Spring @Autowire on Properties vs Constructor
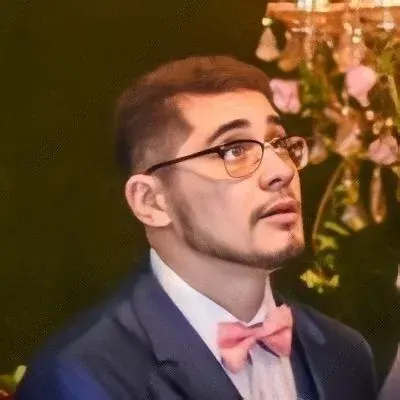
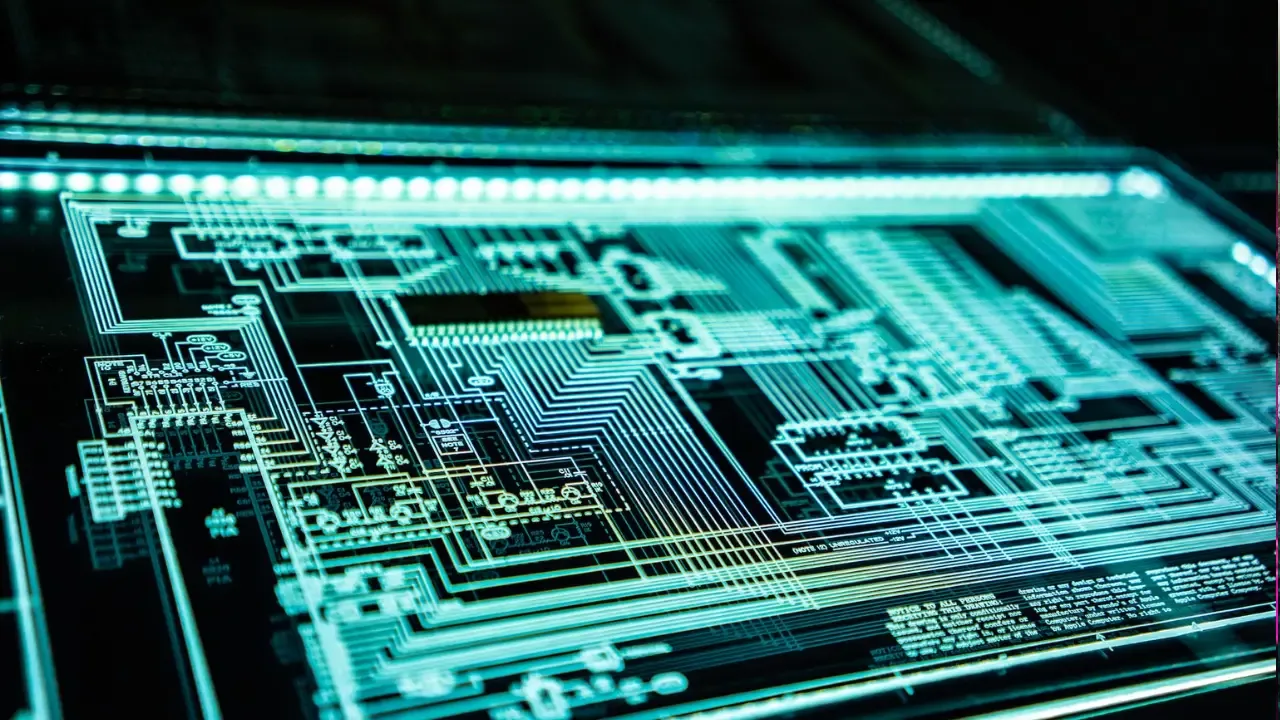
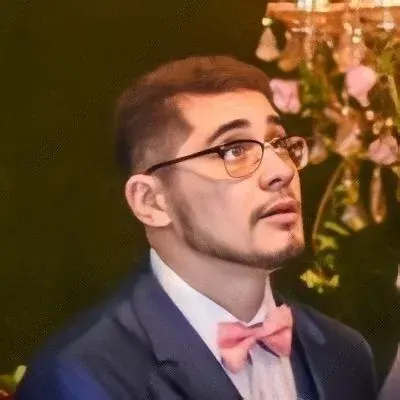
Spring @Autowire on Properties vs Constructor: Which Approach Should You Choose? 🧩
So you've been using Spring and you've stumbled upon two different ways to achieve dependency injection: using the @Autowired
annotation on properties or using it on the constructor. Which approach should you use? Is there any advantage to using one method over the other? Let's dive into this conundrum and find easy solutions to common issues that might arise with these approaches. 🤔
Understanding the Two Approaches 👩🏫👨🏫
Approach A: @Autowired
on Properties
In this approach, the @Autowired
annotation is applied directly to the property you want to inject. Let's take a look at an example:
@Component
public class SomeService {
@Autowired
private SomeOtherService someOtherService;
}
With this approach, Spring will automatically inject the SomeOtherService
bean into the someOtherService
property of the SomeService
class.
Approach B: @Autowired
on Constructor
In the second approach, the @Autowired
annotation is used on the constructor instead of the property. Here's how it looks:
@Component
public class SomeService {
private final SomeOtherService someOtherService;
@Autowired
public SomeService(SomeOtherService someOtherService){
this.someOtherService = someOtherService;
}
}
This approach explicitly declares a constructor that takes the SomeOtherService
bean as a parameter. Spring then uses this constructor to inject the dependency when creating an instance of SomeService
.
The Advantage of Approach B 🛠
You might be wondering, "Is there any advantage to using approach B when approach A seems simpler?" Absolutely! Let's explore the advantages of using @Autowired
on the constructor:
Explicit dependencies: Using the constructor makes the dependencies of a class explicit. By declaring the dependencies in the constructor, you can immediately see what other beans this class relies on. This provides better clarity and maintainability.
Enhanced testability: When you use approach B, you can easily inject mock dependencies during testing. By writing constructor-based tests, you have full control over the dependencies being used. This can result in more robust and isolated unit tests.
Reduced coupling: Approach B reduces the coupling between classes, making your code more flexible and modular. The use of constructor injection allows for easier swapping of dependencies without modifying the class itself.
No need for
@InjectMocks
: With approach A, you might be familiar with using the@InjectMocks
annotation to inject dependencies into a class instance during testing. However, with approach B, there's no need for@InjectMocks
as you can simply pass the mock dependency in the constructor while creating the instance for testing.
While approach A might seem simpler and more concise, approach B provides clear advantages in terms of code clarity, testability, and flexibility. It promotes best practices in dependency injection, making your codebase more maintainable in the long run. 🏗
Conclusion - The Preferred Way to Do Dependency Injection 🎉
To sum it up, using @Autowired
on the constructor (approach B) is considered a best practice when it comes to dependency injection in Spring. It provides explicit dependencies, enhances testability, reduces coupling, and eliminates the need for @InjectMocks
.
While both approaches technically work, the benefits of approach B make it the more preferred way to achieve dependency injection.
So, next time you're faced with a dependency injection dilemma, remember the advantages of approach B and consider adopting it in your Spring projects. 🌱
Share Your Thoughts! 💬
Have you encountered any challenges or benefits while using Spring's @Autowired
on properties or constructors? Let us know in the comments below! We'd love to hear your experiences with different dependency injection approaches.
Happy coding! 💻