Fast way to discover the row count of a table in PostgreSQL
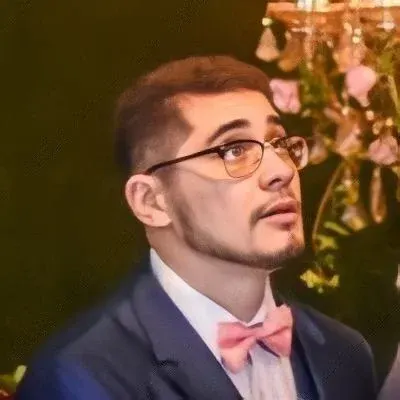
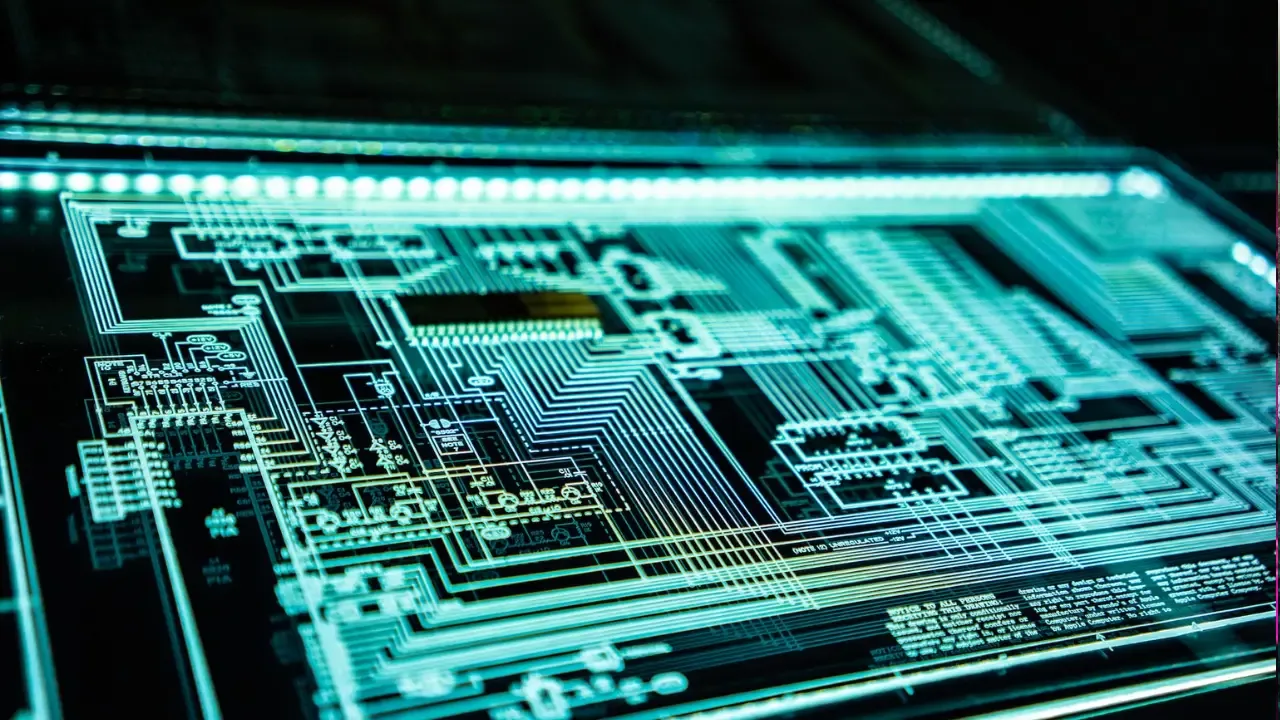
How to Quickly Discover the Row Count of a Table in PostgreSQL
:bar_chart:
Are you in desperate need of finding the row count of a table in PostgreSQL? :thinking: Don't worry, we've got you covered! In this guide, we'll walk you through a fast and efficient way to get the exact number of rows you desire, without wasting unnecessary time. :rocket:
The Problem: Counting Rows Efficiently
:thought_balloon: Let's say you need to calculate a percentage based on the number of rows in a table. However, if the total row count exceeds a pre-defined constant value, you'd rather use that constant instead of counting every single row. :hourglass_flowing_sand:
The Solution: Stop Counting When the Limit is Reached
:bulb:
Fortunately, PostgreSQL provides an elegant solution to tackle this issue. Instead of manually counting each row by executing SELECT COUNT(*) FROM table
, we can employ a more intelligent approach that stops counting as soon as the given limit is surpassed. :white_check_mark:
To achieve this, we can incorporate a user-defined function that returns the count up until the limit, and then switches to the constant value if necessary. Let's break it down step by step:
Create the user-defined function:
CREATE OR REPLACE FUNCTION count_with_limit(limit_value integer, constant_value integer)
RETURNS integer AS
$$
DECLARE
row_count integer;
BEGIN
SELECT COUNT(*) INTO row_count FROM your_table;
IF row_count <= limit_value THEN
RETURN row_count;
ELSE
RETURN constant_value;
END IF;
END;
$$
LANGUAGE plpgsql;
Use the function in your query:
SELECT text, count(*), count_with_limit(500000, 500000) AS percent_calculated
FROM your_table
GROUP BY text
ORDER BY count DESC;
:sparkles:
By incorporating the count_with_limit
function into your query, you can now obtain the exact row count below the limit, or the constant value if the count exceeds the limit. :raised_hands: This way, you save valuable time and avoid unnecessary computations.
