What is Dependency Injection and Inversion of Control in Spring Framework?
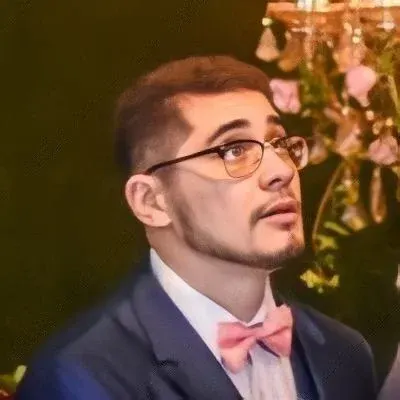
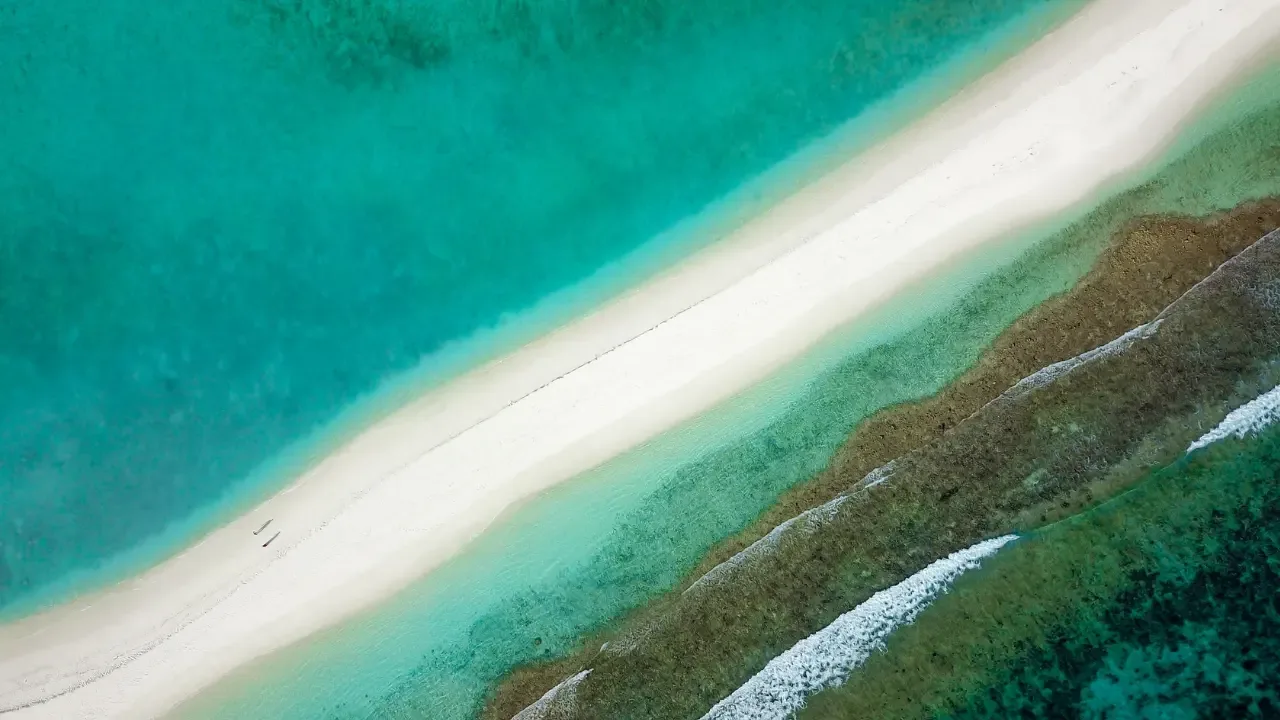
✨ Demystifying Dependency Injection and Inversion of Control in Spring Framework ✨
So, you've heard about "Dependency Injection" and "Inversion of Control" (IoC) in the Spring Framework, and you're wondering what they are all about? Don't worry, my friend, I've got you covered! 👍
🧐 Understanding the Basics
Let's start with the basics. In a nutshell, Dependency Injection (DI) is a design pattern that allows us to remove the responsibility of creating and managing objects from our code. Instead, it's handed over to a separate entity called the dependency injector.
On the other hand, Inversion of Control (IoC) is a broader concept that refers to the overall principle of inverting control within a system. In simple terms, instead of your code controlling the flow and creation of objects, the framework takes control and handles the wiring of dependencies between objects.
🤔 So, How Does it Work?
Imagine you're building a house 🏠. You need various components, such as doors, windows, plumbing, and electrical systems. Typically, you would manually assemble all these pieces yourself.
