Spring Data JPA map the native query result to Non-Entity POJO
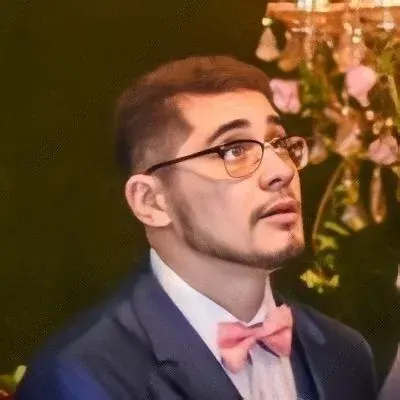
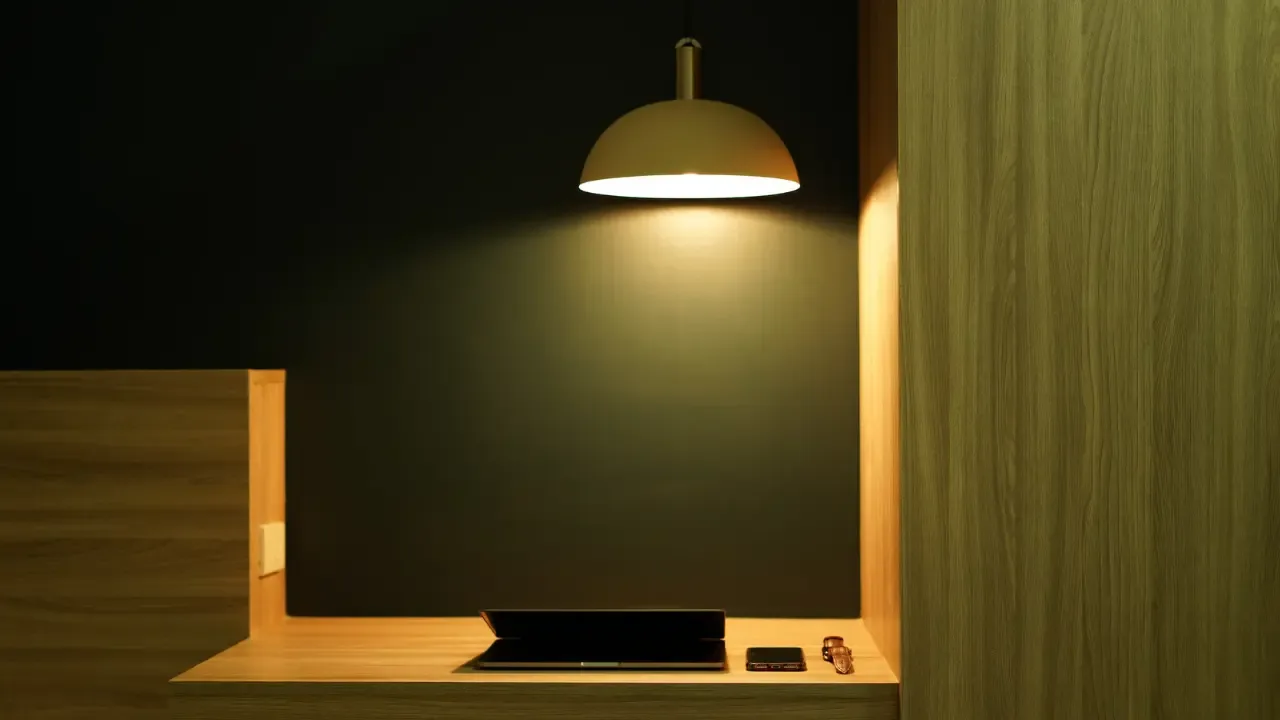
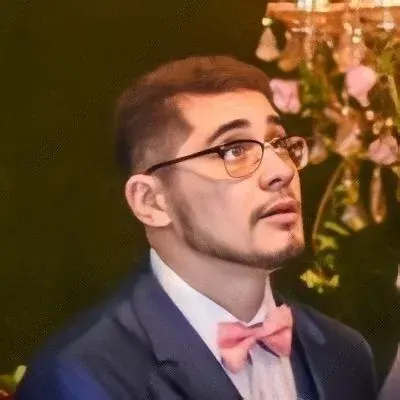
📝 Spring Data JPA: Mapping Native Query Results to Non-Entity POJOs 🌱
Have you ever faced the challenge of mapping the result of a native query to a Non-Entity POJO in Spring Data JPA? Fear not! In this blog post, we will explore common issues and provide easy solutions to help you achieve this goal seamlessly. So, let's dive in and get your data mapped effortlessly! 💪🔍
🔮 The Problem: Mapping Native Query Results to Non-Entity POJOs
Let's say you have a Spring Data repository method that utilizes a native query to fetch data from your database. You might want to map the result of this query to a Non-Entity POJO, in this case, the GroupDetails
class – but how can you accomplish this? 🤔
🔧 The Solution: Utilizing the @SqlResultSetMapping
and EntityManager
Spring Data JPA offers several mechanisms to map native query results to Non-Entity POJOs. In this example, we will leverage the @SqlResultSetMapping
annotation and the EntityManager
class for a straightforward solution. Here's how you can do it:
1️⃣ Define the Result Set Mapping
Create a GroupDetailsMapping
class with the @SqlResultSetMapping
annotation. This annotation allows you to specify the mapping between the result set columns and the fields in your Non-Entity POJO.
@SqlResultSetMapping(
name = "GroupDetailsMapping",
classes = @ConstructorResult(
targetClass = GroupDetails.class,
columns = {
@ColumnResult(name = "group_id", type = Integer.class),
@ColumnResult(name = "group_name", type = String.class),
// Add more columns and their respective types here
}
)
)
2️⃣ Implement the Repository Method
In your repository interface, add the native query method and annotate it with @Query
as you did before. However, this time, include the resultSetMapping
parameter with the name of the result set mapping you defined previously.
@Query(
