Injecting Mockito mocks into a Spring bean
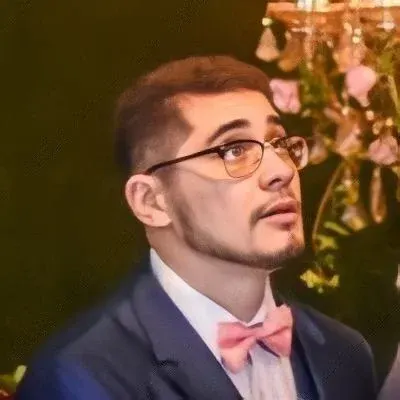
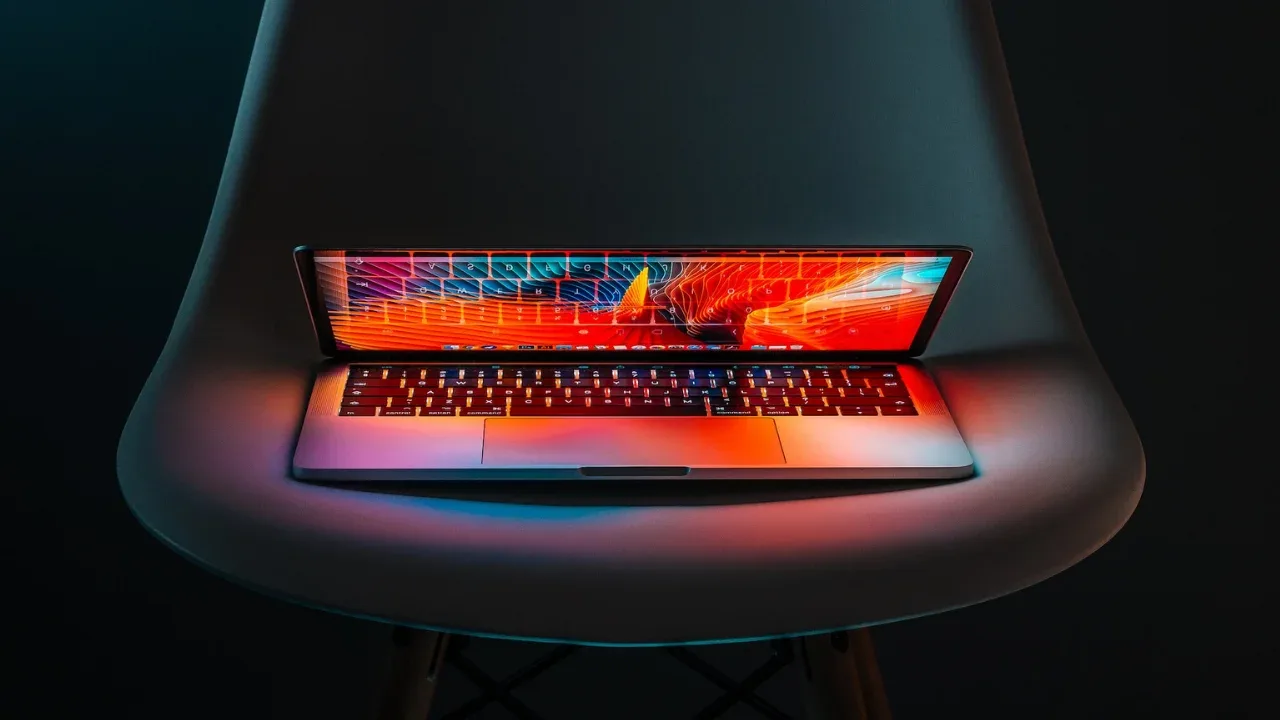
๐ Injecting Mockito mocks into a Spring bean: Easy Solutions! ๐
Do you find it challenging to inject a Mockito mock object into a Spring bean for unit testing? ๐ Don't worry, we've got you covered! In this blog post, we will address common issues and provide easy solutions to help you overcome this obstacle. Let's dive in! ๐ช
The Problem:
You want to inject a Mockito mock object into a Spring bean, but you're encountering issues with auto-wiring and proxying. ๐ซ
The Solution:
Here's a simple solution to inject your Mockito mock into the Spring bean effectively:
Create a Mockito mock bean using the
<bean>
tag in your Spring configuration XML file. Specify the class you want to mock and the ID for referencing it.<bean id="myMockBean" class="org.mockito.Mockito" factory-method="mock"> <constructor-arg value="com.example.MyClass" /> </bean>
Replace
com.example.MyClass
with the fully qualified name of the bean you want to inject the mock into.In your bean class, use the
@Autowired
annotation to inject the mock bean into a private member field. Make sure the field has the same type or interface as the bean you want to mock.@Autowired private MyClass myMockBean;
The
@Autowired
annotation will automatically wire the mock bean into the field.During your unit tests with JUnit, Spring will inject the mock bean into your Spring bean, allowing you to use and manipulate it freely.
