How to use LocalDateTime RequestParam in Spring? I get "Failed to convert String to LocalDateTime"
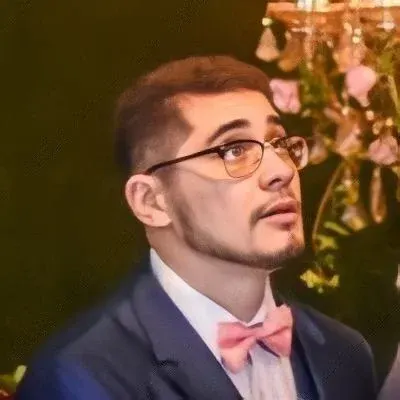
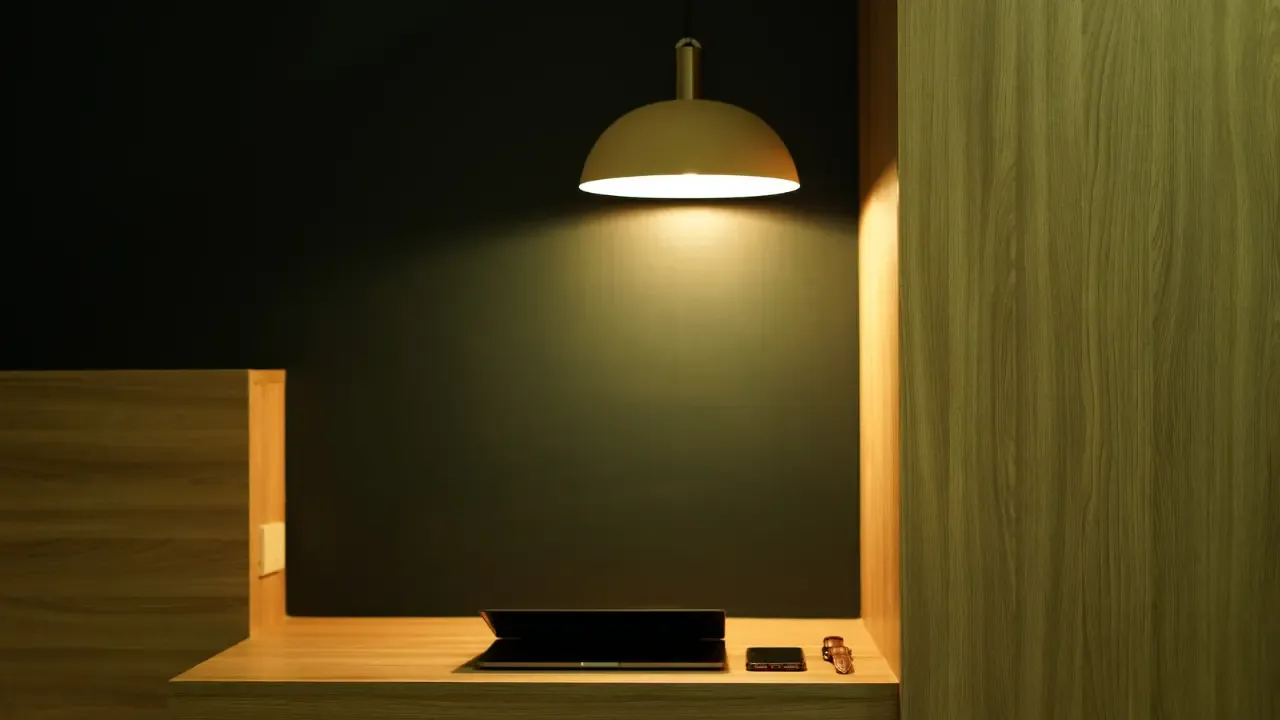
📝 Hey there! Are you struggling with using LocalDateTime RequestParam in Spring and getting the "Failed to convert String to LocalDateTime" error? 😱 Don't worry, I've got your back! In this blog post, I'll guide you through the common issues and provide you with easy solutions. So, let's get started! 🚀
First things first, make sure you have included the "jackson-datatype-jsr310" dependency in your Spring Boot project. This dependency helps with the serialization and deserialization of Java 8 Date/Time types. You can add it to your Maven project like this:
<dependency>
<groupId>com.fasterxml.jackson.datatype</groupId>
<artifactId>jackson-datatype-jsr310</artifactId>
<version>2.7.3</version>
</dependency>
Once you have the dependency configured, let's dive into the issue. In your controller method, you're using a RequestParam with LocalDateTime. Here's an example:
@GetMapping("/test")
public Page<User> get(
@RequestParam(value = "start", required = false)
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE_TIME) LocalDateTime start) {
// ...
