Spring 3 RequestMapping: Get path value
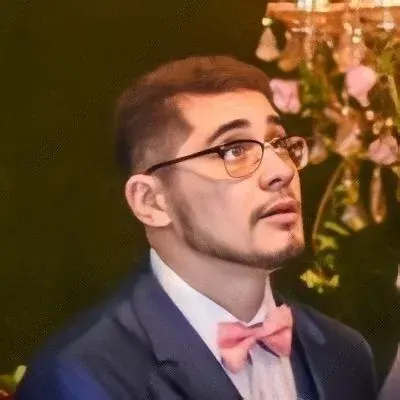
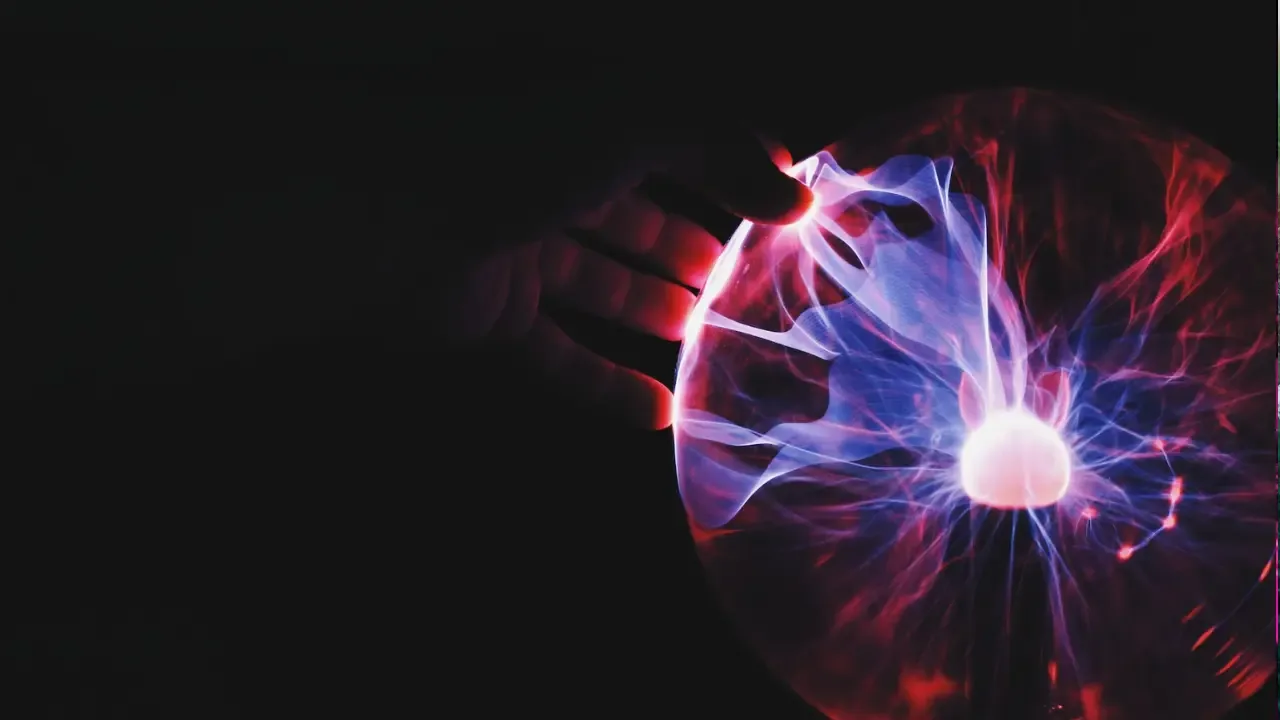
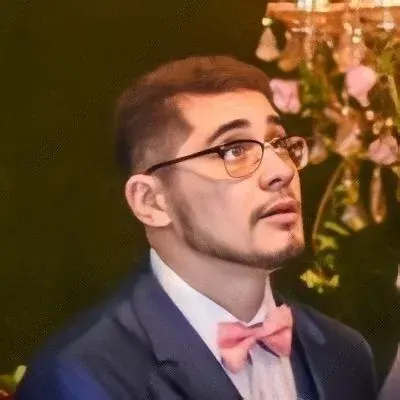
š Blog Post Title: Mastering Spring 3 RequestMapping: Unleash the Power of Path Values
š” Introduction: Spring 3 RequestMapping is a core feature that allows developers to map URLs to specific controller methods. While it offers great convenience, there may be times when you need to extract specific values from the URL path after the @PathVariable values have been parsed. Fear not, for in this blog post, we will uncover the secrets of accessing the complete path value using Spring 3 RequestMapping.
