Spring @PropertySource using YAML
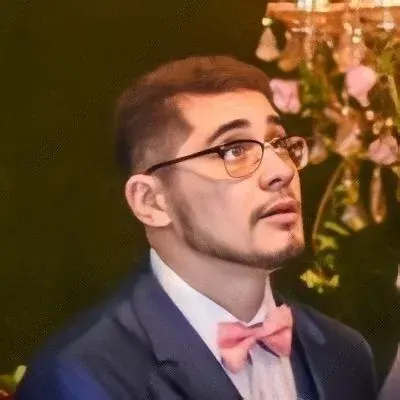
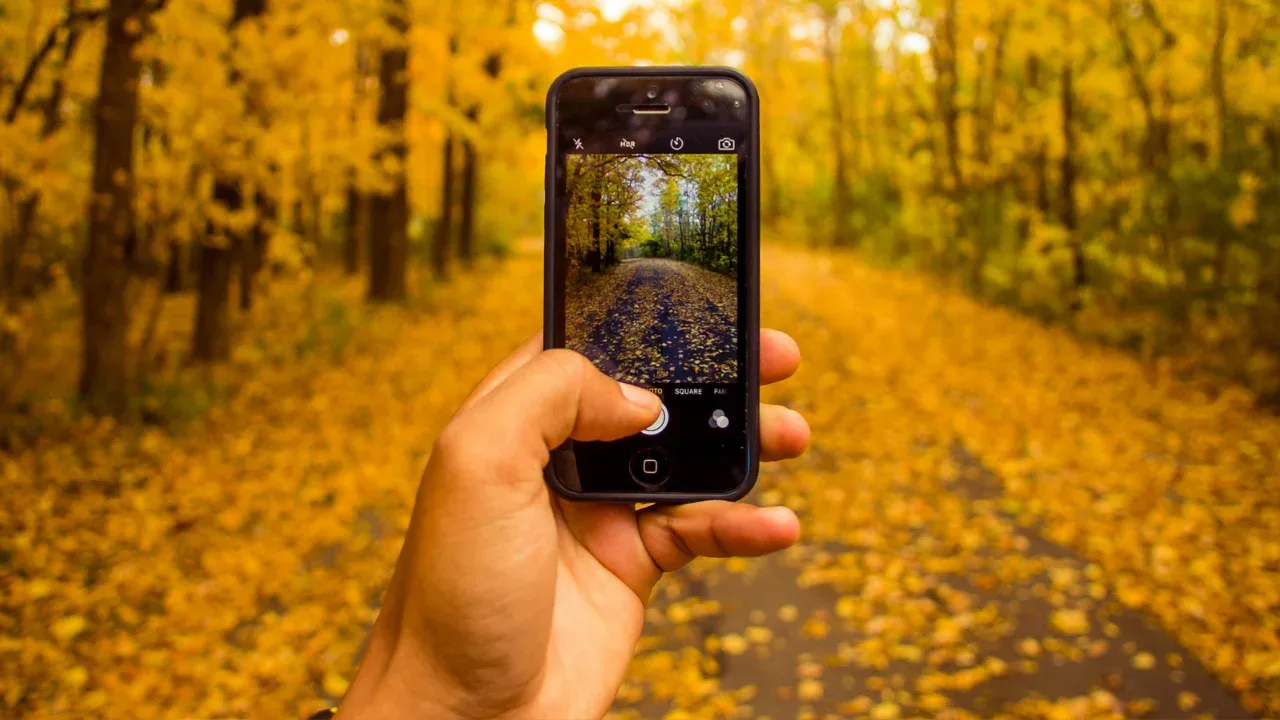
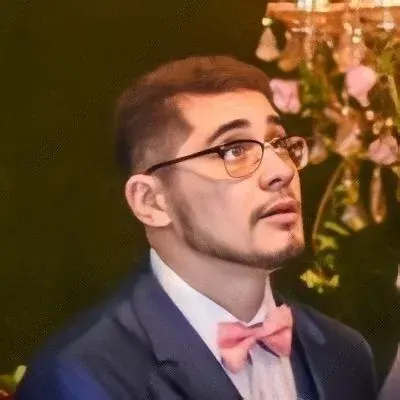
🌸 Spring @PropertySource using YAML: A YAMLicious Solution 🌸
Are you getting tangled up when trying to use YAML for your Spring Boot configuration files? 😖 Don't worry, you're not alone! Many developers encounter issues, especially when it comes to using YAML in their tests. 🙇♂️ But fear not! In this guide, we'll unravel the mystery and show you how to leverage the YAML goodness in your tests with ease. 🎉
The Dilemma
Let's start by examining the problem. When you use the @PropertySource
annotation (which points to your YAML file), you might encounter an error where Spring is unable to resolve the placeholder values. 😩
Unveiling the Solution
To overcome this hurdle, we need to make a few modifications to our code. Let's dive right in! 💪
Step 1: Update the Configuration
First, check your existing code. If you have a TestConfiguration
class annotated with @PropertySource
like this:
@PropertySource(value = "classpath:application-test.yml")
You need to make a small change. 😉 Instead of using @PropertySource
, use @ConfigurationProperties
to bind your YAML properties directly to your Java class.
Step 2: Create a Java Class
Create a new Java class specifically for storing the YAML properties. Let's call it YamlProperties
.
@Configuration
@ConfigurationProperties(prefix = "db")
public class YamlProperties {
private String url;
private String username;
private String password;
// Getters and setters for the properties
}
By using @ConfigurationProperties
, we're telling Spring to map the properties in our YAML file with the corresponding fields in our Java class. The prefix
attribute specifies the common prefix for the properties in our YAML file.
Step 3: Refactor Your TestConfiguration
Now, update your TestConfiguration
class to use the newly created YamlProperties
:
@Configuration
public class TestConfiguration {
private final YamlProperties yamlProperties;
public TestConfiguration(YamlProperties yamlProperties) {
this.yamlProperties = yamlProperties;
}
// Your configuration code
}
Spring will automatically inject the YamlProperties
bean into our TestConfiguration
class.
Step 4: Leverage the YAML Goodness
Finally, make use of the values from your YAML file, just like you did before:
@Value("${db.username}")
private String username;
🚀 Time to Shine in Your Tests! 🚀
With these simple steps, you can now fully embrace the YAML goodness in your tests! No more placeholder resolution errors! 🎊
What are you waiting for? Give it a try and let us know how it works for you! If you have any questions or alternative solutions, share them below in the comments. We'd love to hear from you! 😊
Stay tuned for more exciting tech tips and tricks. Don't forget to follow us on Twitter!
🏁 Conclusion
In this guide, we demystified the challenge of using YAML for Spring Boot configuration files in your tests. By making a few adjustments to your code, you can seamlessly leverage the YAML goodness without facing any placeholder resolution issues.
Now it's time to put your newfound knowledge into action! Update your tests, embrace the YAML way, and conquer any configuration hurdle that comes your way!
Remember, YAML is no longer an obstacle but a powerful ally in your Spring Boot adventures. Happy coding! 💻💪