How to set an "Accept:" header on Spring RestTemplate request?
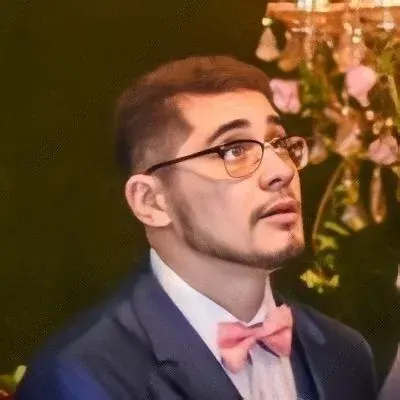
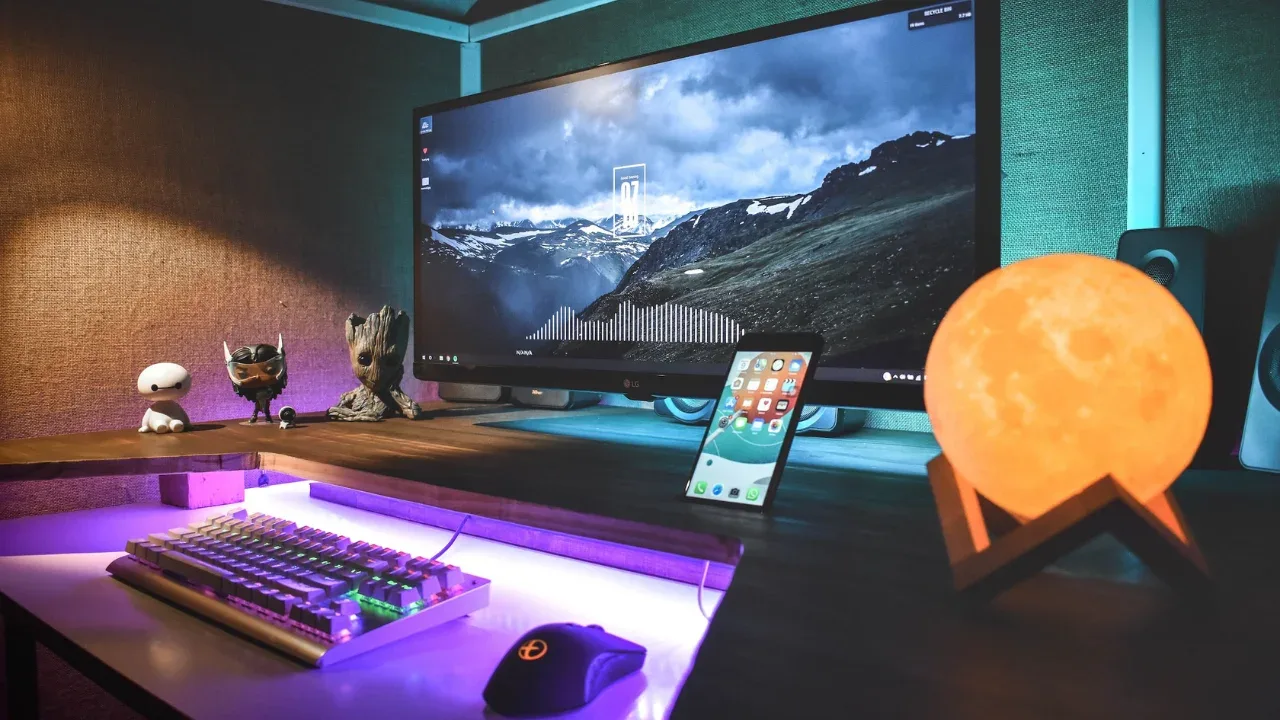
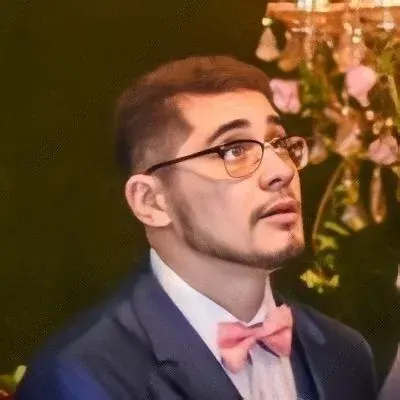
How to Set an "Accept:" Header on Spring RestTemplate Request?
Are you using Spring's RestTemplate to make requests in your Java REST client? Do you need to specify the "Accept:" header in your request to specify the response format you want to receive, such as "application/json" or "application/xml"? You're in the right place! In this blog post, we'll show you how to set the "Accept:" header on a Spring RestTemplate request.
The Problem: Missing "Accept:" Header
By default, when you send a request using RestTemplate, it sets the "Accept:" header to "*" (wildcard), which means you accept any media type. However, you may want to be more specific and indicate the media types you are willing to accept. This is especially useful if your server can respond with different types of data, and you want to ensure you receive the type you need.
In the provided context, the code snippet you shared doesn't explicitly set the "Accept:" header in the request. Therefore, RestTemplate uses the default wildcard value for the "Accept:" header.
The Solution: Setting the "Accept:" Header
To set the "Accept:" header in a RestTemplate request, you can use the HttpHeaders
class to create a HttpHeaders object and add the desired value to it. Then, when making the request, you can pass the HttpHeaders object as a parameter to the exchange
or execute
method of RestTemplate.
Here's an example of how you can set the "Accept:" header to "application/json" using RestTemplate:
public void post() {
HttpHeaders headers = new HttpHeaders();
headers.setAccept(Collections.singletonList(MediaType.APPLICATION_JSON));
HttpEntity<MultiValueMap<String, String>> requestEntity = new HttpEntity<>(params, headers);
ResponseEntity<String> response = rest.exchange(url, HttpMethod.POST, requestEntity, String.class);
String result = response.getBody();
System.out.println(result);
}
In this example, we create a new HttpHeaders object and use the setAccept
method to set the accepted media types to "application/json". Then, we create a HttpEntity
object with our request parameters and headers. Finally, we use the exchange
method of RestTemplate to send the request, passing the URL, request method (POST in this case), request entity, and the response type.
Additional Tips
For different media types, you can simply change the value passed to
setAccept
. For example, if you want to accept XML responses instead of JSON, you can useMediaType.APPLICATION_XML
instead.If you need to set other headers, such as "Content-Type", you can use the
add
method of HttpHeaders to add them to the HttpHeaders object.Don't forget to handle any exceptions that may occur when making the request, such as
RestClientException
.
Now that you know how to set the "Accept:" header on a RestTemplate request, you can make your requests more specific and ensure you receive the desired response format.
Got Feedback or Questions?
Did you find this blog post helpful? Do you have any feedback or questions? We'd love to hear from you! Feel free to leave a comment below or reach out to us on our social media channels. Happy coding! 🚀✨