! ๐
Got any other SQL questions or need help with a different tech topic? Feel free to reach out or leave a comment below. Keep learning and stay curious! ๐ฉโ๐ป
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
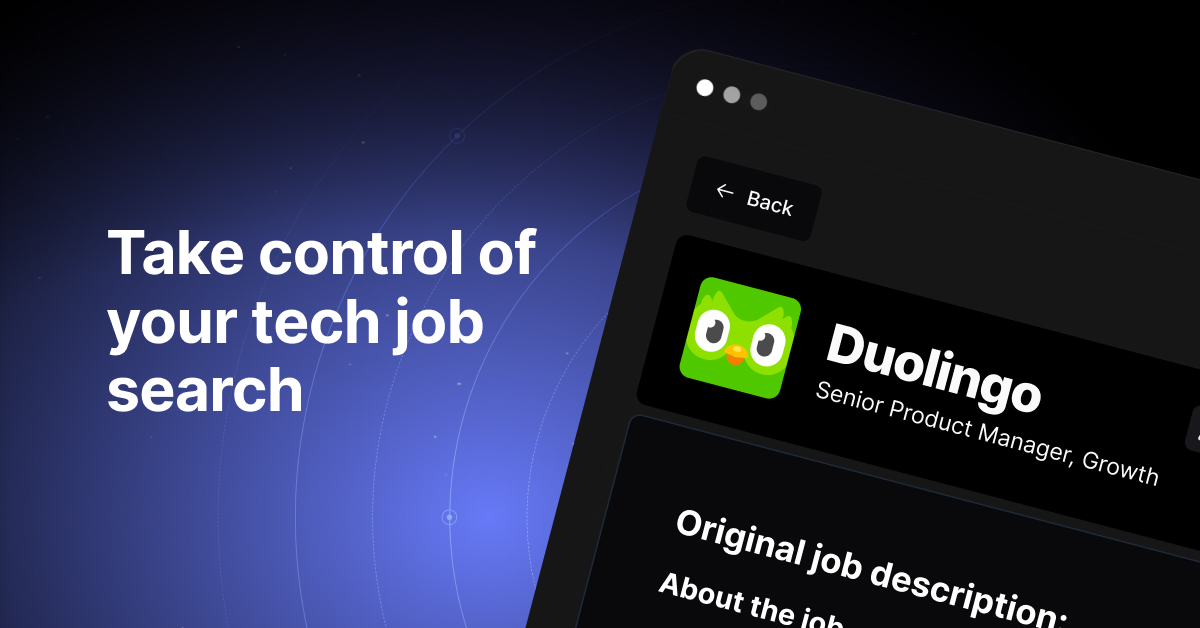