š Understanding the Challenge:
The question at hand is how to extract the complete path value, including the remaining URL segments, after the @PathVariable values have been parsed. Let's break it down with an example:
Consider the following URL pattern: /{id}/{restOfTheUrl}
We want to obtain the values id=1
and restOfTheUrl=/dir1/dir2/file.html
from the URL /1/dir1/dir2/file.html
. Sounds tricky, huh?
šØāš¬ Potential Solutions:
Solution 1 - Use HttpServletRequest: One way to achieve this is by using the HttpServletRequest object provided by Spring. You can access the complete request URL using
request.getRequestURI()
. To extract the remaining URL segments, you can remove the @PathVariable segments usingrequest.getAttribute(HandlerMapping.URI_TEMPLATE_VARIABLES_ATTRIBUTE)
.Solution 2 - Custom Method Argument Resolver: Another approach is to create a custom method argument resolver. By implementing the HandlerMethodArgumentResolver interface, you can intercept the request and extract the necessary path values. This gives you greater control and flexibility over the process.
Solution 3 - Regular Expressions: If you prefer a more concise solution, you can leverage regular expressions (regex) to match the desired pattern and extract the required path values. This option requires a solid understanding of regex, but it can be a powerful tool once mastered.
š Implementation Examples:
Solution 1 - Using HttpServletRequest:
@RequestMapping("/{id}/{restOfTheUrl}")
public ResponseEntity<String> handleRequest(HttpServletRequest request, @PathVariable("id") int id) {
String completePath = request.getRequestURI();
Map<String, String> pathVariables = (Map<String, String>) request.getAttribute(HandlerMapping.URI_TEMPLATE_VARIABLES_ATTRIBUTE);
String restOfTheUrl = pathVariables.get("restOfTheUrl");
// Use the extracted values as needed
// ...
return ResponseEntity.ok("Success");
}
Solution 2 - Custom Method Argument Resolver:
@Component
public class RestOfTheUrlResolver implements HandlerMethodArgumentResolver {
@Override
public boolean supportsParameter(MethodParameter parameter) {
return parameter.getParameterType().equals(String.class) &&
parameter.hasParameterAnnotation(RestOfTheUrl.class);
}
@Override
public Object resolveArgument(MethodParameter parameter, ModelAndViewContainer mavContainer, NativeWebRequest webRequest, WebDataBinderFactory binderFactory) throws Exception {
HttpServletRequest request = (HttpServletRequest) webRequest.getNativeRequest();
String requestURI = request.getRequestURI();
String[] pathSegments = requestURI.split("/");
int startIndex = Arrays.asList(pathSegments).indexOf(parameter.getParameterName());
return String.join("/", Arrays.copyOfRange(pathSegments, startIndex, pathSegments.length));
}
}
@RequestMapping("/{id}/{restOfTheUrl}")
public ResponseEntity<String> handleRequest(@PathVariable int id, @RestOfTheUrl String restOfTheUrl) {
// Use the extracted values as needed
// ...
return ResponseEntity.ok("Success");
}
š£ Call-to-Action: Now that you have discovered the magic behind extracting the complete path value in Spring 3 RequestMapping, it's time to put this knowledge into practice! Experiment with the provided examples and see which solution works best for your use case. If you have any questions or alternative approaches, feel free to share them in the comments below. Happy coding! š»āØ
Remember to share this blog post with other developers who might find it useful. Sharing is caring! šš
š Author's note: Throughout the blog post, I used Markdown language to format the content properly. Markdown is a lightweight markup language that allows for easy text organization, formatting, and hyperlinks. It's widely used in blogs and documentation.