What is the difference between ApplicationContext and WebApplicationContext in Spring MVC?
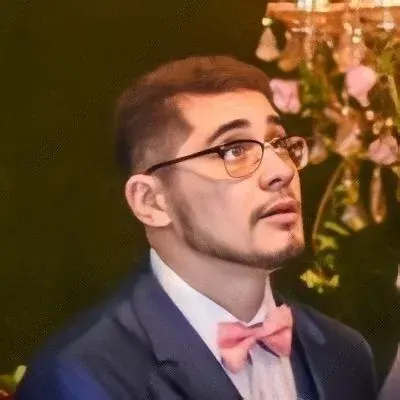
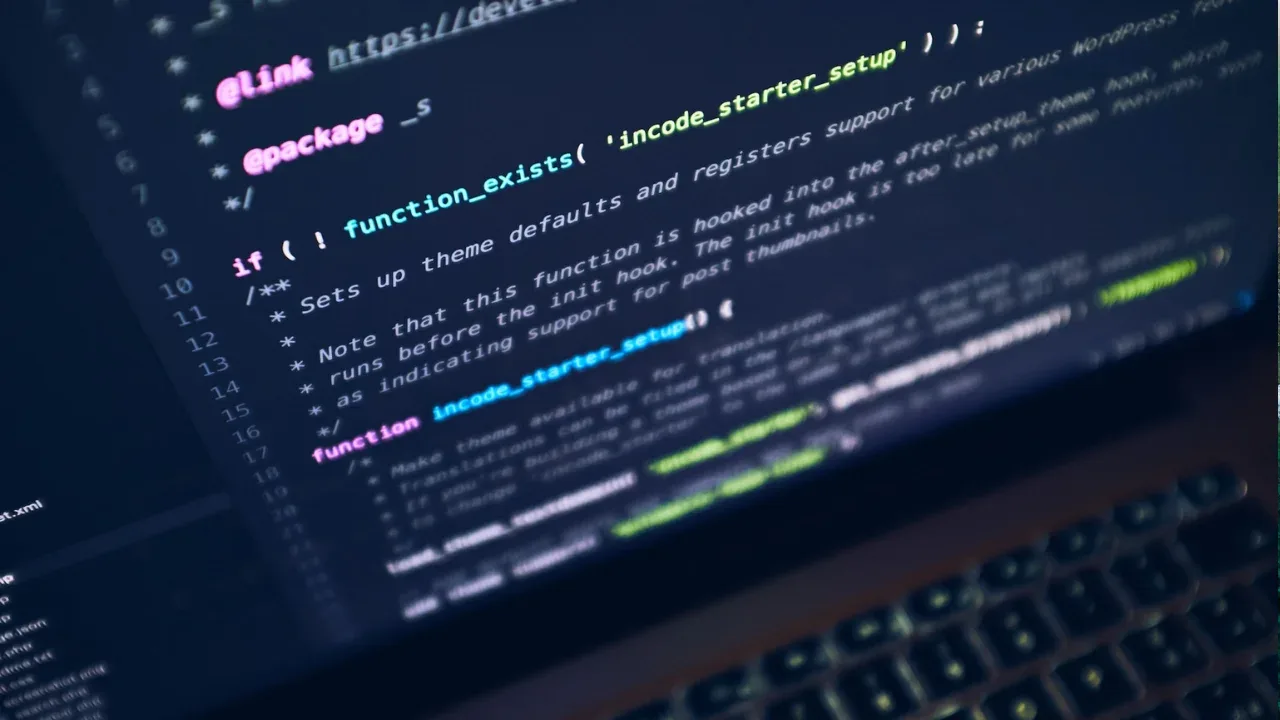
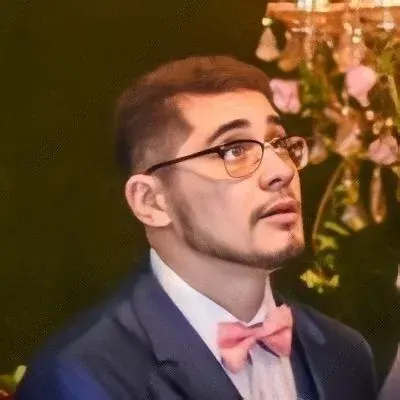
π± What is the difference between ApplicationContext and WebApplicationContext in Spring MVC? π±
Spring MVC is a powerful framework for building Java web applications. It provides a modular and flexible approach to developing web applications, making it easier to manage and scale your projects. One common question that often comes up is the difference between ApplicationContext and WebApplicationContext in Spring MVC. π€
π Let's dive in and explore the key differences and use cases for each:
1οΈβ£ ApplicationContext: The ApplicationContext is the heart of the Spring framework. It is the container that holds and manages all the beans in your application. These beans are the building blocks of your application and provide the necessary functionality. The ApplicationContext is not specifically tied to web applications and can be used in any Java application, including standalone applications. π
β‘οΈ Use case: The ApplicationContext is typically used for managing non-web components and services in your application. For example, you might use it to manage database connections, transaction handling, or any other application-specific services.
2οΈβ£ WebApplicationContext: The WebApplicationContext is an extension of the ApplicationContext specifically designed for web applications. It inherits all the features and functionality of the ApplicationContext and adds additional web-related features. It is aware of the web-specific resources and configurations. πΈοΈ
β‘οΈ Use case: The WebApplicationContext is primarily used in Spring MVC applications where web-specific components like controllers, view resolvers, and other related beans need to be managed. It provides additional features like handling of web requests, session management, and other web-related services.
π The key distinction between ApplicationContext and WebApplicationContext lies in their focus areas: ApplicationContext manages general-purpose application beans, while WebApplicationContext manages web-specific beans. π
π§° Now that we understand the difference, let's see some practical examples of bean definitions in each context:
1οΈβ£ ApplicationContext example:
<context:component-scan base-package="com.example.services" />
<bean id="userService" class="com.example.services.UserService" />
<bean id="emailService" class="com.example.services.EmailService" />
In the ApplicationContext, we can define beans for various services like UserService and EmailService, which are not specific to web applications but provide general functionality.
2οΈβ£ WebApplicationContext example:
<context:component-scan base-package="com.example.controllers" />
<mvc:annotation-driven />
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
In the WebApplicationContext, we define beans for web components like controllers and view resolvers. We can also enable features like annotation-driven MVC, which simplifies the development of web controllers.
β‘οΈ In a nutshell: ApplicationContext is for managing general-purpose application beans, while WebApplicationContext is an extension of ApplicationContext specifically designed for web applications. β‘οΈ
π‘ Quick Tips:
When building a Spring MVC application, always use WebApplicationContext for managing web-specific components.
Use ApplicationContext for creating and managing non-web components and services in your application.
π Now that you have a clear understanding of the difference between ApplicationContext and WebApplicationContext, go ahead and enhance your Spring MVC applications with the right context! π
π€ We hope this blog post has helped clarify this common Spring MVC question for you. If you have any further queries or want to share your thoughts, please leave a comment below. Let's engage in a meaningful discussion! π