Using Spring MVC Test to unit test multipart POST request
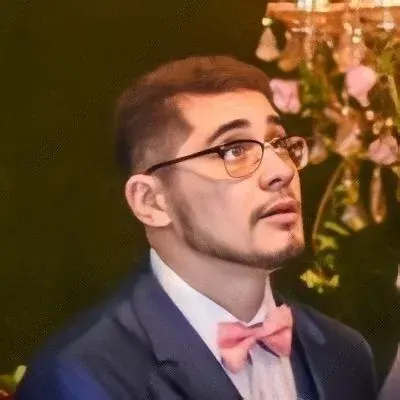
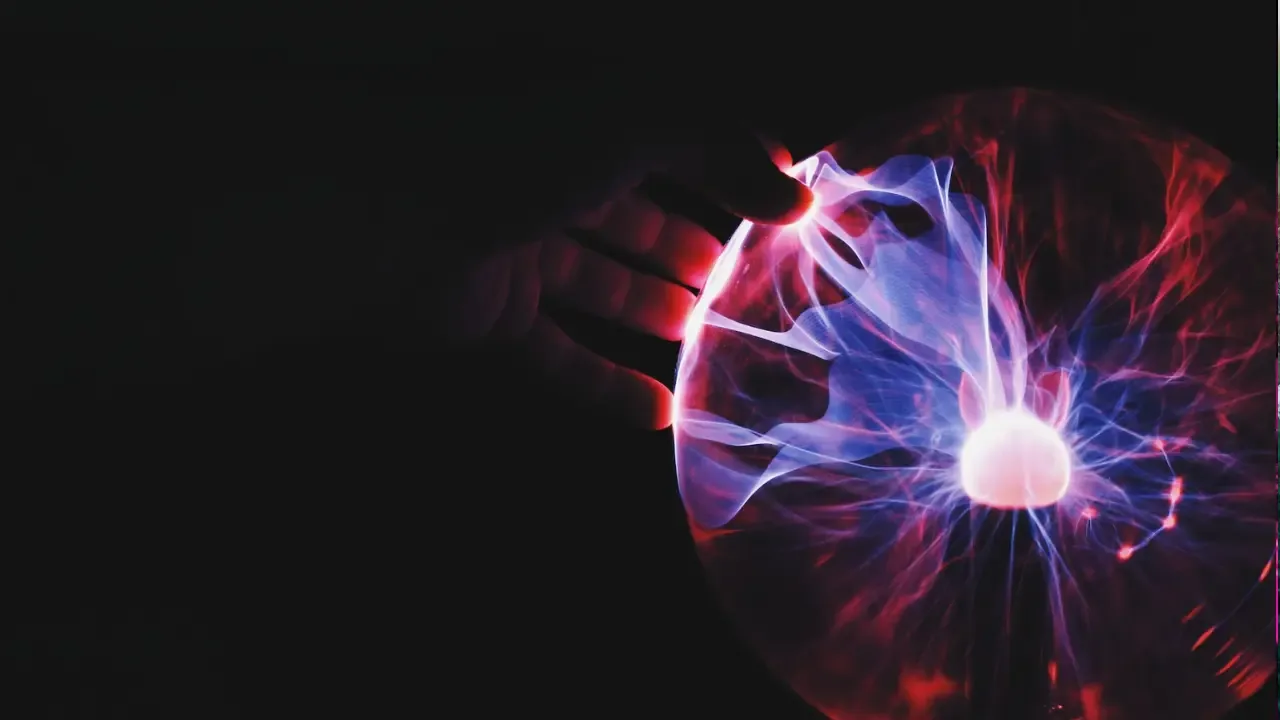
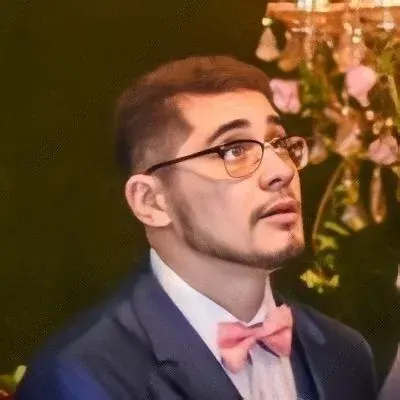
🚀 Unit Testing Multipart POST Requests with Spring MVC Test 🚀
So, you've got this awesome Spring MVC application and you want to write some unit tests for your multipart POST requests. You're in the right place! In this blog post, we'll walk you through a step-by-step guide on how to unit test your saveAuto()
method using Spring MVC Test. Let's dive in! 💪
The Challenge
First, let's understand the problem you're facing. You have a request handler for saving autos, where you accept a JSON representation of your auto along with one or more file uploads. You want to unit test this method, but you're struggling to figure out how to make it work with Spring MVC Test.
The Solution
No worries! We've got you covered. Here's how you can unit test your saveAuto()
method with Spring MVC Test:
Add Dependencies: Make sure you have the necessary dependencies in your project's
pom.xml
orbuild.gradle
file. You'll needspring-boot-starter-test
, which already includesspring-boot-starter-web
andspring-boot-starter-tomcat
.Create Test Class: Create a new test class for your
saveAuto()
method, let's call itAutoControllerTest
. Annotate it with@RunWith(SpringRunner.class)
and@WebMvcTest
.Mock Dependencies: Since we are only testing the controller layer, you can mock any necessary dependencies using
@MockBean
annotation. This will allow you to focus on testing your actual logic.Mock File Upload: Use the
MockMultipartFile
class to create a mockMultipartFile
object. This allows you to simulate file uploads during testing. You can create multiple mock files if needed.Perform the Request: Use the
MockMvc
instance to perform the request. Create aMockHttpServletRequestBuilder
and set the necessary request parts using thecontent()
method. Add the JSON representation of your auto as one part, and the mock file(s) as another part.Assert the Response: Use the
andExpect()
method to assert the response. You can check the status code, headers, and even the response body if needed.
That's it! 🎉 You have successfully unit tested your saveAuto()
method with Spring MVC Test.
Example Code
Let's put everything together with some example code:
@RunWith(SpringRunner.class)
@WebMvcTest(AutoController.class)
public class AutoControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private AutoService autoService;
@Test
public void testSaveAuto() throws Exception {
// Create mock file
MockMultipartFile mockFile = new MockMultipartFile(
"files[]",
"file1.txt",
"text/plain",
"Hello, World!".getBytes()
);
// Perform the request
mockMvc.perform(
multipart("/autos")
.file(mockFile)
.param("data", "{\"make\":\"Tesla\",\"model\":\"Model S\"}")
)
.andExpect(status().isOk())
.andExpect(content().string("Success"));
}
}
In this example, we mock the AutoService
dependency, create a mock file using MockMultipartFile
, and perform the request with the necessary request parts using .file()
and .param()
methods.
Call-to-Action
Now that you know how to unit test your multipart POST requests with Spring MVC Test, it's time to give it a try! Feel free to experiment, add more test cases, and see how your controller behaves under different scenarios. Leave a comment below to let us know how it went, or if you have any questions or suggestions.
Happy testing! 😃🔬
🔥 Don't forget to claim the 100 in bounty for the correct answer! 🔥