@Scope("prototype") bean scope not creating new bean
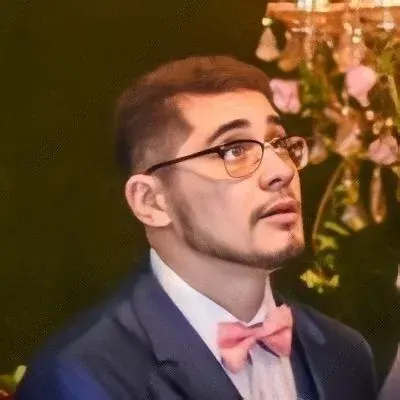
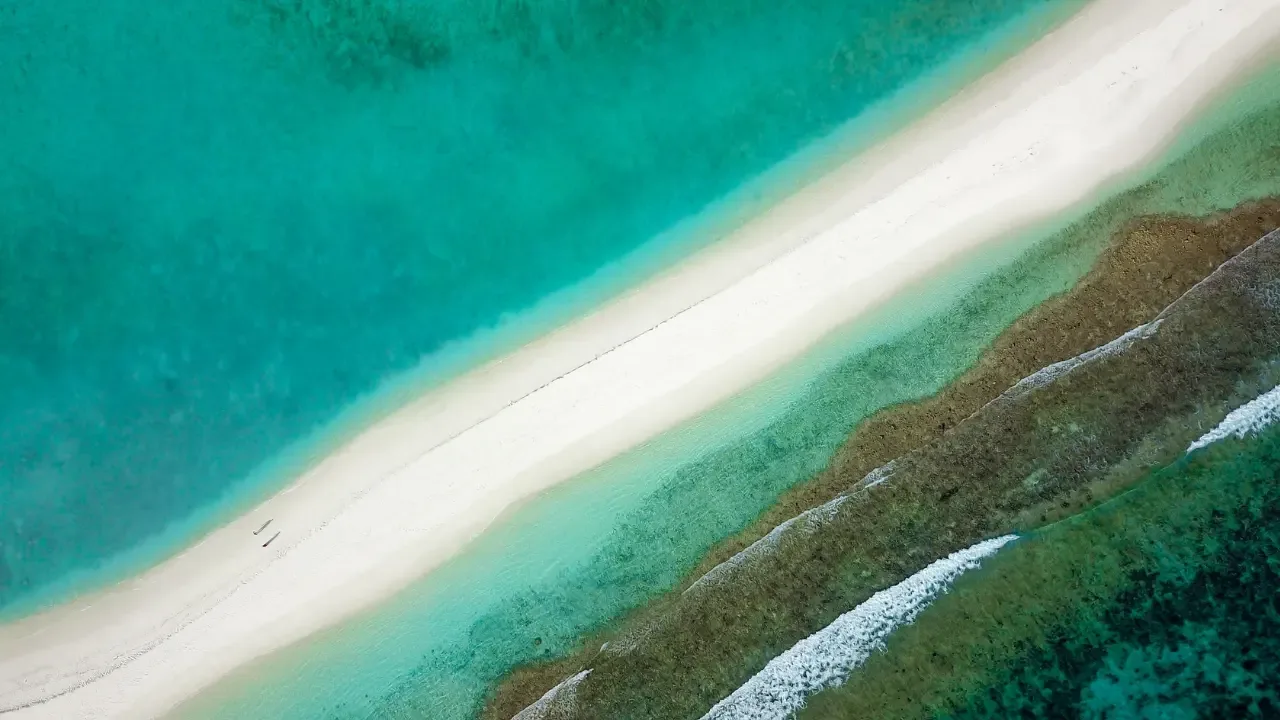
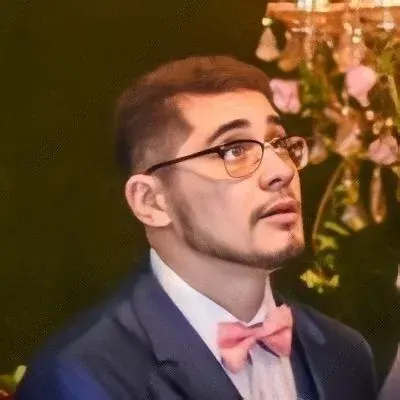
š Blog Post: Why is my @Scope("prototype") Bean not Creating a New Bean?
⨠Introduction āØ
Hey there fellow tech enthusiasts! Have you ever encountered a situation where your annotated prototype bean in your Spring application didn't create a new instance and opted for a singleton instead? Frustrating, isn't it? But worry no more! In this blog post, we will dive into this common issue and provide you with easy solutions to fix it. So, let's get started!
š Problem Analysis šµļøāāļø
Okay, let's assess the provided code snippet to understand what's going on. We can see that the LoginAction
class is annotated with @Component
and @Scope("prototype")
, indicating that Spring should create a new instance of this bean whenever it's requested.
However, in the HomeController
class, when injecting the LoginAction
bean using @Autowired
, it appears that Spring is not creating new instances of the LoginAction
bean as expected. Instead, it seems to be using a singleton instance.
So, why is this happening? Let's explore some possible causes and their solutions.
š” Possible Causes and Solutions š”
1ļøā£ Cause: Controller not being web application context-aware.
Solution: As suggested by the update in the question, making the HomeController
web application context aware can resolve this issue. By injecting the WebApplicationContext
, we can dynamically fetch a new instance of the LoginAction
bean from the application context.
Updated Solution Code:
@Controller
public class HomeController {
@Autowired
private WebApplicationContext context;
@RequestMapping(value = "/view", method = RequestMethod.GET)
public ModelAndView display(HttpServletRequest req){
ModelAndView mav = new ModelAndView("home");
mav.addObject("loginAction", getLoginAction());
return mav;
}
public LoginAction getLoginAction() {
return (LoginAction) context.getBean("loginAction");
}
}
2ļøā£ Cause: Incorrect configuration or missing annotations. Solution: Ensure that the necessary configuration and annotations are in place. Here are a few points to check:
Verify that
config.xml
has component scanning enabled, as shown in the provided snippet.Make sure the base package defined in the
component-scan
configuration includes the package where yourLoginAction
class resides.Double-check that the
LoginAction
class does not have any additional annotations that might interfere with the expected prototype behavior.
If you have verified everything and the issue persists, let's move on to the next possible cause.
3ļøā£ Cause: Bean proxying interferes with prototype behavior. Solution: In certain scenarios, such as when using AOP or proxy-based transactions, Spring may create a proxy for your bean. This proxy intercepts method invocations, leading to the reuse of the same instance instead of creating new ones.
To overcome this, you can try using interface-based proxies instead of class-based proxies by coding to interfaces wherever possible. Alternatively, you can set the proxyTargetClass
attribute of the <aop:config>
element to true
in your config.xml
.
Updating config.xml
with proxy configuration:
<aop:config proxy-target-class="true">
<!-- Your AOP configurations here -->
</aop:config>
ā Wrap Up and Call-to-Action ā
Congratulations on making it this far! By now, you should have a clearer understanding of why your @Scope("prototype")
bean might not be creating new instances and how to address this issue.
Remember, if you encounter this problem again or face any other technical challenges, don't hesitate to consult the vast knowledge base of the developer community. Sharing your experiences and solutions can benefit others who might encounter similar issues.
So, let's engage in a conversation! Share your own experience with prototype bean issues, provide additional insights, or ask any questions you may have in the comments section below. Together, we can level up our coding game!
Happy coding! šš©āš»šØāš»