Spring Scheduled Task running in clustered environment
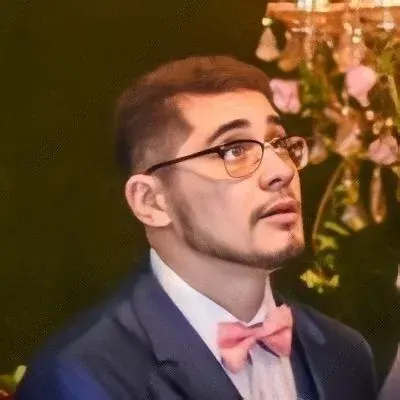
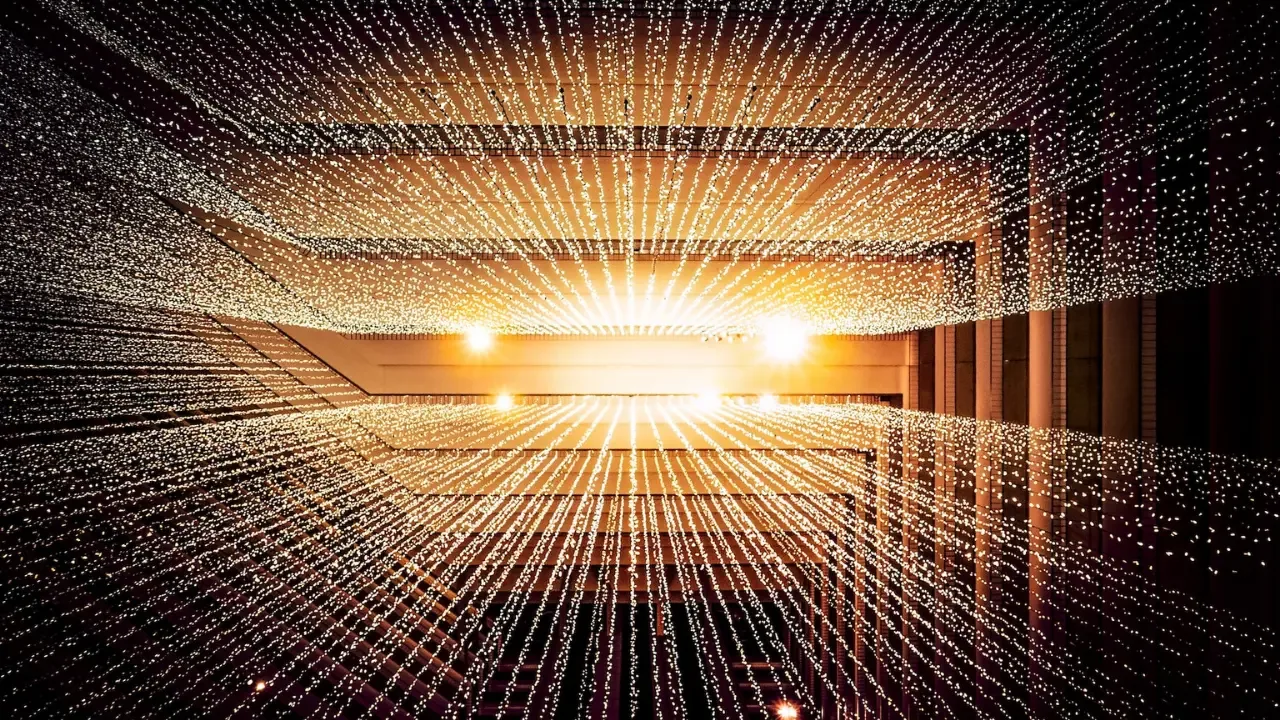
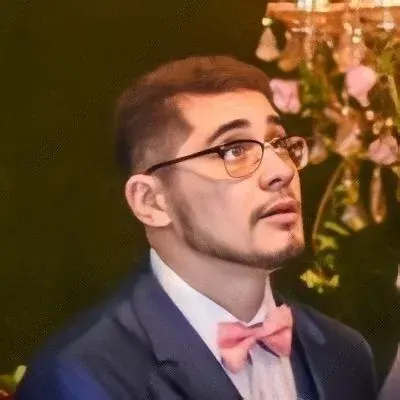
📢 Hey there tech enthusiasts! Welcome to my blog post where we'll dive into the exciting world of Spring Scheduled Tasks in a clustered environment! 😎
So, here's the situation: you're working on an application that needs to scale onto multiple instances as required. You have a cron job that needs to run every 60 seconds, but you want it to run on only one instance at a time (any node will do). 🔄
Now, you might be surprised to find out that out-of-the-box, Spring doesn't provide a direct solution for this particular scenario. But worry not, my friend, because I've got some easy solutions to get you out of this pickle! Let's get started. ⚙️
Solution 1: Implement a Distributed Lock Mechanism
One approach you can take is to implement a distributed lock mechanism. This involves leveraging technologies like ZooKeeper, Redis, or Hazelcast to coordinate among the different instances and ensure that only one instance executes the scheduled task at a time. 🗝️
Here's a snippet to help you get started using ZooKeeper as your distributed lock:
import org.apache.curator.framework.CuratorFramework;
import org.apache.curator.framework.recipes.locks.InterProcessMutex;
// ...
@Autowired
private CuratorFramework curatorFramework;
@Scheduled(cron = "0/60 * * * * *")
public void executeScheduledTask() throws Exception {
InterProcessMutex lock = new InterProcessMutex(curatorFramework, "/my-lock");
try {
if (lock.acquire(1, TimeUnit.SECONDS)) {
// Your task execution logic goes here
}
} finally {
lock.release();
}
}
Solution 2: Leverage Spring Integration
Another option you can explore is using Spring Integration. With Spring Integration, you can easily create a message-driven architecture where multiple instances can listen to a specific channel, but only one instance will receive the message and execute the task. 📨
Here's an example configuration using Spring Integration:
<int:channel id="scheduledTaskChannel" />
<int:service-activator input-channel="scheduledTaskChannel" ref="beanName" method="execute" />
<task:scheduled-tasks>
<task:scheduled ref="scheduledTaskGateway" method="trigger" cron="0/60 * * * * *" />
</task:scheduled-tasks>
@Component
public class ScheduledTaskGateway {
@Autowired
private MessageChannel scheduledTaskChannel;
public void trigger() {
scheduledTaskChannel.send(MessageBuilder.withPayload("execute").build());
}
}
Whew! 😅 Those were some handy solutions to tackle your Spring Scheduled Task problem in a clustered environment. I hope you found them useful! But hey, don't leave just yet - I've got a surprise for you.
🚀 Time for Action: Let's Engage! 🚀
Now that you're armed with these solutions, it's time to put them into practice! Give them a try and let me know how they work for you. Have a better solution in mind? I'm all ears! Share your thoughts in the comments below and let's start a tech discussion. 💬
Remember, as we explore the ever-evolving tech landscape, it's important to share our knowledge and help each other grow. So hit that share button and spread the love for tech! Together, we can conquer any coding challenge. 💪
That's it for today's blog post, folks! Thanks for joining me on this Spring adventure. Until next time, happy coding! ✌️