Spring Boot - Loading Initial Data
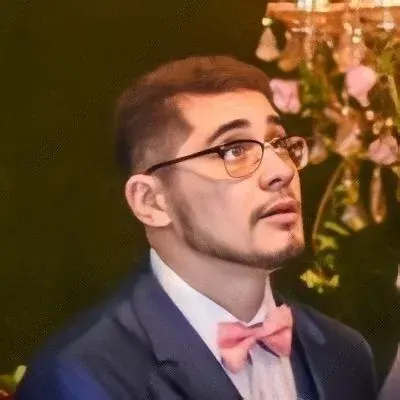
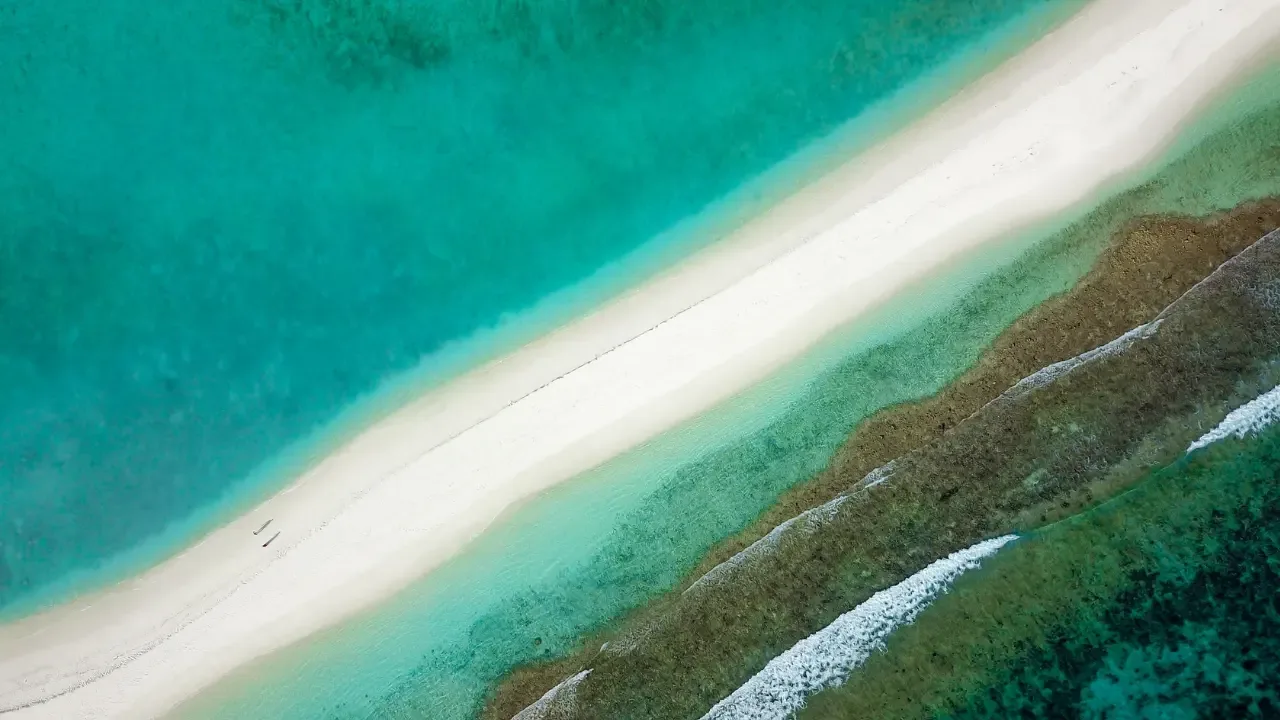
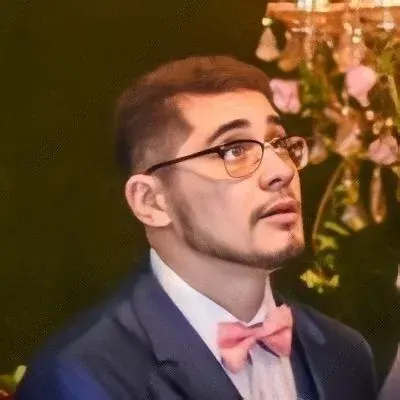
🌱 Spring Boot - Loading Initial Data 🌱
Are you wondering how to load initial data into your Spring Boot application's database? 🤔 Don't worry, I've got you covered! In this blog post, I'll explain the best way to load initial data and provide you with some easy solutions. Let's get started!
The Challenge: Filling your H2 Database with Data
Let's say you have a Spring Boot application with an H2 database, and you want to initially populate it with some data. For example, you have a domain model called "User" and want to access users by going to /users
. However, initially, your database won't have any users, so you need to create them.
The Traditional Approach: Using a DataLoader Bean
The code you provided shows a common approach called a DataLoader
bean. This bean gets instantiated by the container and is responsible for creating users in your database. While this approach works, you might be wondering if there's a better way. 🤔
@Component
public class DataLoader {
private UserRepository userRepository;
@Autowired
public DataLoader(UserRepository userRepository) {
this.userRepository = userRepository;
loadUsers();
}
private void loadUsers() {
userRepository.save(new User("lala", "lala", "lala"));
}
}
A Better Solution: Using Data.sql
Fortunately, Spring Boot provides an even easier and more elegant solution for loading initial data: the data.sql
file. By placing a file named data.sql
in the classpath, Spring Boot will automatically execute the SQL statements inside it during application startup.
To use this feature, follow these steps:
Create a file named
data.sql
in thesrc/main/resources
directory of your project.Inside
data.sql
, write your SQL statements to populate the database. For example, to create a user, you could use the following statement:
INSERT INTO users (username, password, name) VALUES ('lala', 'lala', 'lala');
That's it! Spring Boot will take care of executing the SQL statements in data.sql
for you on application startup.
Take it to the Next Level: Using data-h2.sql
If you're using an H2 database, you can further enhance your initial data loading process by using a file named data-h2.sql
instead of data.sql
. By doing this, you can write H2-specific SQL statements that take advantage of H2 features.
For example, you could leverage H2's advanced capabilities by using an IDENTITY
column for automatically generated IDs:
CREATE TABLE users (
id INT PRIMARY KEY AUTO_INCREMENT,
username VARCHAR(255) NOT NULL,
password VARCHAR(255),
name VARCHAR(255)
);
Conclusion and Call to Action
Congratulations! Now you know the best way to load initial data into your Spring Boot application's database. By using the data.sql
or data-h2.sql
file, you can easily populate your database with the necessary data and ensure a smooth application start.
So, what are you waiting for? Go ahead and try it out in your project. Don't forget to share this blog post with your fellow developers who might find it useful. Let's make database initialization a breeze! 💪
Feel free to leave a comment below and let me know if you have any further questions or if there's any other topic you'd like me to cover in my next blog post. Happy coding! 😊