But with DI and IoC, it's like having a magical contractor who knows all the details of your house and can automatically install the components for you! 🪄
In the context of the Spring Framework, the magical contractor is the Spring IoC Container. It knows the dependencies your code needs and manages their creation and injection into your objects.
🌿 Practical Example
To make things crystal clear, let's dive into a practical example. Consider a simple Java class called Car
:
public class Car {
private Engine engine;
public Car() {
this.engine = new Engine(); // Creating the Engine object manually
}
// ...other methods
}
In the traditional approach, creating an instance of Car
means manually creating an Engine
object within its constructor.
However, with DI and IoC, we can let the Spring Framework take care of the object creation and supply the Engine
object for us! Here's how it would look:
public class Car {
private Engine engine;
public Car(Engine engine) {
this. engine = engine; // Engine object is injected through constructor
}
// ...other methods
}
By adding the Engine
parameter to the constructor, the dependency injector will automatically provide an instance of Engine
to the Car
class, ensuring seamless integration.
💡 Benefits of DI and IoC in Spring
Now that you understand the basics, let's look at some of the benefits of using Dependency Injection and Inversion of Control in the Spring Framework:
✅ Modularity: Objects are loosely coupled, making it easier to replace or update individual components without impacting the entire system.
✅ Testability: With dependencies injected, it becomes easier to mock and test components in isolation, improving overall code quality.
✅ Reusability: DI promotes reusable components by abstracting away dependencies, allowing them to be easily reused in different parts of the application.
📢 Take Action!
Are you ready to embrace the power of Dependency Injection and Inversion of Control in your Spring projects? Start by refactoring your code to rely on the Spring IoC Container for managing your dependencies.
Don't be afraid to explore more advanced features of Spring DI, such as autowiring and qualifiers, to further simplify your development process.
And, of course, don't forget to share your experiences and thoughts in the comments section below! I'd love to hear your success stories or help you with any questions you may have. 💬
Now go forth, my fellow developers, and elevate your Spring Framework game with the magic of DI and IoC! ✨🚀
Thank you for reading! If you found this blog post helpful, be sure to share it with your fellow developers. And don't forget to subscribe to our newsletter for more tech tips and tricks! 💻💡
Reference
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
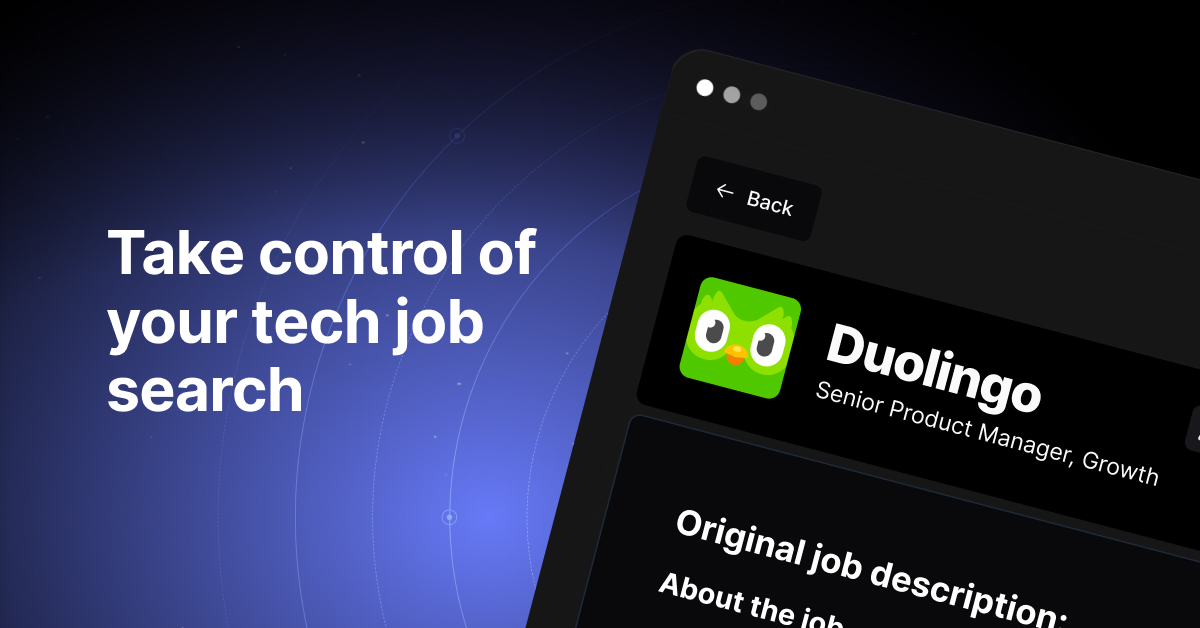