Spring Test & Security: How to mock authentication?
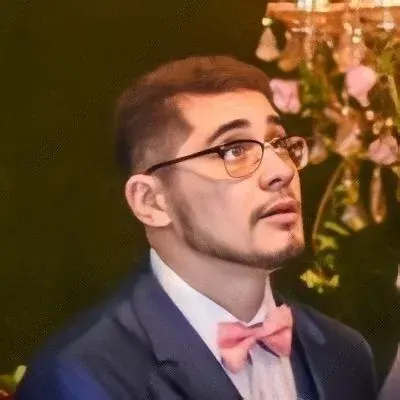
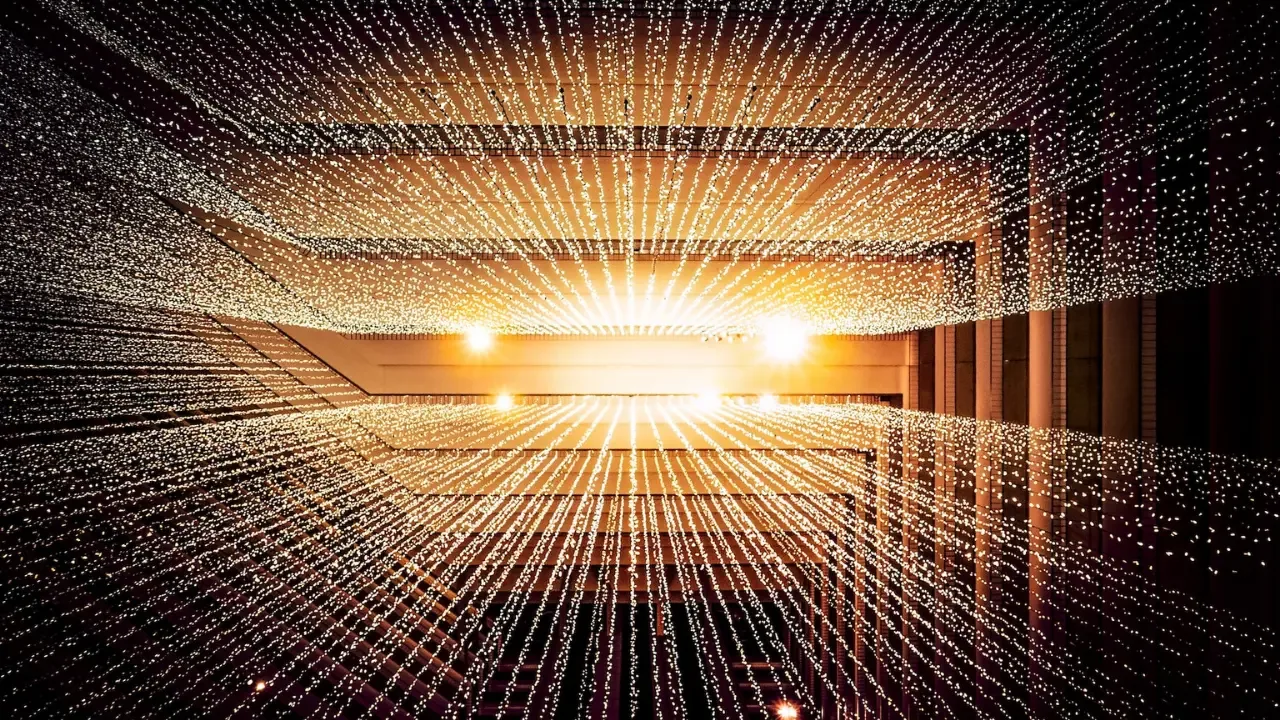
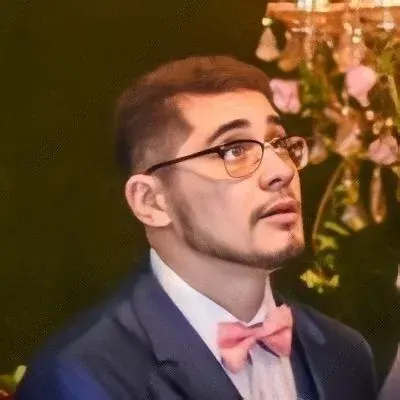
๐ How to Mock Authentication in Spring Test & Security
Are you struggling with unit testing the security of your Spring controllers? Do you want to make sure that your URLs are properly secured and that no one accidentally removes security settings? Well, you're in luck! In this blog post, we'll discuss how to mock authentication in your Spring tests to ensure that your controllers are secure. Let's dive in!
๐ค The Problem
Let's say you have a controller method like this:
@RequestMapping("/api/v1/resource/test")
@Secured("ROLE_USER")
public @ResonseBody String test() {
return "test";
}
And you want to test if the URL /api/v1/resource/test
is properly secured. You want to make sure that only users with the ROLE_USER
role can access this resource. But how do you do that in your unit tests?
๐ก The Solution
To mock authentication in your Spring tests, you can use the MockMvc
framework and the SecurityContextHolder
. Here's how you can set it up:
// Set up your test environment
@RunWith(SpringJUnit4ClassRunner.class)
@WebAppConfiguration
@ContextConfiguration({
"file:src/main/webapp/WEB-INF/spring/security.xml",
"file:src/main/webapp/WEB-INF/spring/applicationContext.xml",
"file:src/main/webapp/WEB-INF/spring/servlet-context.xml" })
public class WebappTestEnvironment {
// Set up your dependencies
@Resource
private FilterChainProxy springSecurityFilterChain;
@Autowired
@Qualifier("databaseUserService")
protected UserDetailsService userDetailsService;
@Autowired
private WebApplicationContext wac;
@Autowired
protected DataSource dataSource;
protected MockMvc mockMvc;
// Helper method to create a mock authentication token
protected UsernamePasswordAuthenticationToken getPrincipal(String username) {
UserDetails user = this.userDetailsService.loadUserByUsername(username);
return new UsernamePasswordAuthenticationToken(
user,
user.getPassword(),
user.getAuthorities()
);
}
// Set up your mock MVC
@Before
public void setupMockMvc() throws NamingException {
this.mockMvc = MockMvcBuilders
.webAppContextSetup(this.wac)
.addFilters(this.springSecurityFilterChain)
.build();
}
}
Now, in your actual test method, you can mock the authentication and perform the request like this:
public class MyControllerTest extends WebappTestEnvironment {
@Test
public void testAccess() throws Exception {
// Mock the authentication token
UsernamePasswordAuthenticationToken principal = this.getPrincipal("testUser");
SecurityContextHolder.getContext().setAuthentication(principal);
// Perform the request
super.mockMvc
.perform(
get("/api/v1/resource/test")
)
.andExpect(status().isOk());
}
}
By setting the authentication token in the SecurityContextHolder
, you can simulate the authentication of a specific user for your test. This way, you can ensure that only authorized users can access your secured resources.
๐ช Take it to the Next Level
Now that you know how to mock authentication in your Spring tests, why stop there? Extend your tests to cover more scenarios, such as testing access for different user roles or testing unauthorized access. The possibilities are endless!
๐ Conclusion
Unit testing the security of your Spring controllers can be challenging, but with the right tools and techniques, it becomes much easier. By mocking authentication in your tests, you can ensure that your URLs are properly secured and that security settings are not accidentally removed.
So go ahead and start mocking! ๐งช And remember, keep testing, keep securing, and keep rocking! ๐ค
Have you ever struggled with mocking authentication in your Spring tests? How did you overcome it? Share your experiences in the comments below and let's discuss! ๐