Take It to the Next Level: Share Your Thoughts!
:tada:
Now that you've learned a fast and efficient way to discover the row count of a table in PostgreSQL, it's time to put your knowledge to the test. Try implementing this approach in your own projects and share your results with us! :chart_with_upwards_trend:
Have you encountered any other performance-related challenges in PostgreSQL? Let us know in the comments section below. We'd love to help you find a solution! :raised_hand_with_fingers_splayed:
And don't forget to share this post with your tech-savvy friends who might be struggling with similar problems. Sharing is caring! :heart:
Happy counting! :abacus:
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
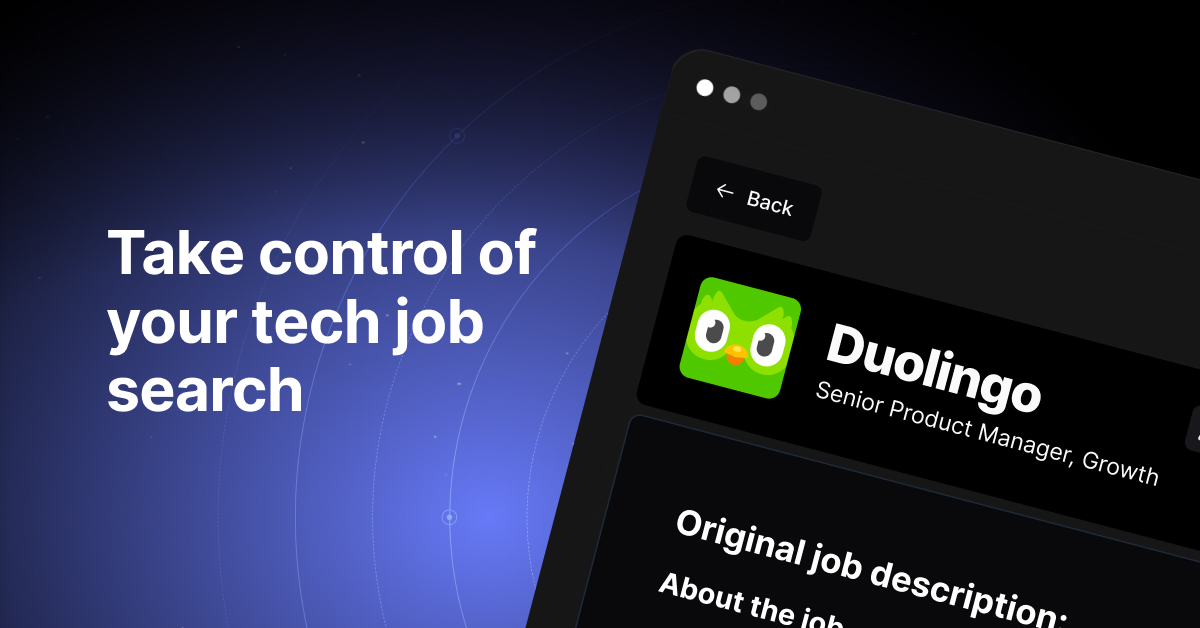