With Spring can I make an optional path variable?
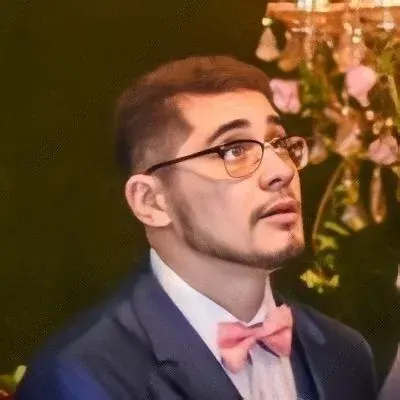
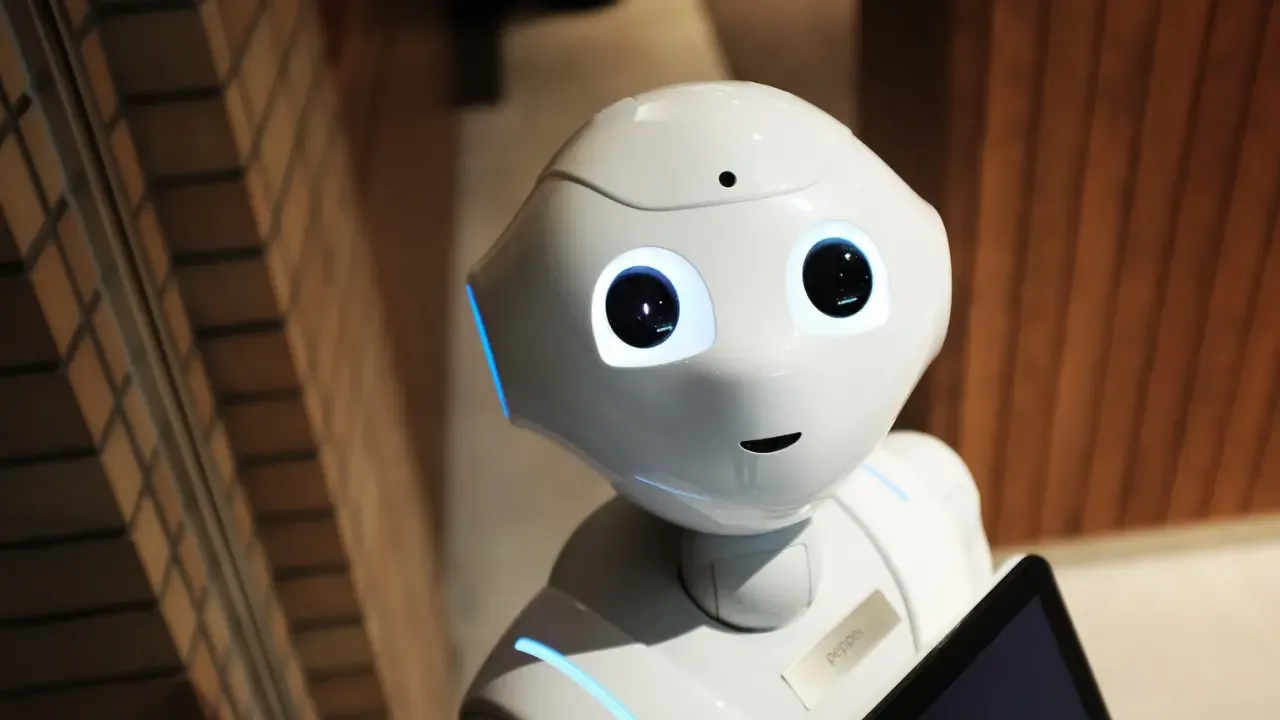
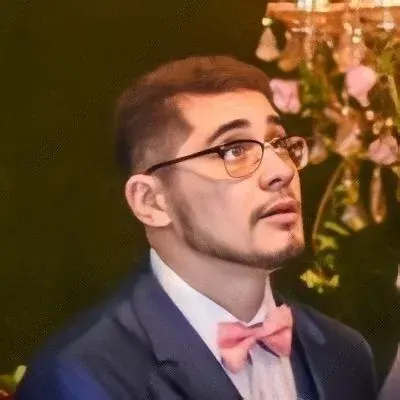
🌸 Spring and Optional Path Variables: A Quick Guide 🌸
Do you find yourself asking, "Can I have an optional path variable with Spring 3.0?" 🤔 Look no further! We've got you covered with easy solutions to this common issue. Let's dive in and explore some alternatives!
🌱 The Problem
Consider the following code snippet:
@RequestMapping(value = "/json/{type}", method = RequestMethod.GET)
public @ResponseBody TestBean testAjax(
HttpServletRequest req,
@PathVariable String type,
@RequestParam("track") String track) {
return new TestBean();
}
In this example, we would like both /json/abc
and /json
to call the same method. However, Spring treats path variables as mandatory by default 🚫, so it expects a value for {type}
. How can we make it optional? Let's find out!
✨ The Workaround
One way to make the path variable optional is to declare it as a request parameter instead:
@RequestMapping(value = "/json", method = RequestMethod.GET)
public @ResponseBody TestBean testAjax(
HttpServletRequest req,
@RequestParam(value = "type", required = false) String type,
@RequestParam("track") String track) {
return new TestBean();
}
In this second example, we replaced @PathVariable
with @RequestParam(value = "type", required = false)
. Now, we can simply pass type
as a query parameter with the /json
endpoint like this: /json?type=abc&track=aa
.
🎉 The Solution
By utilizing this workaround, we ensure that both /json/abc
and /json
will call the same method. If you use the /json
endpoint without specifying the type
query parameter, no worries! Spring handles it gracefully. 🎊
So, if you're facing a similar situation where you need an optional path variable, make use of @RequestParam
instead of @PathVariable
. You'll be able to achieve the desired functionality without any hassle. 🙌
📣 Engage with Us!
We hope this guide helped you solve your Spring path variable woes! If you have any questions, or if you know of any other clever workarounds, share your thoughts and experiences in the comments below. Let's learn and grow together! 👯♀️
And don't forget to share this post with your fellow developers who might be struggling with optional path variables in Spring. Spread the knowledge! 🌟
Happy coding! 💻💡