Spring Boot Remove Whitelabel Error Page
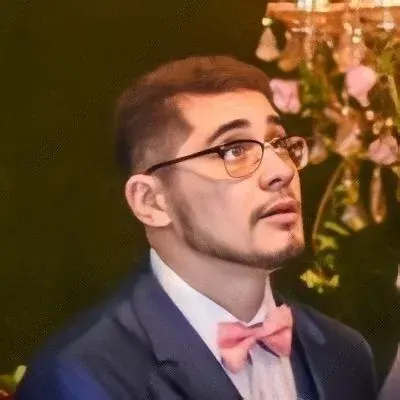
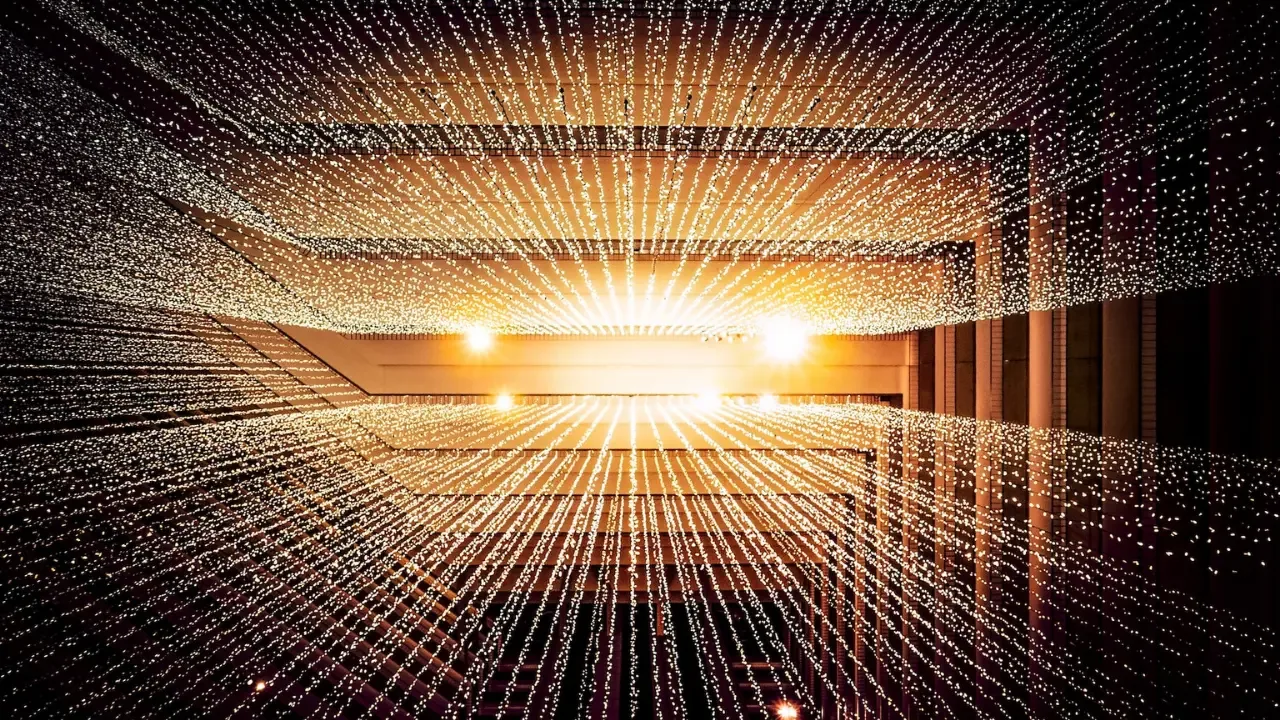
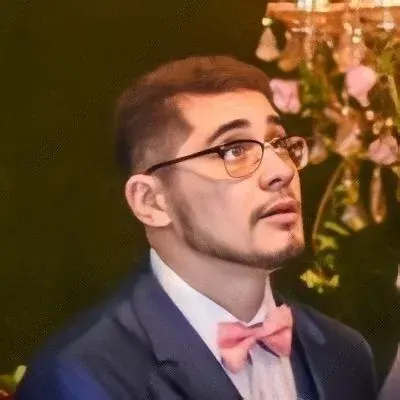
🚀 Removing the Whitelabel Error Page in Spring Boot
Are you frustrated with the default Whitelabel Error Page in your Spring Boot application? Don't worry, we've got you covered! In this blog post, we'll discuss a common issue when trying to remove the Whitelabel Error Page and provide you with easy-to-follow solutions. Let's dive in!
The Problem 😫
You followed the traditional approach by creating a controller mapping for "/error" in your Spring Boot application:
@RestController
public class IndexController {
@RequestMapping(value = "/error")
public String error() {
return "Error handling";
}
}
But unfortunately, instead of getting rid of the Whitelabel Error Page, you encountered an exception:
Exception in thread "AWT-EventQueue-0" org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'requestMappingHandlerMapping' defined in class path resource [org/springframework/web/servlet/config/annotation/DelegatingWebMvcConfiguration.class]:
Invocation of init method failed; nested exception is java.lang.IllegalStateException: Ambiguous mapping found. Cannot map 'basicErrorController' bean method
public org.springframework.http.ResponseEntity<java.util.Map<java.lang.String, java.lang.Object>> org.springframework.boot.autoconfigure.web.BasicErrorController.error(javax.servlet.http.HttpServletR equest)
to {[/error],methods=[],params=[],headers=[],consumes=[],produces=[],custom=[]}: There is already 'indexController' bean method
You might be scratching your head, wondering if you did something wrong. Fear not, because we'll help you troubleshoot and resolve this issue step by step!
The Solution 💡
1. Check for conflicting mappings
The error message mentions an "Ambiguous mapping" issue. It seems that there is a conflict between the BasicErrorController
bean method and your IndexController
method. To overcome this, you need to remove the conflict by slightly modifying your IndexController
. Here's an example:
@RestController
public class IndexController {
@RequestMapping(value = "/error", method = RequestMethod.GET)
public String error() {
return "Error handling";
}
}
By explicitly specifying the request method as GET
, you ensure that there is no conflicting mapping and the Whitelabel Error Page is properly removed.
2. Verify application.properties setup
You mentioned that you already added error.whitelabel.enabled=false
to your application.properties
file, but you're still encountering the same error. Let's double-check your setup:
Ensure that the
application.properties
file is in the correct location (src/main/resources
).Verify that there are no typos or extra spaces in the property
error.whitelabel.enabled=false
.
If everything looks good, let's move on to the next step.
3. Try alternative application.yml setup
Instead of using the application.properties
file, you can also try configuring the property in the application.yml
file. Here's an example format:
error:
whitelabel:
enabled: false
Make sure the application.yml
file is placed in the same location as the application.properties
file. Sometimes, switching to the YAML configuration file can resolve certain issues related to property settings.
That's it! 😎
You've successfully troubleshooted and resolved the issue of removing the Whitelabel Error Page in your Spring Boot application. Now, you can handle errors in a more customized and user-friendly manner with your own error handling logic.
If you found this guide helpful, don't hesitate to share it with other developers facing the same problem. Feel free to leave a comment below if you have any questions or need further assistance. Happy coding!
💌 Your Turn!
Have you encountered any other challenging Spring Boot issues? Let us know in the comments, and we'll be happy to assist you with more guides and solutions. Keep exploring, keep learning, and keep coding!