How to use OrderBy with findAll in Spring Data
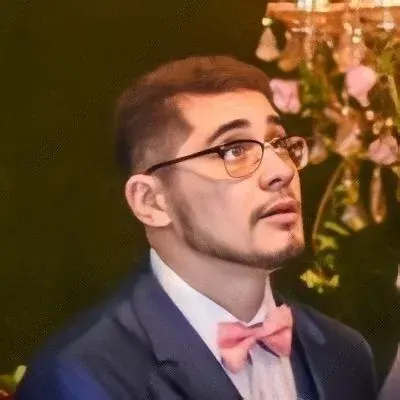
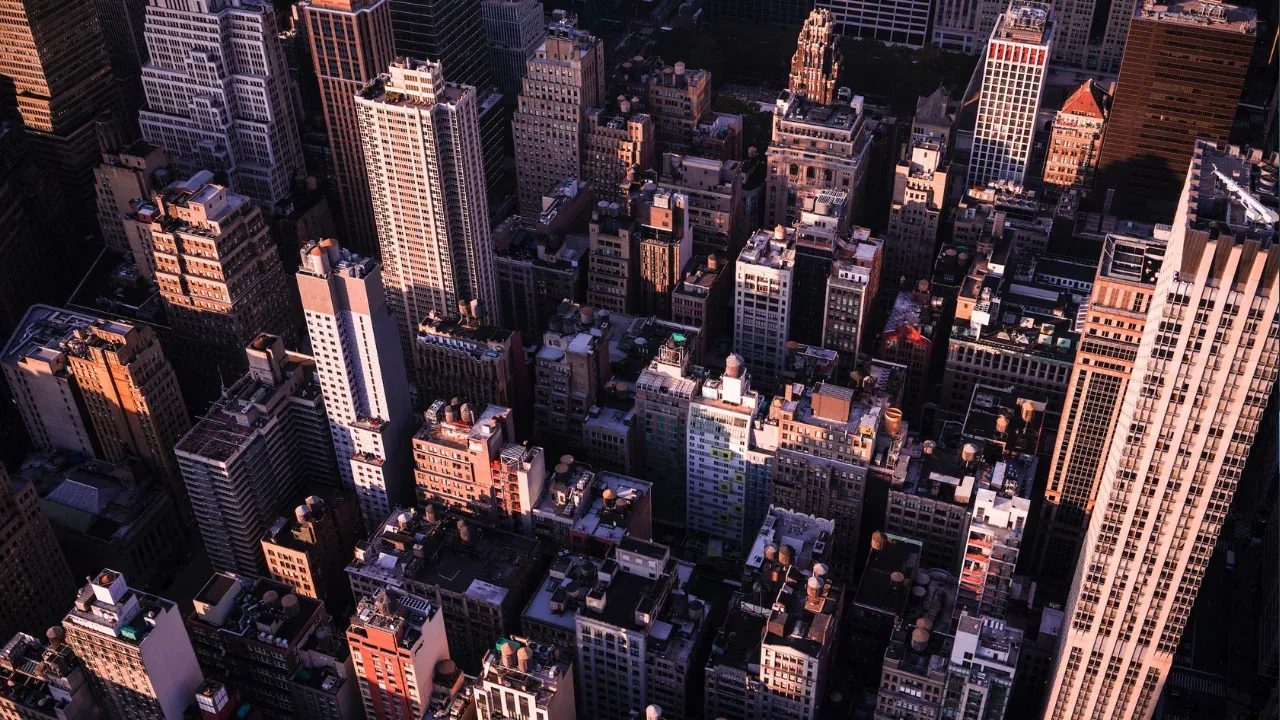
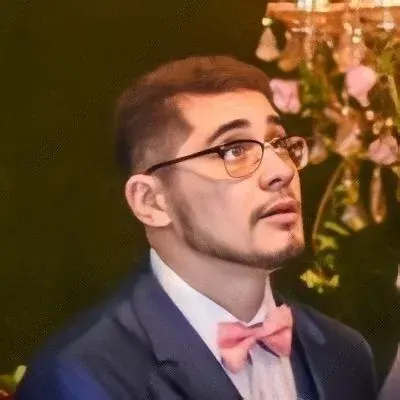
How to use OrderBy with findAll in Spring Data
So you want to use the OrderBy
clause with the findAll
method in Spring Data to retrieve records in a specific order. You're in luck because Spring Data provides built-in functionality to achieve this. Let's dive in and explore how you can achieve your goal!
The Problem: Ordering Records in Spring Data
In the example code you provided, you have a StudentDAO
interface that extends JpaRepository
. You're looking for a way to order the records by the id
column in ascending (ASC
) or descending (DESC
) order using a method like findAllOrderByIdAsc
.
The Solution: Using OrderBy in Spring Data
Spring Data offers a simple and concise way to achieve ordered results using the OrderBy
clause in method declarations. Here's how you can modify your code to accomplish this:
public interface StudentDAO extends JpaRepository<StudentEntity, Integer> {
List<StudentEntity> findAllByOrderByIdAsc(); // Ordering by id in ascending order
List<StudentEntity> findAllByOrderByIdDesc(); // Ordering by id in descending order
}
By including OrderByIdAsc
or OrderByIdDesc
in the method name, Spring Data automatically generates the appropriate SQL query with the ORDER BY
clause. This simplifies the process and provides a clean approach to sorting records.
Examples: Ordering Student Records
Let's demonstrate some usage examples for ordering student records using the methods we defined above:
List<StudentEntity> studentsAsc = studentDAO.findAllByOrderByIdAsc();
List<StudentEntity> studentsDesc = studentDAO.findAllByOrderByIdDesc();
In the studentsAsc
list, you will find the student records ordered by id in ascending order, while the studentsDesc
list will contain records ordered by id in descending order.
Conclusion and Call-to-Action
Ordering records in Spring Data is made easy with the OrderBy
clause in method declarations. Remember to append OrderByIdAsc
or OrderByIdDesc
to the method name to specify the desired sorting order. With just a few lines of code, you can retrieve your records in the order you want!
Now it's your turn to give it a try. Implement the order-by functionality in your project and see how it enhances the user experience. If you have any questions or need further assistance, feel free to leave a comment below.
Happy coding! 🚀