}
The problem arises when you try to test the endpoint with a URL like this:
/test?start=2016-10-8T00:00
You receive an error message like this:
{
"timestamp": 1477528408379,
"status": 400,
"error": "Bad Request",
"exception": "org.springframework.web.method.annotation.MethodArgumentTypeMismatchException",
"message": "Failed to convert value of type [java.lang.String] to required type [java.time.LocalDateTime]; nested exception is org.springframework.core.convert.ConversionFailedException: Failed to convert from type [java.lang.String] to type [@org.springframework.web.bind.annotation.RequestParam @org.springframework.format.annotation.DateTimeFormat java.time.LocalDateTime] for value '2016-10-8T00:00'; nested exception is java.lang.IllegalArgumentException: Parse attempt failed for value [2016-10-8T00:00]",
"path": "/test"
}
This error is thrown because Spring is unable to convert the String value "2016-10-8T00:00" to a LocalDateTime object.
To fix this issue, you need to provide a date format that matches the pattern of your input string. In this case, the pattern is "yyyy-MM-dd'T'HH:mm". Here's how you can modify your controller method:
@GetMapping("/test")
public Page<User> get(
@RequestParam(value = "start", required = false)
@DateTimeFormat(pattern = "yyyy-MM-dd'T'HH:mm") LocalDateTime start) {
// ...
}
Now, when you test the endpoint with the same URL, you should no longer encounter the "Failed to convert String to LocalDateTime" error. 🎉
That's it! I hope this guide helped you resolve your issue. Feel free to share this blog post with your friends who might be facing a similar problem. If you have any further questions or need assistance, leave a comment below. Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
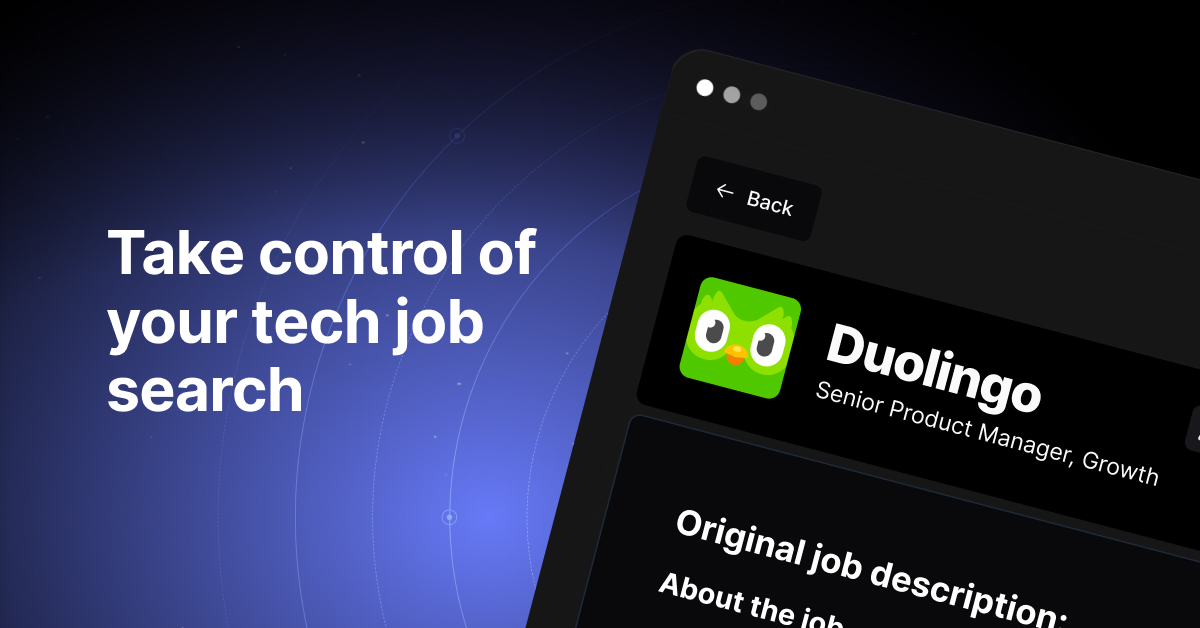