How to check if a table exists in a given schema
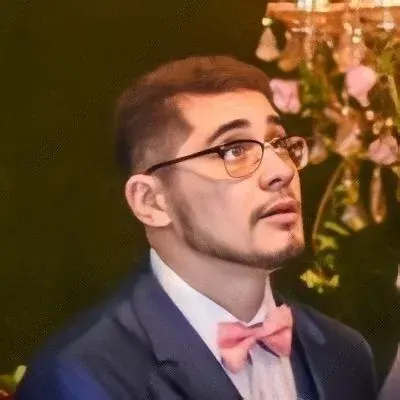
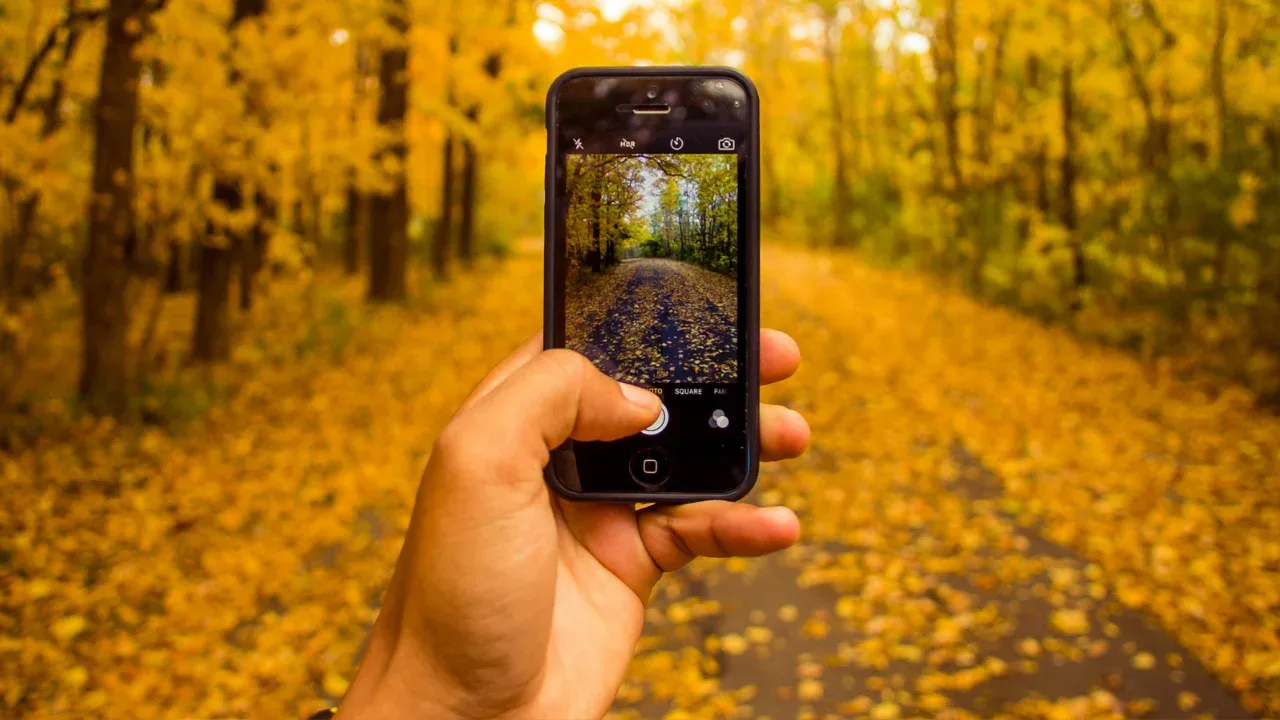
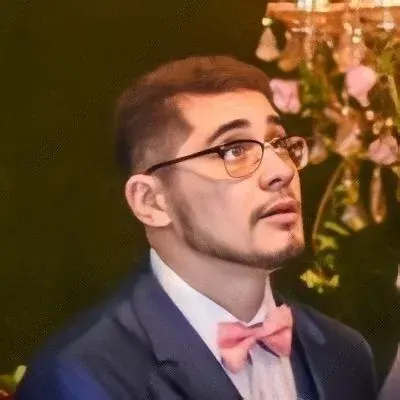
How to Check if a Table Exists in a Given Schema 📊💡
Are you working with a PostgreSQL database and need to check if a specific table exists in a particular schema?
In this blog post, we'll address this common issue and provide you with easy solutions. By the end, you'll be able to determine if a table exists, even in cases where it exists in multiple schemas. Let's get started! 🚀💻
The Context 📝🔍
In PostgreSQL 8.4 and greater databases, common tables are typically stored in the public
schema, while company-specific tables are stored in individual company
schemas. The company schemas are named in the format companyX
, where X
represents the company number.
For example, you may have schemas such as:
public
company1
company2
company3
...
Each application works with a single company, and the search_path
parameter in the connection string specifies the schema order to search for tables.
The Problem 🔍❓
Given this context, how can you check if a table exists in a specified companyn
schema?
For instance, when using the following query:
select isSpecific('company3','tablenotincompany3schema')
The expected result is false
.
And when using:
select isSpecific('company3','tableincompany3schema')
The expected result is true
.
The function should only check the specified companyn
schema and not other schemas. Additionally, if the given table exists in both the public
schema and the specified schema, the function should return true
. Finally, it should be compatible with PostgreSQL 8.4 and later versions.
Easy Solution 💡🛠️
To solve this problem, we can create a function that checks for the existence of a table in a given schema. Here's an example of how the function can be implemented:
CREATE OR REPLACE FUNCTION isSpecific(schema_name text, table_name text)
RETURNS boolean
AS $$
BEGIN
RETURN EXISTS (
SELECT 1
FROM pg_catalog.pg_tables
WHERE schemaname = schema_name
AND tablename = table_name
);
END;
$$ LANGUAGE plpgsql;
How the Function Works 🔄🛠️
The isSpecific
function takes two parameters: schema_name
and table_name
. It uses the EXISTS
keyword to determine whether a table exists in the specified schema.
Here's how the function works:
It queries the
pg_catalog.pg_tables
system catalog view.The
schemaname
column is compared with theschema_name
parameter to ensure that the table is checked only in the specified schema.The
tablename
column is compared with thetable_name
parameter.If a row is found, the function returns
true
; otherwise, it returnsfalse
.
Conclusion and Call-to-Action ✅📢
You've now learned how to check if a table exists in a given schema in PostgreSQL. By using the isSpecific
function we created, you can easily determine whether a table exists, even if it exists in multiple schemas.
Feel free to implement this function in your own PostgreSQL database and adjust it to fit your specific needs. If you have any questions or encounter any issues, don't hesitate to reach out for further assistance.
So go ahead, try out the isSpecific
function and let us know how it works for you! 😄🎉