Correct use of transactions in SQL Server
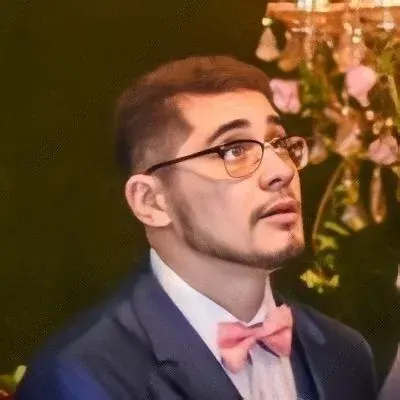
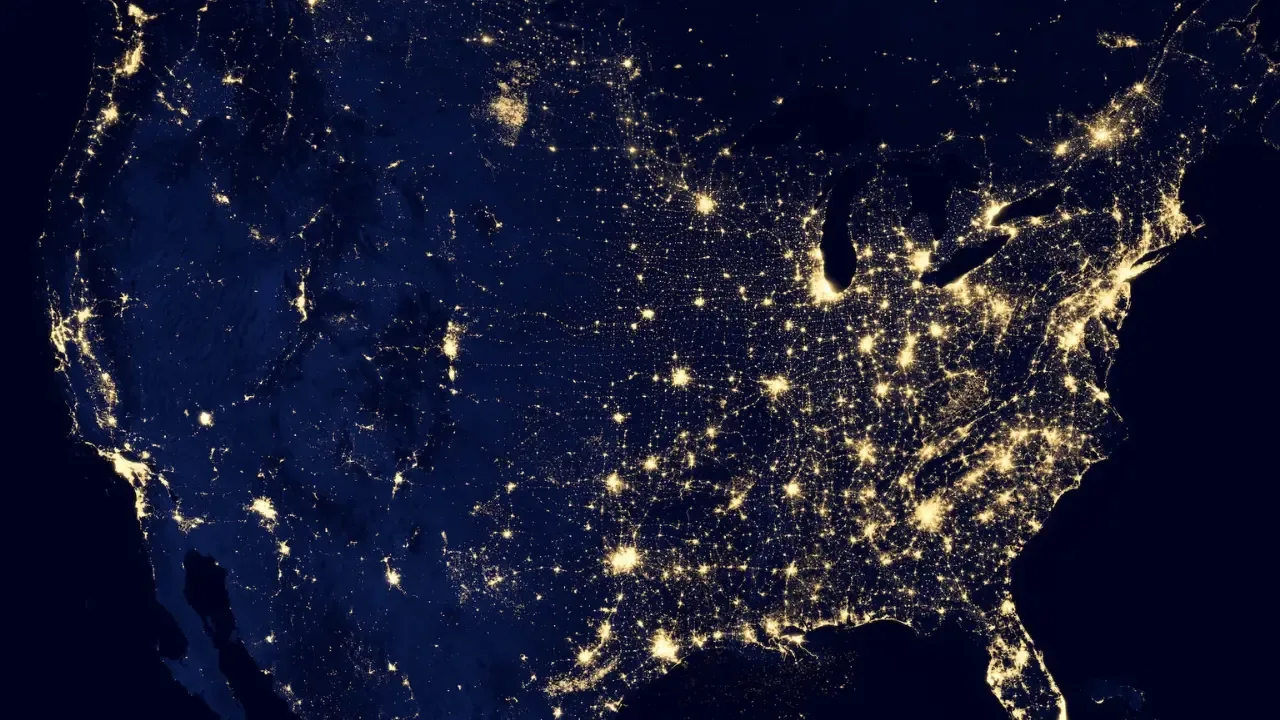
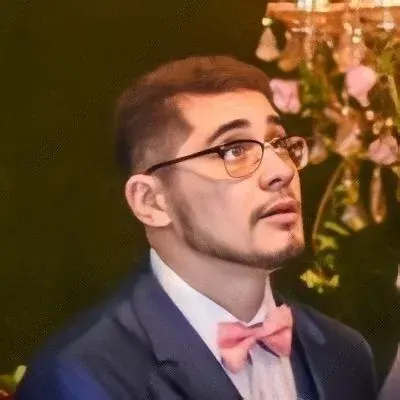
The Correct Use of Transactions in SQL Server 🔄
Transactions are a powerful tool in SQL Server to ensure data integrity and consistency. They allow you to group a series of SQL commands into a single unit of work, ensuring that either all commands succeed or none of them are executed. This can be particularly useful when you have critical operations that must be atomic - if one fails, you want to roll back all changes.
The Mistake in the Script 🚫
Let's take a look at the script that raises the question:
BEGIN TRANSACTION [Tran1]
INSERT INTO [Test].[dbo].[T1]
([Title], [AVG])
VALUES ('Tidd130', 130), ('Tidd230', 230)
UPDATE [Test].[dbo].[T1]
SET [Title] = N'az2', [AVG] = 1
WHERE [dbo].[T1].[Title] = N'az'
COMMIT TRANSACTION [Tran1]
GO
In this script, there are two commands - an INSERT
and an UPDATE
. The intention is to execute both commands correctly or none of them at all. The script begins a transaction with BEGIN TRANSACTION
, executes the commands, and then commits the transaction with COMMIT TRANSACTION
.
However, there is a problem with the UPDATE
command. It has an incorrect syntax, which causes the entire transaction to fail. And when a transaction fails, SQL Server does not automatically roll back the changes made before the failure. This can lead to inconsistent data.
Solving the Problem with Transactions 💡
To implement a transaction that rolls back all commands if any of them have an error, we need to make a small adjustment to the script. We should introduce error handling and use a TRY
...CATCH
block.
BEGIN TRY
BEGIN TRANSACTION [Tran1]
INSERT INTO [Test].[dbo].[T1]
([Title], [AVG])
VALUES ('Tidd130', 130), ('Tidd230', 230)
UPDATE [Test].[dbo].[T1]
SET [Title] = N'az2', [AVG] = 1
WHERE [dbo].[T1].[Title] = N'az'
COMMIT TRANSACTION [Tran1]
END TRY
BEGIN CATCH
IF @@TRANCOUNT > 0
ROLLBACK TRANSACTION [Tran1]
END CATCH
By enclosing the commands within a TRY
block and adding a CATCH
block, we can handle any errors that occur. If an error occurs, the control is transferred to the CATCH
block, where we check if there is an active transaction (@@TRANCOUNT > 0
). If there is, we roll back the transaction using ROLLBACK TRANSACTION
.
Now, if any of the commands encounter an error, the entire transaction will be rolled back, ensuring data integrity.
Your Turn: Start Using Transactions Effectively ✅
Transactions are a vital concept in SQL Server, and understanding how to use them correctly can save you from data inconsistencies. Take a moment to review your codebase and identify areas where transactions could be implemented to guarantee atomicity.
Have you encountered any issues or challenges with transactions before? Share your stories and insights in the comments below! Let's learn from each other and build a robust SQL Server community. 😊📚
🙌 Join the SQL Server Mastery Club
Interested in learning more about SQL Server and mastering its powerful features? Join our exclusive SQL Server Mastery Club! We provide weekly tips, tricks, and tutorials to help you become a SQL Server ninja. Don't miss out - sign up now and level up your SQL skills! 🎓🔥
Remember, transactions are your allies when it comes to maintaining data integrity. Implement them wisely, and your database will thank you! 💪💾