Extract date (yyyy/mm/dd) from a timestamp in PostgreSQL
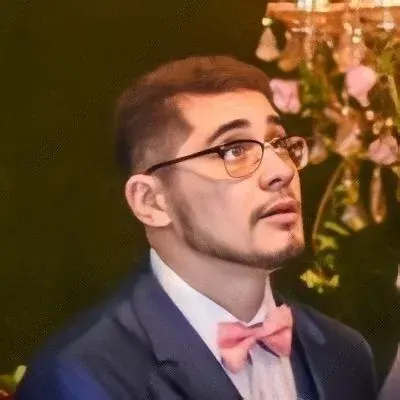
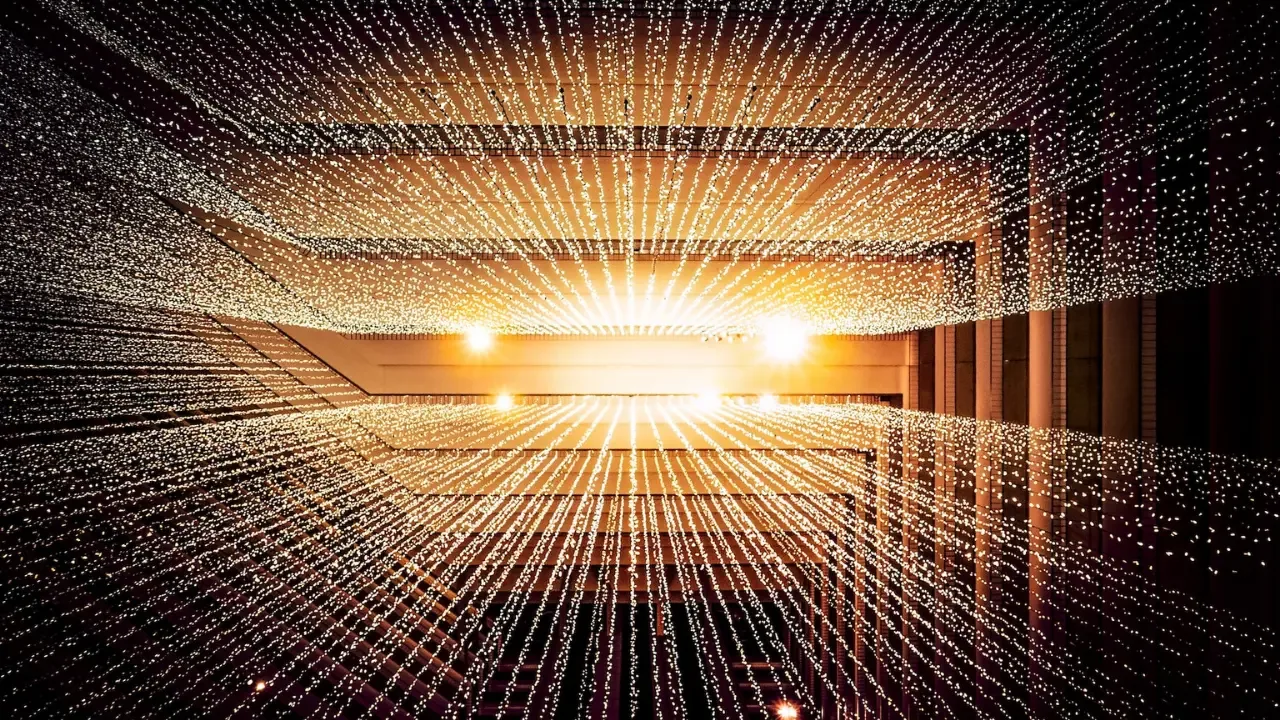
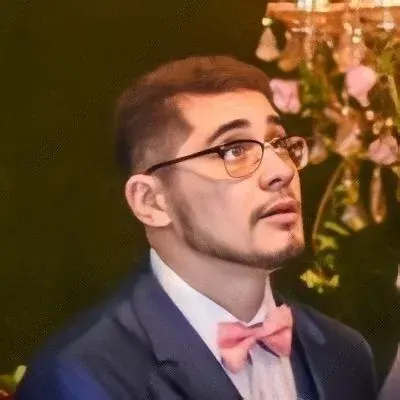
How to Extract the Date from a Timestamp in PostgreSQL
So you have a timestamp in PostgreSQL, but you only need the date part. Maybe you want to insert it into another table that expects a DATE
value. Don't worry, we've got you covered! In this guide, we'll explore the best ways to extract the date from a timestamp in PostgreSQL, along with some common issues you may encounter.
The Problem
Let's start with the problem at hand. You have a timestamp like 2011/05/26 09:00:00
, and you want to extract just the date part 2011/05/26
. Simple, right? Well, it turns out that some methods may not give you the desired result.
The Failed Attempts
Casting
One approach is to use casting to convert the timestamp to a DATE
type. However, if you try something like CAST(timestamp AS DATE)
, you might end up getting only the year, like 2011
. Not exactly what you were expecting.
Using to_char()
and to_date()
Another attempt could involve using the functions to_char()
and to_date()
. You might try something like:
SELECT to_date(to_char(timestamp, 'YYYY/MM/DD'), 'YYYY/MM/DD')
FROM yourTable WHERE id=1;
This approach seems promising, as it converts the timestamp to a string representation in the desired format (YYYY/MM/DD
) and then converts it back to a DATE
using to_date()
. However, this can still lead to unexpected results, especially with certain timestamp formats.
Creating a Function
If you're still not getting the expected output, you might think about creating a function to handle the extraction. You could try something like:
CREATE OR REPLACE FUNCTION extract_date(timestamp) RETURNS date AS $$
DECLARE i_date DATE;
BEGIN
SELECT to_date(to_char($1, 'YYYY/MM/DD'), 'YYYY/MM/DD')
INTO i_date FROM yourTable WHERE id=1;
RETURN i_date;
END;
$$ LANGUAGE plpgsql;
This function should take a timestamp as input and return the extracted date. However, be cautious when using this approach, as it can be unnecessarily complex for such a seemingly simple task.
The Best Way Forward
So, after some trial and error, what is the best way to extract the date (yyyy/mm/dd) from a timestamp in PostgreSQL? Thankfully, PostgreSQL provides a simpler solution.
Using the DATE()
Function
The DATE()
function in PostgreSQL allows you to extract the date part from a timestamp directly. You can use it like this:
SELECT DATE(timestamp) FROM yourTable WHERE id=1;
This will give you the expected output of 2011-05-26
. The result is automatically converted to the DATE
type, making it suitable for your intended use.
Conclusion
Extracting the date from a timestamp in PostgreSQL doesn't have to be complicated. By using the DATE()
function, you can easily get the desired date part without any unnecessary conversions or complex functions.
So go ahead, give it a try, and let us know how it works for you! Have any other methods that worked well for you? Feel free to share in the comments below.
Happy timestamp extraction! 🗓️