Get all table names of a particular database by SQL query?
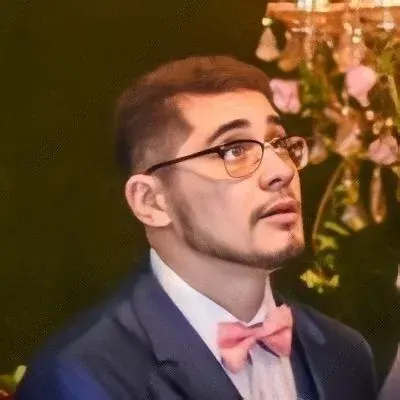
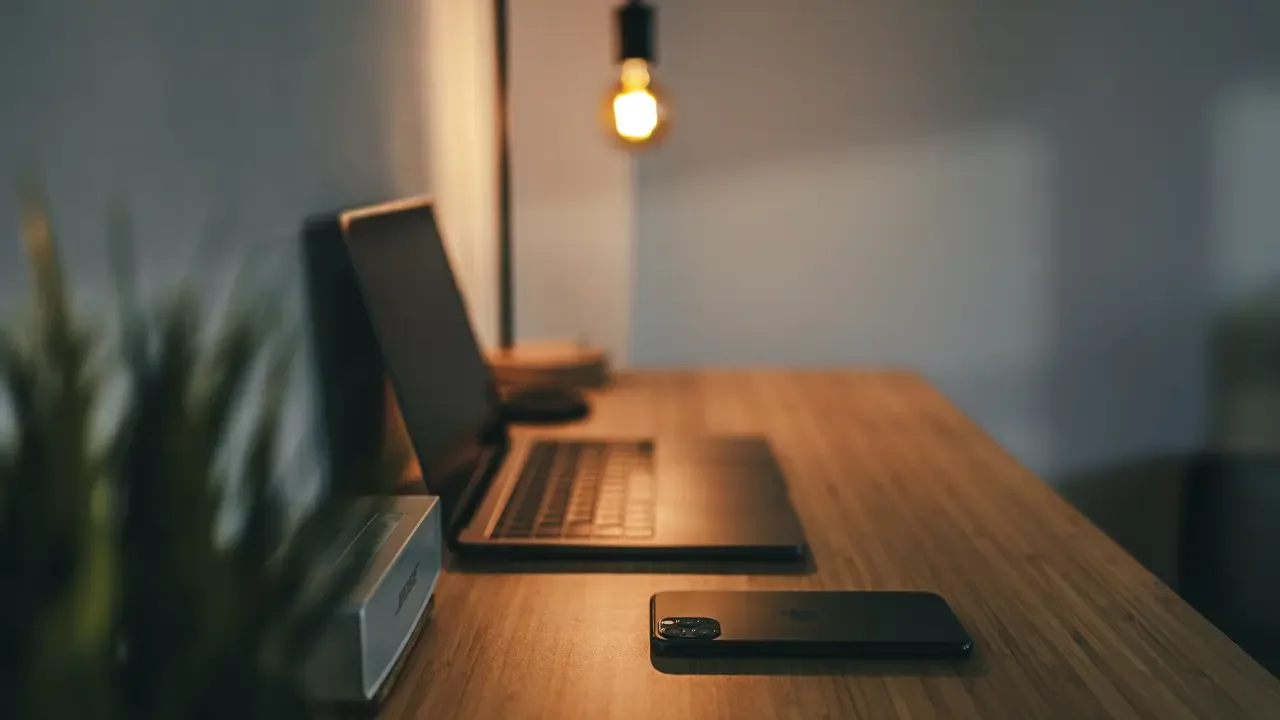
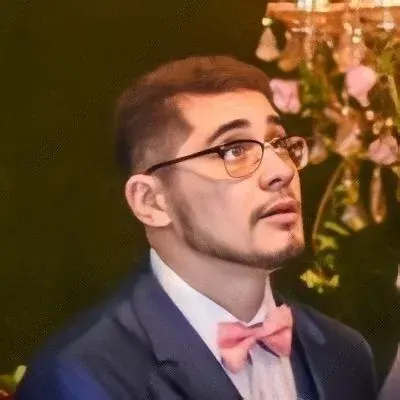
🔍 Getting All Table Names of a Specific Database Using SQL Query
Are you working on an application that deals with multiple database servers like MySQL and MS SQL Server? Do you need to find a reliable and universal SQL query to retrieve the names of tables in a certain database? Look no further! 🙌
You might have already tried using the SQL query below, which retrieves the table names of all databases on a particular server:
SELECT TABLE_NAME
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_TYPE='BASE TABLE'
However, this query fetches the table names from all databases on the server, not just the selected database. But don't worry, I'll guide you through how to modify this query to restrict it to a specific database. Let's dive in! 🌊
💡 Solution: Restricting the Query to a Specific Database
To get the table names of a particular database only, you need to add an additional condition in the WHERE clause of your query. This condition will match the desired database name against the TABLE_SCHEMA column in the INFORMATION_SCHEMA.TABLES system view. Here's an updated version of the query:
SELECT TABLE_NAME
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_TYPE='BASE TABLE'
AND TABLE_SCHEMA='<your_database_name>'
Replace <your_database_name>
with the name of the database you want to retrieve the table names from. For example, if your database is named "my_app_db," your query would be:
SELECT TABLE_NAME
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_TYPE='BASE TABLE'
AND TABLE_SCHEMA='my_app_db'
Running this query will give you a result set with the table names of the selected database only. 🎉
🔥 Take It to the Next Level: Automating the Process
Now that you have the solution to retrieve the table names of a specific database, why not take it a step further? You can create a function or a stored procedure that accepts the database name as a parameter and returns the table names programmatically. This will make your application more robust and modular.
For example, in MySQL, you can create a stored procedure like this:
DELIMITER $$
CREATE PROCEDURE GetTableNamesOfDatabase(IN database_name VARCHAR(255))
BEGIN
SELECT TABLE_NAME
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_TYPE='BASE TABLE'
AND TABLE_SCHEMA=database_name;
END$$
DELIMITER ;
With this stored procedure, you can simply call GetTableNamesOfDatabase('<your_database_name>')
, and it will return the table names for you. You can then use this stored procedure throughout your application whenever you need to retrieve table names dynamically. 💪
🙌 Share Your Experience and Get Involved!
Have you encountered any challenges while working with multiple database servers? How did you handle them? Share your experience in the comments below! Let's learn from each other and build a community of database-savvy developers. 🚀
If you found this guide helpful, don't forget to share it with your fellow developers who might benefit from it. Together, we can make database interactions a breeze! 🌟