How to avoid the "divide by zero" error in SQL?
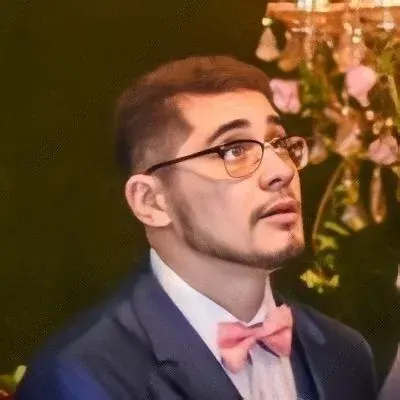
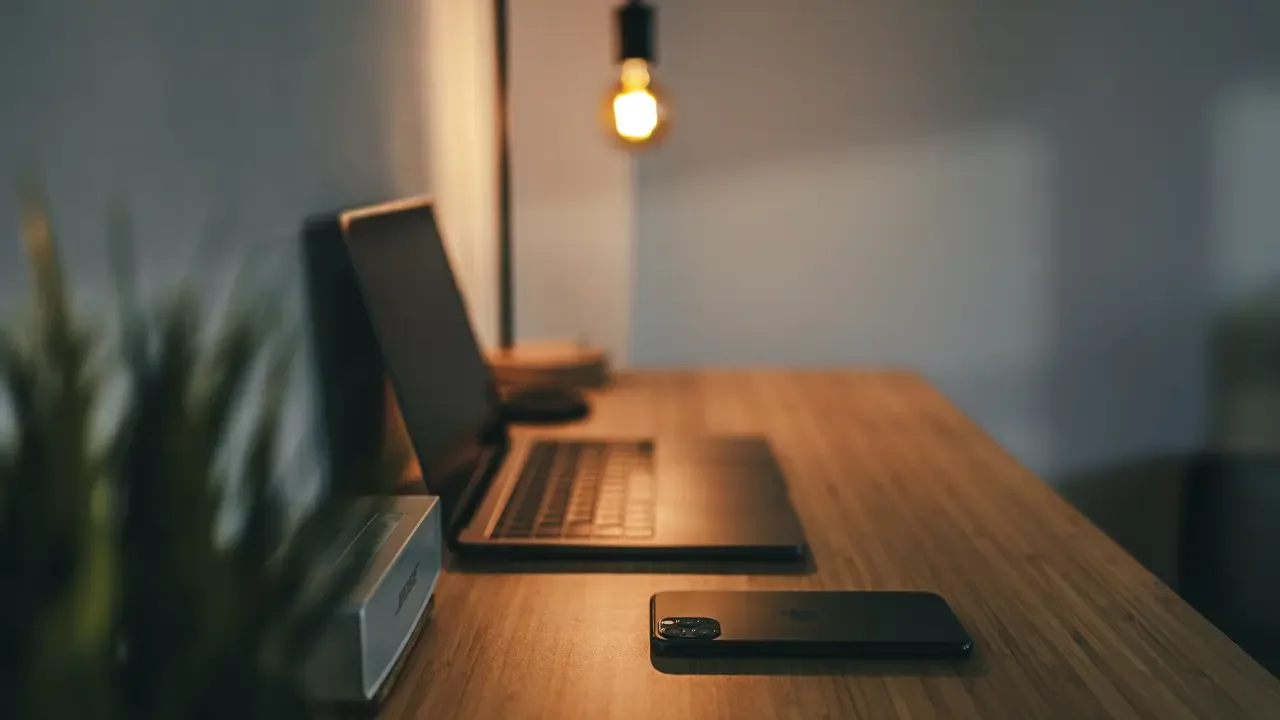
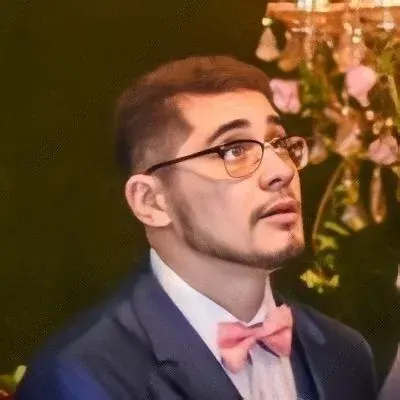
How to Avoid the "Divide by Zero" Error in SQL? 🚫➗🔢
Have you ever encountered the dreaded "Divide by zero" error while working with SQL? It can be frustrating and can cause your queries to fail. In this blog post, we will explore different ways to overcome this issue and write SQL code that ensures you never see this error message again. 💥😫
The Error Message 📝
Let's start by taking a look at the error message that is commonly encountered:
Msg 8134, Level 16, State 1, Line 1
Divide by zero error encountered.
This error occurs when you attempt to perform division in your SQL code, and the divisor (the number you're dividing by) is zero. SQL does not allow division by zero, as it results in undefined behavior.
Solution 1: Adding a WHERE Clause 🕵️♀️
One way to avoid the "Divide by zero" error is by adding a WHERE clause to your SQL code. The WHERE clause acts as a filter and ensures that your divisor is never zero. Let's take a look at an example:
SELECT column1 / column2
FROM your_table
WHERE column2 <> 0
In this example, we are dividing the values in column1
by the values in column2
. The WHERE clause column2 <> 0
ensures that only non-zero values are considered for division. This way, we prevent the error from occurring.
Solution 2: Adding a CASE Statement 🔄
Another approach is to use a CASE statement in your SQL code, which allows you to provide special treatment for zero values. Here's an example:
SELECT column1,
CASE
WHEN column2 = 0 THEN 0 -- Special treatment for divisor being zero
ELSE column1 / column2
END AS result
FROM your_table
In this example, if column2
is zero, we assign the value of 0 to the result. Otherwise, we perform the division as usual. This way, we handle the zero divisor scenario gracefully without causing an error.
Better Solution: Using the NULLIF Clause ✨
While the above solutions work, there is a better way to handle the "Divide by zero" error in SQL. The NULLIF clause comes to our rescue! 👏👍
The NULLIF clause compares two expressions and returns NULL if they are equal. We can utilize this to handle the zero divisor scenario. Take a look at the following example:
SELECT column1 / NULLIF(column2, 0) AS result
FROM your_table
In this example, we use the NULLIF clause to compare column2
with zero. If they are equal, NULL is returned. Otherwise, the division is performed as usual. This approach not only avoids the error but also provides an elegant and concise solution.
Enforcing the Solution 🛡
To enforce the usage of the above solutions and prevent the "Divide by zero" error from creeping back into your SQL code, you can follow these best practices:
Educate your team: Make sure all team members are aware of this error and the solutions mentioned in this blog post. Share this knowledge across your organization.
Code reviews: Incorporate a code review process in which code snippets involving division are thoroughly checked to ensure the error handling techniques are applied correctly.
Documentation: Update your organization's SQL coding guidelines and documentation to include the recommended solutions for handling the "Divide by zero" error.
Conclusion and Call-to-Action 🎯
By implementing any of the suggested solutions (adding a WHERE clause, using a CASE statement, or leveraging the power of the NULLIF clause), you can avoid the "Divide by zero" error in your SQL code. Remember to enforce these best practices within your organization to prevent future occurrences.
If you found this blog post helpful, share it with your friends and colleagues who work with SQL. Let's eliminate the "Divide by zero" error together! 🙌💪
Have you encountered any other frustrating SQL errors? Let us know in the comments below or reach out to us on social media. Happy coding! 😃👩💻👨💻