Common Issues:
If you encounter any issues during the process, here are a few tips to help you troubleshoot:
Make sure you have the necessary dependencies in your project, such as Mockito and the Spring framework.
Check that your Spring configuration XML file is correctly referencing the mocking factory method and the target class/interface.
Verify that the package and class names you provide in the
<constructor-arg>
match the actual ones.Ensure that the bean you want to mock is correctly annotated with
@Component
or any other relevant Spring annotation.
Your Turn to Shine! โจ
Now that you have an easy solution in hand, it's time to put it into action! Try injecting your Mockito mock into a Spring bean and witness the magic of effective unit testing. ๐งช
Feel free to reach out if you encounter any issues or have any questions. Remember, we're here to support you in your tech journey! ๐
Happy coding and happy testing! ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
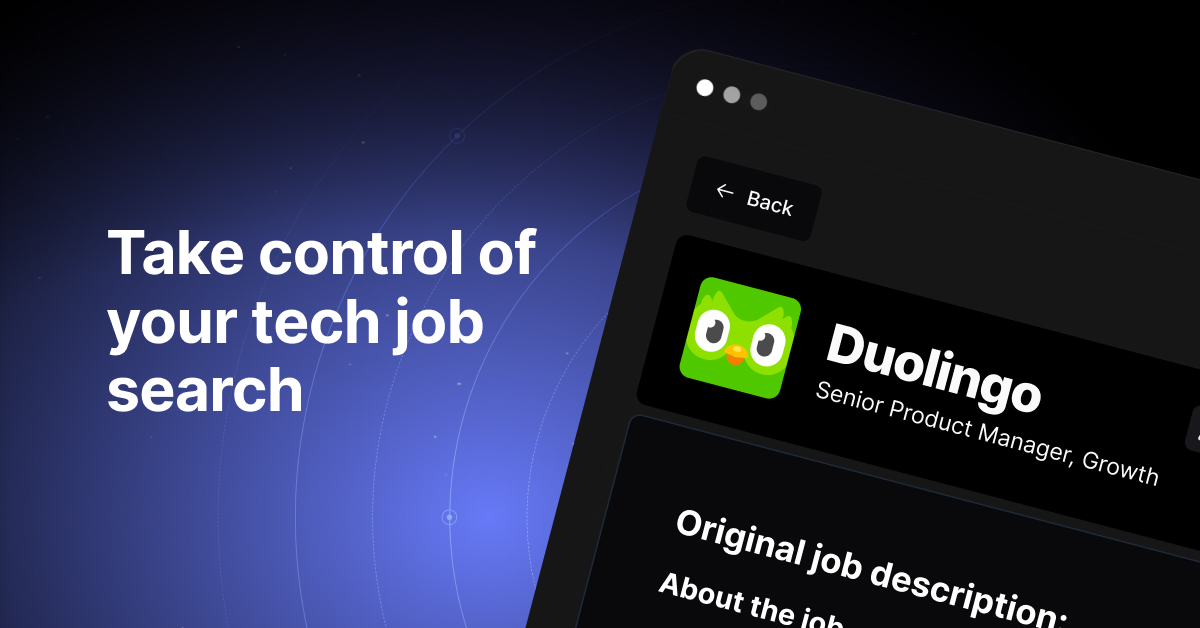