How to define @Value as optional
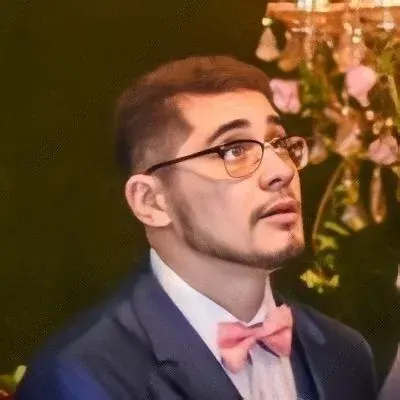
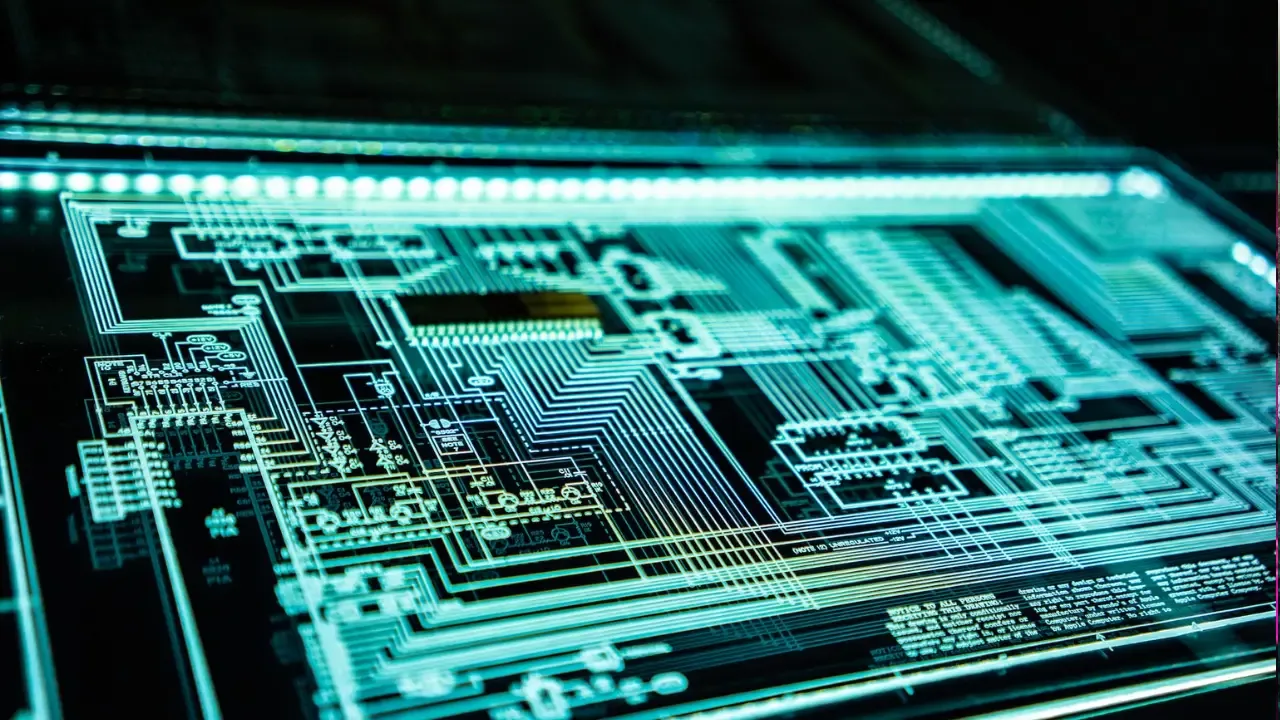
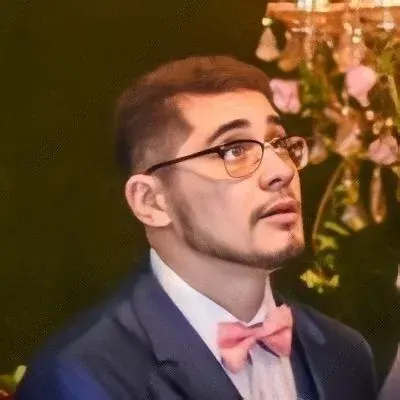
How to Define @Value as Optional: A Guide for Spring Beans
Are you struggling with making the @Value
annotation optional in your Spring bean? Fear not! We've got you covered. In this blog post, we will address the common issue of making the variable optional when it is passed as a command line parameter. We will provide you with easy solutions to ensure that your @Value
annotation is not required. So, let's dive in!
The Problem
Let's take a look at the code snippet you provided:
@Value("${myValue}")
private String value;
The above code correctly injects the value specified by the key myValue
. However, you mentioned that this variable needs to be optional, as it is passed in as a command line parameter. The issue arises when this command line argument does not always exist.
Solution 1: Using a Default Value
One solution you tried was using a default value in the @Value
annotation. You attempted both of the following approaches:
@Value("${myValue:}")
@Value("${myValue:DEFAULT}")
However, you noticed that in each case, the default value after the colon is injected even when there is an actual value. This behavior seems to override what Spring should inject.
Solution 2: Using the @Nullable Annotation
To make the @Value
annotation optional, you can make use of the @Nullable
annotation from the javax.annotation
package. Here's how you can modify your code:
import javax.annotation.Nullable;
// ...
@Value("${myValue}")
@Nullable
private String value;
By adding the @Nullable
annotation alongside the @Value
annotation, you are indicating that the value
variable can be null, meaning it is optional. With this approach, Spring will not inject a default value when the actual value is present.
Solution 3: Providing a Default Value in Code
If you prefer providing a default value in code instead of using the @Value
annotation, you can modify your code as follows:
@Value("${myValue:#{null}}")
private String value;
By specifying #{null}
as the default value, you indicate that if the myValue
key is not present in the properties file or as a command line argument, the value will be null
.
Conclusion
You've learned three effective solutions to make the @Value
annotation optional in your Spring bean. You can use the @Nullable
annotation to indicate that the variable can be null, or you can provide a default value using either the @Value
annotation (${myValue:}
or ${myValue:DEFAULT}
) or in code (${myValue:#{null}}
).
Now that you have these solutions at your disposal, you can easily handle optional values in your Spring beans. Go ahead and try them out!
If you have any further questions or face any difficulties, feel free to leave a comment below. Happy coding! 💻💪
*[JSON]: JavaScript Object Notation