value = "SELECT g.group_id, g.group_name FROM group g LEFT JOIN group_members gm ON g.group_id = gm.group_id and gm.user_id = :userId WHERE g.group_id = :groupId",
nativeQuery = true,
resultSetMapping = "GroupDetailsMapping"
)
GroupDetails getGroupDetails(@Param("userId") Integer userId, @Param("groupId") Integer groupId);
3️⃣ Use the EntityManager to Retrieve the Result
Create a method in your repository implementation class to retrieve the query result using the EntityManager
class. This method should be annotated with @PersistenceContext
to inject the EntityManager
.
@Repository
public class GroupRepositoryImpl implements GroupRepository {
@PersistenceContext
private EntityManager entityManager;
public GroupDetails getGroupDetails(Integer userId, Integer groupId) {
Query query = entityManager.createNativeQuery(
"SELECT g.group_id, g.group_name FROM group g LEFT JOIN group_members gm ON g.group_id = gm.group_id and gm.user_id = :userId WHERE g.group_id = :groupId",
"GroupDetailsMapping"
);
query.setParameter("userId", userId);
query.setParameter("groupId", groupId);
return (GroupDetails) query.getSingleResult();
}
}
📢 Call-to-Action: Dive into Spring Data JPA Native Query Mapping! Now that you understand how to map native query results to Non-Entity POJOs in Spring Data JPA, it's time to put this knowledge into action! Don't shy away from using native queries or mapping complex result sets – you've got this! 💪💡
Feel free to experiment with different mappings and customize them to suit your specific needs. And if you encounter any further challenges, remember that the Spring Data JPA documentation and vibrant community are always there to lend a helping hand. Happy coding! 🎉🚀
Now, let's hear from you. Have you faced any difficulties when mapping native query results to Non-Entity POJOs in Spring Data JPA? Share your experiences, thoughts, and solutions in the comments below! Let's learn and grow together. 🌱📝
✍️ Be sure to follow our blog for more engaging tech content and subscribe to our newsletter for regular updates and exclusive tips. Until next time, happy coding! 😄👩💻👨💻